This package includes the SharkSSL lite library and header files.
Dependents: WebSocket-Client-Example SharkMQ-LED-Demo
SingleList.h
00001 /* 00002 * ____ _________ __ _ 00003 * / __ \___ ____ _/ /_ __(_)___ ___ ___ / / ____ ____ _(_)____ 00004 * / /_/ / _ \/ __ `/ / / / / / __ `__ \/ _ \/ / / __ \/ __ `/ / ___/ 00005 * / _, _/ __/ /_/ / / / / / / / / / / / __/ /___/ /_/ / /_/ / / /__ 00006 * /_/ |_|\___/\__,_/_/ /_/ /_/_/ /_/ /_/\___/_____/\____/\__, /_/\___/ 00007 * /____/ 00008 **************************************************************************** 00009 * HEADER 00010 * 00011 * $Id: SingleList.h 3215 2013-11-29 14:43:17Z wini $ 00012 * 00013 * COPYRIGHT: Real Time Logic, 2002 00014 * 00015 * This software is copyrighted by and is the sole property of Real 00016 * Time Logic LLC. All rights, title, ownership, or other interests in 00017 * the software remain the property of Real Time Logic LLC. This 00018 * software may only be used in accordance with the terms and 00019 * conditions stipulated in the corresponding license agreement under 00020 * which the software has been supplied. Any unauthorized use, 00021 * duplication, transmission, distribution, or disclosure of this 00022 * software is expressly forbidden. 00023 * 00024 * This Copyright notice may not be removed or modified without prior 00025 * written consent of Real Time Logic LLC. 00026 * 00027 * Real Time Logic LLC. reserves the right to modify this software 00028 * without notice. 00029 * 00030 * http://www.realtimelogic.com 00031 **************************************************************************** 00032 00033 CONTENTS 00034 -------- 00035 00036 1 Description 00037 2 History of developmentDoubleLink 00038 3 Macros 00039 4 Include files 00040 5 types Constants Variables 00041 6 Function prototypes 00042 00043 **************************************************************************** 00044 */ 00045 #ifndef _SingleList_h 00046 #define _SingleList_h 00047 00048 /* 00049 **************************************************************************** 00050 * 1 DESCRIPTION. 00051 **************************************************************************** 00052 * 00053 */ 00054 00055 /* 00056 **************************************************************************** 00057 * 00058 */ 00059 00060 /* 00061 **************************************************************************** 00062 * 3 MACROS. 00063 **************************************************************************** 00064 */ 00065 00066 /* 00067 **************************************************************************** 00068 * 4 INCLUDE FILES. 00069 **************************************************************************** 00070 */ 00071 #include <TargConfig.h> 00072 00073 00074 /* 00075 **************************************************************************** 00076 * 5 TYPES CONSTANTS VARIABLES 00077 **************************************************************************** 00078 */ 00079 00080 /*Forward declarations*/ 00081 struct SingleList; 00082 struct SingleListEnumerator; 00083 00084 /*=========================================================================== 00085 * 00086 * Class: SingleLink 00087 *--------------------------------------------------------------------------- 00088 * Description: 00089 * Contains the link chain for the next element in the SingleList. Subclass 00090 * o link for data stored in the SingleList. 00091 * Note, o class contains no virtual destructor and the subclassed node 00092 * will not be informed when the node is deleted. 