This package includes the SharkSSL lite library and header files.
Dependents: WebSocket-Client-Example SharkMQ-LED-Demo
WsClientLib.h
00001 /* 00002 * ____ _________ __ _ 00003 * / __ \___ ____ _/ /_ __(_)___ ___ ___ / / ____ ____ _(_)____ 00004 * / /_/ / _ \/ __ `/ / / / / / __ `__ \/ _ \/ / / __ \/ __ `/ / ___/ 00005 * / _, _/ __/ /_/ / / / / / / / / / / / __/ /___/ /_/ / /_/ / / /__ 00006 * /_/ |_|\___/\__,_/_/ /_/ /_/_/ /_/ /_/\___/_____/\____/\__, /_/\___/ 00007 * /____/ 00008 * 00009 * SharkSSL Embedded SSL/TLS Stack 00010 **************************************************************************** 00011 * PROGRAM MODULE 00012 * 00013 * $Id: WsClientLib.h 3616 2014-12-03 00:40:53Z wini $ 00014 * 00015 * COPYRIGHT: Real Time Logic LLC, 2013 00016 * 00017 * This software is copyrighted by and is the sole property of Real 00018 * Time Logic LLC. All rights, title, ownership, or other interests in 00019 * the software remain the property of Real Time Logic LLC. This 00020 * software may only be used in accordance with the terms and 00021 * conditions stipulated in the corresponding license agreement under 00022 * which the software has been supplied. Any unauthorized use, 00023 * duplication, transmission, distribution, or disclosure of this 00024 * software is expressly forbidden. 00025 * 00026 * This Copyright notice may not be removed or modified without prior 00027 * written consent of Real Time Logic LLC. 00028 * 00029 * Real Time Logic LLC. reserves the right to modify this software 00030 * without notice. 00031 * 00032 * http://realtimelogic.com 00033 * http://sharkssl.com 00034 **************************************************************************** 00035 * 00036 */ 00037 00038 #ifndef _WsClientLib_h 00039 #define _WsClientLib_h 00040 00041 00042 #include "selib.h" 00043 00044 /** @addtogroup WsClientLib 00045 @{ 00046 */ 00047 00048 /** @defgroup WebSocketOpcodes WebSocket Opcodes 00049 @ingroup WsClientLib 00050 00051 \brief WebSocket Opcodes 00052 00053 RFC6455 Page 29 - Opcode - 4 bits 00054 00055 WebSocket Opcodes with FIN=1. We do not manage WebSocket fragments 00056 (FIN=0/1) since it is rarely used and the complexity is not 00057 something you want in a tiny device. 00058 @{ 00059 */ 00060 00061 /** Text */ 00062 #define WSOP_Text 0x81 00063 /** Binary */ 00064 #define WSOP_Binary 0x82 00065 /** RFC6455 5.5 - Control Frame - Close */ 00066 #define WSOP_Close 0x88 00067 /** RFC6455 5.5 - Control Frame - Ping */ 00068 #define WSOP_Ping 0x89 00069 /** RFC6455 5.5 - Control Frame - Pong */ 00070 #define WSOP_Pong 0x8A 00071 00072 /** @} */ /* end group WebSocketOpcodes */ 00073 00074 00075 00076 /** The WebSocket protocol is frame based and the following struct keeps state 00077 information for #wscRead. 00078 */ 00079 typedef struct 00080 { 00081 /** The WebSocket frame length */ 00082 int frameLen; 00083 /** Read frame data until: frameLen - bytesRead = 0 */ 00084 int bytesRead; 00085 00086 U8* overflowPtr; /* Set if: consumed more data from stream than frame len */ 00087 int overflowLen; /* overflowPtr len is used internally in wsRawRead */ 00088 int frameHeaderIx; /* Cursor used when reading frameHeader from socket */ 00089 U8 frameHeader[4]; /*[0] FIN+opcode, [1] Payload len, [2-3] Ext payload len*/ 00090 00091 /** Set when the read function returns due to a timeout. */ 00092 U8 isTimeout; 00093 } WscReadState; 00094 00095 00096 #ifdef __cplusplus 00097 extern "C" { 00098 #endif 00099 00100 00101 /** Upgrades (morphs) an HTTPS request/response pair to a WebSocket 00102 connection. Sends the HTTP request header to the server and 00103 validates the server's HTTP response header -- the function 00104 simulates a very basic HTTP client library. The function is designed 00105 to be as simple as possible and the code is, for this reason, making 00106 a few assumptions that could fail when used with a non traditional 00107 HTTP server. Read the comments in the source code file WsClientLib.c 00108 if you should experience problems. 