
hghfg
Fork of _library_jsmn by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include <ctype.h> 00002 00003 #include "mbed.h" 00004 00005 #include "jsmn.h" 00006 00007 #define MAXTOKEN 64 00008 00009 const char *jsmn_type_str[] = { 00010 "PRIMITIVE", 00011 "OBJECT", 00012 "ARRAY", 00013 "STRING" 00014 }; 00015 00016 00017 int main() 00018 { 00019 const char *js; // Pointer to json string 00020 int r; // Number of token parsed 00021 jsmn_parser p; // jsmn parser 00022 jsmntok_t t[MAXTOKEN]; // Parsed token 00023 00024 // JSON may contain multibyte characters or encoded unicode in 00025 // \uXXXX format. 00026 // mbed compiler may complain "invalid multibyte character sequence". 00027 js = 00028 "{" 00029 " \"menu\":" 00030 " {" 00031 " \"id\": 1234," 00032 " \"group\": \"File\"," 00033 " \"popup\":" 00034 " {" 00035 " \"menuitem\":" 00036 " [" 00037 " {\"value\": true, \"onclick\" : \"বিশাল\"}," 00038 " {\"value\": false, 0x1328 : \"groß\"}," 00039 " {\"value\": null, \"15\u00f8C\": \"3\u0111\"}," 00040 " {\"value\": \"測試\", -12.34 : 99}" 00041 " ]" 00042 " }" 00043 " }" 00044 "}"; 00045 00046 jsmn_init(&p); 00047 r = jsmn_parse(&p, js, strlen(js), t, MAXTOKEN); 00048 00049 printf("Parsed %d tokens\n", r); 00050 00051 printf(" TYPE START END SIZE PAR\n"); 00052 printf(" ---------- ----- ---- ---- ---\n"); 00053 00054 char ch; 00055 jsmntok_t at; // A token for general use 00056 for (int i = 0; i < r; i++) 00057 { 00058 at = t[i]; 00059 printf("Token %2d = %-10.10s (%4d - %4d, %3d, %2d) --> ", 00060 i, jsmn_type_str[at.type], 00061 at.start, at.end, 00062 at.size, at.parent); 00063 00064 switch (at.type) 00065 { 00066 case JSMN_STRING: 00067 printf("%-10.*s\n", at.end - at.start + 2, js + at.start - 1); 00068 break; 00069 00070 case JSMN_PRIMITIVE: 00071 ch = *(js + at.start); 00072 00073 if (isdigit(ch) || ch == '-') 00074 printf("%-10.*s\n", at.end - at.start, js + at.start); 00075 else if (tolower(ch) == 'n') 00076 printf("null\n"); 00077 else if (tolower(ch) == 't') 00078 printf("true\n"); 00079 else if (tolower(ch) == 'f') 00080 printf("false\n"); 00081 break; 00082 00083 default: 00084 printf("\n"); 00085 break; 00086 } 00087 } 00088 00089 while (1) 00090 ; 00091 }
Generated on Sat Jul 16 2022 02:00:27 by
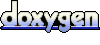