
Simple Pong game on NokiaLCD with PS2
Dependencies: mbed PS2 NokiaLCD
main.cpp
00001 #include "mbed.h" 00002 #include "NokiaLCD.h" 00003 #include "PS2Keyboard.h" 00004 #include "ball.h" 00005 #include "paddle.h" 00006 00007 // State enumerator 00008 typedef enum { 00009 RESET, RUN, PAUSE 00010 } STATES; 00011 00012 NokiaLCD lcd(p5, p7, p8, p9, NokiaLCD::LCD6610); // mosi, sclk, cs, rst, type 00013 00014 PS2Keyboard ps2kb(p12, p11); // CLK, DAT 00015 00016 DigitalIn sw2(p24); 00017 DigitalIn sw1(p25); 00018 00019 PwmOut g(p21); 00020 PwmOut b(p22); 00021 PwmOut r(p23); 00022 00023 // Button enumerator for PS/2 keyboard 00024 enum BUTTONS{ 00025 UP = 0xe75, 00026 DOWN = 0xe72, 00027 }; 00028 00029 /* 00030 * Subroutine drawScreen: 00031 * Description: Draws both paddles 00032 * and the ball. 00033 */ 00034 void drawScreen(Paddle paddle1, Paddle paddle2, Ball theBall, bool isBlack) { 00035 paddle1.draw(lcd, isBlack); 00036 paddle2.draw(lcd ,isBlack); 00037 theBall.draw(lcd ,isBlack); 00038 } 00039 00040 /* 00041 * Subroutine drawScores: 00042 * Description: Draws the scoreboard 00043 */ 00044 void drawScores(Paddle paddle1, Paddle paddle2) { 00045 lcd.locate(7,0); 00046 lcd.putc('0' + paddle1.getScore()); 00047 lcd.locate(9,0); 00048 lcd.putc('0' + paddle2.getScore()); 00049 lcd.fill(66,0,2,130,0xFFFFFF); 00050 lcd.locate(7,15); 00051 lcd.putc('0' + paddle1.getLives()); 00052 lcd.locate(9,15); 00053 lcd.putc('0' + paddle2.getLives()); 00054 } 00055 00056 int main() { 00057 PS2Keyboard::keyboard_event_t evt_kb; // Setup keyboard interrupt 00058 lcd.background(0x000000); 00059 lcd.cls(); 00060 Paddle paddle1, paddle2; 00061 Ball theBall; 00062 int temp, count=0; 00063 drawScreen(paddle1, paddle2, theBall, false); 00064 drawScores(paddle1, paddle2); 00065 STATES state = RESET; // Initial state is RESET 00066 while(1) { 00067 switch(state) { 00068 case RESET: // Reset objects, draw the screen, state = PAUSE 00069 lcd.cls(); 00070 paddle1 = Paddle(1,10,5,25,0xFFFFFF,paddle1.getLives(),paddle1.getScore()); 00071 paddle2 = Paddle(125,3,5,25,0xFFFFFF,paddle2.getLives(),paddle2.getScore()); 00072 theBall = Ball(6,25,5,5,0xFFFF00,1,1); 00073 drawScreen(paddle1, paddle2, theBall, false); 00074 drawScores(paddle1, paddle2); 00075 state = PAUSE; 00076 break; 00077 case PAUSE: // Set RGB LED to Red, wait for switch input 00078 r = 0; 00079 b = g = 1; 00080 if(!sw2) { 00081 while(!sw2); 00082 paddle2.setLives(3); 00083 paddle1.setLives(3); 00084 state = RESET; 00085 break; 00086 } 00087 if(!sw1) { 00088 while(!sw1); 00089 state = RUN; 00090 } 00091 break; 00092 case RUN: // Set RGB LED to Blue and run program 00093 r = g = 1; 00094 b = 0; 00095 if(!sw2) { // Reset if SW2 is pressed 00096 while(!sw2); 00097 paddle2.setLives(3); 00098 paddle1.setLives(3); 00099 state = RESET; 00100 break; 00101 } 00102 if(!sw1) { // Pause if SW1 is pressed 00103 while(!sw1); 00104 state = PAUSE; 00105 break; 00106 } 00107 if (ps2kb.processing(&evt_kb)) { // Executes if a key is pressed 00108 temp = evt_kb.scancode[0]; 00109 for (int i = 1; i < evt_kb.length; i++) { // Parse keyboard input into a key 00110 temp <<= 4; 00111 temp |= evt_kb.scancode[i]; 00112 } 00113 switch(temp) { // Use key enumerator to move paddle1 00114 case UP: 00115 if(paddle1.getY()>2) 00116 paddle1.move(lcd, -2); 00117 break; 00118 case DOWN: 00119 if(paddle1.getY()+paddle1.getHeight()<128) 00120 paddle1.move(lcd, 2); 00121 break; 00122 } 00123 } 00124 if(count%2) // Only let CPU move once every 2 times through the loop 00125 paddle2.moveCPU(lcd, theBall.getY()); 00126 if(++count==5) { // Only move the ball once every 5 times through the loop 00127 count = 0; 00128 if(theBall.hitP1((paddle1.getX()+paddle1.getWidth()), paddle1.getY(), paddle1.getHeight())) 00129 theBall.reverseX(); 00130 if(theBall.hitP2(paddle2.getX(), paddle2.getY(), paddle2.getHeight())) 00131 theBall.reverseX(); 00132 if(theBall.hitX()) { // If the ball hits one of the sides of the screen 00133 if(theBall.getX()<7) { // If the ball hit paddle1's side 00134 if(!paddle1.loseLife()) { // If paddle1 has no more lives 00135 paddle1.setLives(3); 00136 paddle2.setLives(3); 00137 paddle2.addPoint(); 00138 } 00139 } 00140 else if(theBall.getX()>120) { // If the ball hit paddle2's side 00141 if(!paddle2.loseLife()) { // If paddle2 has no more lives 00142 paddle2.setLives(3); 00143 paddle1.setLives(3); 00144 paddle1.addPoint(); 00145 } 00146 } 00147 theBall.reverseX(); 00148 state = RESET; // Reset the objects 00149 } 00150 if(theBall.hitY()) 00151 theBall.reverseY(); 00152 theBall.move(lcd); 00153 } 00154 break; 00155 } 00156 drawScreen(paddle1, paddle2, theBall, false); 00157 drawScores(paddle1, paddle2); 00158 } 00159 }
Generated on Tue Jul 12 2022 18:11:13 by
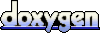