This package includes the SharkSSL lite library and header files.
Dependents: WebSocket-Client-Example SharkMQ-LED-Demo
seLwIP.c
00001 /** 00002 * ____ _________ __ _ 00003 * / __ \___ ____ _/ /_ __(_)___ ___ ___ / / ____ ____ _(_)____ 00004 * / /_/ / _ \/ __ `/ / / / / / __ `__ \/ _ \/ / / __ \/ __ `/ / ___/ 00005 * / _, _/ __/ /_/ / / / / / / / / / / / __/ /___/ /_/ / /_/ / / /__ 00006 * /_/ |_|\___/\__,_/_/ /_/ /_/_/ /_/ /_/\___/_____/\____/\__, /_/\___/ 00007 * /____/ 00008 * 00009 * SharkSSL Embedded SSL/TLS Stack 00010 **************************************************************************** 00011 * PROGRAM MODULE 00012 * 00013 * $Id: seLwIP.c 3871 2016-03-27 01:23:13Z wini $ 00014 * 00015 * COPYRIGHT: Real Time Logic LLC, 2014 - 2016 00016 * 00017 * This software is copyrighted by and is the sole property of Real 00018 * Time Logic LLC. All rights, title, ownership, or other interests in 00019 * the software remain the property of Real Time Logic LLC. This 00020 * software may only be used in accordance with the terms and 00021 * conditions stipulated in the corresponding license agreement under 00022 * which the software has been supplied. Any unauthorized use, 00023 * duplication, transmission, distribution, or disclosure of this 00024 * software is expressly forbidden. 00025 * 00026 * This Copyright notice may not be removed or modified without prior 00027 * written consent of Real Time Logic LLC. 00028 * 00029 * Real Time Logic LLC. reserves the right to modify this software 00030 * without notice. 00031 * 00032 * http://realtimelogic.com 00033 * http://sharkssl.com 00034 **************************************************************************** 00035 */ 00036 00037 00038 #include <selib.h> 00039 #include <lwip/opt.h> 00040 #include <lwip/arch.h> 00041 #include <lwip/api.h> 00042 00043 #ifndef SharkSSLLwIP 00044 #error SharkSSLLwIP not defined -> Using incorrect selibplat.h 00045 #endif 00046 00047 00048 #if LWIP_SO_RCVTIMEO != 1 00049 #error LWIP_SO_RCVTIMEO must be set 00050 #endif 00051 00052 #ifndef netconn_set_recvtimeout 00053 #define OLD_LWIP 00054 #define netconn_set_recvtimeout(conn, timeout) \ 00055 ((conn)->recv_timeout = (timeout)) 00056 #endif 00057 00058 00059 00060 00061 int se_accept(SOCKET** listenSock, U32 timeout, SOCKET** outSock) 00062 { 00063 err_t err; 00064 memset(*outSock, 0, sizeof(SOCKET)); 00065 netconn_set_recvtimeout( 00066 (*listenSock)->con, timeout == INFINITE_TMO ? 0 : timeout); 00067 #ifdef OLD_LWIP 00068 (*outSock)->con = netconn_accept((*listenSock)->con); 00069 err = (*outSock)->con->err; 00070 if(!(*outSock)->con && !err) err = ERR_CONN; 00071 #else 00072 err = netconn_accept((*listenSock)->con, &(*outSock)->con); 00073 #endif 00074 if(err != ERR_OK) 00075 { 00076 return err == ERR_TIMEOUT ? 0 : -1; 00077 } 00078 return 1; 00079 } 00080 00081 00082 int se_bind(SOCKET* sock, U16 port) 00083 { 00084 int err; 00085 memset(sock, 0, sizeof(SOCKET)); 00086 sock->con = netconn_new(NETCONN_TCP); 00087 if( ! sock->con ) 00088 return -1; 00089 if(netconn_bind(sock->con, IP_ADDR_ANY, port) == ERR_OK) 00090 { 00091 if(netconn_listen(sock->con) == ERR_OK) 00092 return 0; 00093 err = -2; 00094 } 00095 else 00096 err = -3; 00097 netconn_delete(sock->con); 00098 sock->con=0; 00099 return err; 00100 } 00101 00102 00103 00104 /* Returns 0 on success. 00105 Error codes returned: 00106 -1: Cannot create socket: Fatal 00107 -2: Cannot resolve 'address' 00108 -3: Cannot connect 00109 */ 00110 int se_connect(SOCKET* sock, const char* name, U16 port) 00111 { 00112 #ifdef OLD_LWIP 00113 struct ip_addr addr; 00114 #else 00115 ip_addr_t addr; 00116 #endif 00117 memset(sock, 0, sizeof(SOCKET)); 00118 if(netconn_gethostbyname(name, &addr) != ERR_OK) 00119 return -2; 00120 sock->con = netconn_new(NETCONN_TCP); 00121 if( ! sock->con ) 00122 return -1; 00123 if(netconn_connect(sock->con, &addr, port) == ERR_OK) 00124 return 0; 00125 netconn_delete(sock->con); 00126 sock->con=0; 00127 return -3; 00128 } 00129 00130 00131 00132 void se_close(SOCKET* sock) 00133 { 00134 if(sock->con) 00135 netconn_delete(sock->con); 00136 if(sock->nbuf) 00137 netbuf_delete(sock->nbuf); 00138 memset(sock, 0, sizeof(SOCKET)); 00139 } 00140 00141 00142 00143 S32 se_send(SOCKET* sock, const void* buf, U32 len) 00144 { 00145 err_t err=netconn_write(sock->con, buf, len, NETCONN_COPY); 00146 if(err != ERR_OK) 00147 { 00148 se_close(sock); 00149 return (S32)err; 00150 } 00151 return len; 00152 } 00153 00154 00155 00156 S32 se_recv(SOCKET* sock, void* data, U32 len, U32 timeout) 00157 { 00158 int rlen; 00159 netconn_set_recvtimeout(sock->con, timeout == INFINITE_TMO ? 0 : timeout); 00160 if( ! sock->nbuf ) 00161 { 00162 err_t err; 00163 sock->pbOffs = 0; 00164 #ifdef OLD_LWIP 00165 sock->nbuf = netconn_recv(sock->con); 00166 err = sock->con->err; 00167 if(!sock->nbuf && !err) err = ERR_CONN; 00168 #else 00169 err = netconn_recv(sock->con, &sock->nbuf); 00170 #endif 00171 if(ERR_OK != err) 00172 { 00173 if(sock->nbuf) 00174 netbuf_delete(sock->nbuf); 00175 sock->nbuf=0; 00176 return err == ERR_TIMEOUT ? 0 : (S32)err; 00177 } 00178 } 00179 rlen=(int)netbuf_copy_partial(sock->nbuf,(U8*)data,len,sock->pbOffs); 00180 if(!rlen) 00181 return -1; 00182 sock->pbOffs += rlen; 00183 if(sock->pbOffs >= netbuf_len(sock->nbuf)) 00184 { 00185 netbuf_delete(sock->nbuf); 00186 sock->nbuf=0; 00187 } 00188 return rlen; 00189 } 00190 00191 00192 00193 int se_sockValid(SOCKET* sock) 00194 { 00195 return sock->con != 0; 00196 }
Generated on Wed Jul 13 2022 10:54:53 by
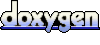