00093 */ 00094 typedef struct SingleLink 00095 { 00096 #ifdef __cplusplus 00097 SingleLink(); 00098 SingleLink* getNext(); 00099 private: 00100 friend struct SingleList; 00101 friend struct SingleListEnumerator; 00102 #endif 00103 struct SingleLink* next; 00104 } SingleLink; 00105 #define SingleLink_constructor(o) ((SingleLink*)(o))->next = 0 00106 #define SingleLink_getNext(o) ((SingleLink*)(o))->next 00107 #define SingleLink_isLinked(o) \ 00108 (((SingleLink*)o)->next ? TRUE : FALSE) 00109 00110 #ifdef __cplusplus 00111 inline SingleLink::SingleLink() {SingleLink_constructor(this);} 00112 inline SingleLink* SingleLink::getNext() {return SingleLink_getNext(this);} 00113 #endif 00114 00115 /*=========================================================================== 00116 * 00117 * Class: SingleList 00118 *--------------------------------------------------------------------------- 00119 * Description: 00120 * Contains nodes of type SingleLink. 00121 */ 00122 typedef struct SingleList 00123 { 00124 #ifdef __cplusplus 00125 SingleList(); 00126 void insertLast(SingleLink* link); 00127 SingleLink* removeFirst(); 00128 SingleLink* peekFirst(); 00129 bool isEmpty(); 00130 bool isLast(SingleLink* link); 00131 private: 00132 friend struct SingleListEnumerator; 00133 #endif 00134 SingleLink link; 00135 SingleLink* last; 00136 } SingleList; 00137 00138 #define SingleList_insertLast(o, linkMA) do \ 00139 { \ 00140 baAssert((SingleLink*)(linkMA) != (SingleLink*)(o)); \ 00141 baAssert(((SingleLink*)(linkMA))->next == 0); \ 00142 (o)->last->next = (SingleLink*)(linkMA); \ 00143 (o)->last = (SingleLink*)(linkMA); \ 00144 ((SingleLink*)(linkMA))->next = (SingleLink*)(o); \ 00145 } while(0) 00146 00147 #define SingleList_peekFirst(o) ((o)->link.next == (SingleLink*)(o) ? 0 : (o)->link.next) 00148 00149 #define SingleList_isEmpty(o) ((o)->link.next == (SingleLink*)(o)) 00150 #define SingleList_isLast(o, n) ((n)->next == (SingleLink*)(o)) 00151 00152 #ifdef __cplusplus 00153 extern "C" { 00154 #endif 00155 BA_API void SingleList_constructor(SingleList* o); 00156 BA_API SingleLink* SingleList_removeFirst(SingleList* o); 00157 #ifdef __cplusplus 00158 } 00159 inline 00160 SingleList::SingleList() { SingleList_constructor(this); } 00161 inline void 00162 SingleList::insertLast(SingleLink* link) { SingleList_insertLast(this, link); } 00163 inline SingleLink* 00164 SingleList::peekFirst() { return SingleList_peekFirst(this); } 00165 inline bool 00166 SingleList::isEmpty() { return SingleList_isEmpty(this); } 00167 inline bool 00168 SingleList::isLast(SingleLink* link) { return SingleList_isLast(this,link); } 00169 inline SingleLink* 00170 SingleList::removeFirst() { return SingleList_removeFirst(this); } 00171 #endif 00172 00173 /*=========================================================================== 00174 * 00175 * Class: SingleListEnumerator 00176 *--------------------------------------------------------------------------- 00177 * Description: 00178 * Usage: 00179 * SingleListEnumerator e(list); 00180 * for(Slink* link = e.getElement() ; link ; link = e.nextElement()) 00181 * or 00182 * SingleListEnumerator e(list); 00183 * Slink* link = e.getElement(); 00184 * while(link) 00185 * { 00186 * if(link bla bla) 00187 * //Deletes current element and returns next element 00188 * link = e.deleteElement(); 00189 * else 00190 * link = e.nextElement(); 00191 * } 00192 */ 00193 typedef struct SingleListEnumerator 00194 { 00195 #ifdef __cplusplus 00196 SingleListEnumerator(){} 00197 SingleListEnumerator(SingleList* list); 00198 SingleLink* getElement(); 00199 SingleLink* nextElement(); 00200 SingleLink* removeElement(); 00201 int insertBefore(SingleLink* l); 00202 private: 00203 #endif 00204 SingleList* list; 00205 SingleLink* prevElement; 00206 SingleLink* curElement; 00207 } SingleListEnumerator; 00208 00209 #define SingleListEnumerator_constructor(o, listMA) do \ 00210 { \ 00211 (o)->list = listMA; \ 00212 (o)->prevElement = (SingleLink*)listMA; \ 00213 (o)->curElement = SingleList_isEmpty(listMA) ? 