
Simple Pong game on NokiaLCD with PS2
Dependencies: mbed PS2 NokiaLCD
ball.cpp
00001 #include "mbed.h" 00002 #include "ball.h" 00003 00004 Ball::Ball() { 00005 int x=y=width=height=color=xInc=yInc=0; 00006 } 00007 00008 Ball::Ball(int x, int y, int w, int h, int c, int xi, int yi) 00009 : x(x), y(y), width(w), height(h), color(c), xInc(xi), yInc(yi) {} 00010 00011 00012 /* 00013 * Member Function move: 00014 * Description: Colors in the previous ball black 00015 * and moves ball to new position. 00016 */ 00017 void Ball::move(NokiaLCD &lcd) { 00018 draw(lcd, true); 00019 x += xInc; y += yInc; 00020 } 00021 00022 /* 00023 * Member Function draw: 00024 * Description: Draws object on screen 00025 * if isBlack, color in black. 00026 */ 00027 void Ball::draw(NokiaLCD &lcd, bool isBlack) const { 00028 lcd.fill(x, y, width, height, (isBlack) ? 0x000000 : color); 00029 } 00030 00031 int Ball::size() const { 00032 return width*height; 00033 } 00034 00035 int Ball::getX() const { 00036 return x; 00037 } 00038 00039 int Ball::getY() const { 00040 return y; 00041 } 00042 00043 bool Ball::hitX() { 00044 return (x+width<=width) || (x+width>=132); 00045 } 00046 00047 bool Ball::hitY() { 00048 return (y<=0) || (y+height>=130); 00049 } 00050 00051 /* 00052 * Member Function hitP1: 00053 * Description: Checks to see if there is 00054 * a collision between paddle1 and the ball. 00055 * Has special functionality for changing 00056 * y-incline based on collision point. 00057 */ 00058 bool Ball::hitP1(int _x, int _y, int _height) { 00059 bool hit = ((_x>=x) && (xInc<0)) && 00060 (((_y<=y) && (_y+_height>=y+height)) || 00061 ((_y>=y) && (_y<=y+height)) || 00062 ((_y+_height>=y) && (_y+_height<=y+height)) 00063 ); 00064 if(hit) { 00065 if(_y+_height-y < 4 && yInc>0) yInc = 2; 00066 if(y-_y < 4 && yInc<0) yInc = -2; 00067 else yInc = (yInc>0) ? 1 : -1; 00068 } 00069 return hit; 00070 } 00071 00072 00073 /* 00074 * Member Function hitP2: 00075 * Description: Checks to see if there is 00076 * a collision between paddle2 and the ball. 00077 * Has special functionality for changing 00078 * y-incline based on collision point. 00079 */ 00080 bool Ball::hitP2(int _x, int _y, int _height) { 00081 bool hit = ((_x<=x+width) && (xInc>0)) && 00082 (((_y<=y) && (_y+_height>=y+height)) || 00083 ((_y>=y) && (_y<=y+height)) || 00084 ((_y+_height>=y) && (_y+_height<=y+height)) 00085 ); 00086 if(hit) { 00087 if(_y+_height-y < 4 && yInc>0) yInc = 2; 00088 if(y-_y < 4 && yInc<0) yInc = -2; 00089 else yInc = (yInc>0) ? 1 : -1; 00090 } 00091 return hit; 00092 } 00093 00094 void Ball::reverseX() { 00095 xInc *= -1; 00096 } 00097 00098 void Ball::reverseY() { 00099 yInc *= -1; 00100 }
Generated on Tue Jul 12 2022 18:11:13 by
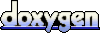