00109 00110 \param wss the WebSocket protocol state information is stored in this 00111 structure. All wss attributes must be initialized to zero before 00112 calling this function for the first time. 00113 \param s the SharkSslCon object 00114 \param sock the SOCKET object 00115 \param tmo in milliseconds. The timeout can be set to #INFINITE_TMO. 00116 \param host is the server's host name 00117 \param path is the path component of the wss URL and the path must 00118 be to the server's WebSocket service. 00119 \param origin some WebSocket server's may require an origin URL: 00120 http://tools.ietf.org/html/rfc6455#section-10.2. Set the parameter 00121 to NULL if it's not required by the server. The Origin header should 00122 only be required by a server when the request is sent from a 00123 browser. 00124 \return Zero success. 00125 */ 00126 int wscProtocolHandshake(WscReadState* wss,SharkSslCon *s,SOCKET* sock,U32 tmo, 00127 const char* host,const char* path,const char* origin); 00128 00129 00130 /** Sends binary data to server. 00131 00132 The function sets the WS frame header's opcode to binary. The WS 00133 protocol supports two payload frame types, UTF8 and binary (RFC6455: 00134 5.6 Data Frames). We are assuming that you will be using the binary 00135 protocol for all data exchange. 00136 */ 00137 int wscSendBin(SharkSslCon *s, SOCKET* sock, U8* buf, int len); 00138 00139 /** Sends a WebSocket control frame. 00140 00141 The code is used internally by the WebSocket functions. You can also use 00142 this function to send your own control frames such as #WSOP_Ping. 00143 00144 See RFC6455: 5.5. Control Frames 00145 */ 00146 int wscSendCtrl( 00147 SharkSslCon *s,SOCKET* sock,U8 opCode, const U8* buf,int len); 00148 00149 /** Sends a WebSocket close control frame to the server and closes the 00150 connection. 00151 00152 \param s the SharkSslCon object. 00153 \param sock the SOCKET object. 00154 00155 \param statusCode is a <a target="_blank" href= 00156 "http://tools.ietf.org/html/rfc6455#section-7.4"> 00157 WebSocket status code</a>. 00158 */ 00159 int wscClose(SharkSslCon *s, SOCKET* sock, int statusCode); 00160 00161 00162 /** Wait for WebSocket frames sent by the server. The function 00163 returns when data is available or on timeout. The function returns 00164 zero on timeout, but the peer can send zero length frames so you must 00165 verify that it is a timeout by checking the status of 00166 WscReadState#isTimeout. 00167 00168 The WebSocket protocol is frame based, but the function can return 00169 a fragment before the complete WebSocket frame is received if the frame 00170 sent by the peer is larger than the SharkSSL receive buffer. The 00171 frame length is returned in WscReadState#frameLen and the data 00172 consumed thus far is returned in WscReadState#bytesRead. The 00173 complete frame is consumed when frameLen == bytesRead. 00174 00175 \param wss is the WebSocket read state. 00176 00177 \param s the SharkSslCon object. 00178 \param sock the SOCKET object. 00179 00180 \param buf is a pointer set to the SharkSSL receive buffer offset to 00181 the start of the WebSocket payload data. 00182 00183 \param timeout in milliseconds. The timeout can be set to #INFINITE_TMO. 00184 00185 \return The payload data length or zero for zero length frames and 00186 timeout. The function returns a negative value on error. 00187 */ 00188 int wscRead( 00189 WscReadState* wss, SharkSslCon *s,SOCKET* sock, U8 **buf, U32 timeout); 00190 00191 #ifdef __cplusplus 00192 } 00193 #endif 00194 00195 /** @} */ /* end group WsClientLib */ 00196 00197 #endif
Generated on Wed Jul 13 2022 10:54:53 by
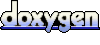