0 : (o)->list->link.next; \ 00214 } while(0) 00215 00216 #define SingleListEnumerator_getElement(o) (o)->curElement 00217 00218 #define SingleListEnumerator_nextElement(o) \ 00219 ((o)->curElement ? ( \ 00220 (o)->prevElement = (o)->prevElement->next, \ 00221 (o)->curElement = (o)->curElement == (o)->list->last ? 0 : (o)->curElement->next, \ 00222 (o)->curElement \ 00223 ) : 0) 00224 00225 #ifdef __cplusplus 00226 extern "C" { 00227 #endif 00228 BA_API int SingleListEnumerator_insertBefore( 00229 SingleListEnumerator*, SingleLink*); 00230 BA_API SingleLink* SingleListEnumerator_removeElement(SingleListEnumerator* o); 00231 #ifdef __cplusplus 00232 } 00233 inline SingleListEnumerator::SingleListEnumerator(SingleList* list) { 00234 SingleListEnumerator_constructor(this, list); } 00235 inline SingleLink* 00236 SingleListEnumerator::removeElement() { 00237 return SingleListEnumerator_removeElement(this); } 00238 inline SingleLink* 00239 SingleListEnumerator::getElement() {return SingleListEnumerator_getElement(this);} 00240 inline SingleLink* 00241 SingleListEnumerator::nextElement() {return SingleListEnumerator_nextElement(this); } 00242 inline int SingleListEnumerator::insertBefore(SingleLink* l) { 00243 return SingleListEnumerator_insertBefore(this, l); } 00244 #endif 00245 00246 00247 #endif /*_SingleList_h*/ 00248 00249 00250 #if defined(SingleListCode) && ! defined(SingleListCodeIncluded) 00251 #define SingleListCodeIncluded 00252 00253 BA_API void 00254 SingleList_constructor(SingleList* o) 00255 { 00256 SingleLink_constructor((SingleLink*)o); 00257 o->last = ((SingleLink*)o); 00258 o->last->next = ((SingleLink*)o); 00259 o->link.next = ((SingleLink*)o); 00260 } 00261 00262 00263 BA_API int 00264 SingleListEnumerator_insertBefore(SingleListEnumerator* o, SingleLink* l) 00265 { 00266 if(l->next) 00267 return -1; 00268 00269 if(SingleList_isEmpty(o->list)) 00270 SingleList_insertLast(o->list, l); 00271 else 00272 { 00273 l->next = o->prevElement->next; 00274 o->prevElement->next = l; 00275 } 00276 o->prevElement = l; 00277 return 0; 00278 } 00279 00280 00281 BA_API SingleLink* 00282 SingleListEnumerator_removeElement(SingleListEnumerator* o) 00283 { 00284 if(o->curElement) 00285 { 00286 /*Store current position and iterate iterator*/ 00287 SingleLink* cur = o->curElement; 00288 /*If element to remove is last element in list*/ 00289 if(cur == o->list->last) 00290 { 00291 o->curElement = 0; 00292 o->list->last = o->prevElement; 00293 o->prevElement->next = (SingleLink*)o->list; 00294 } 00295 else 00296 { 00297 o->curElement = o->curElement->next; 00298 o->prevElement->next = o->curElement; 00299 } 00300 cur->next = 0; 00301 } 00302 return o->curElement; 00303 } 00304 00305 00306 BA_API SingleLink* 00307 SingleList_removeFirst(SingleList* o) 00308 { 00309 SingleLink* link2Remove; 00310 link2Remove = o->link.next; 00311 if(o->link.next == o->last) 00312 { 00313 if(o->link.next == (SingleLink*)o) 00314 return 0; 00315 o->link.next = o->last = (SingleLink*)o; 00316 } 00317 else 00318 o->link.next = o->link.next->next; 00319 link2Remove->next = 0; 00320 return link2Remove; 00321 } 00322 00323 #endif /* SingleListCode */
Generated on Wed Jul 13 2022 10:54:53 by
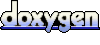