This package includes the SharkSSL lite library and header files.
Dependents: WebSocket-Client-Example SharkMQ-LED-Demo
SharkSslEx.h
00001 /* 00002 * ____ _________ __ _ 00003 * / __ \___ ____ _/ /_ __(_)___ ___ ___ / / ____ ____ _(_)____ 00004 * / /_/ / _ \/ __ `/ / / / / / __ `__ \/ _ \/ / / __ \/ __ `/ / ___/ 00005 * / _, _/ __/ /_/ / / / / / / / / / / / __/ /___/ /_/ / /_/ / / /__ 00006 * /_/ |_|\___/\__,_/_/ /_/ /_/_/ /_/ /_/\___/_____/\____/\__, /_/\___/ 00007 * /____/ 00008 * 00009 * SharkSSL Embedded SSL/TLS Stack 00010 **************************************************************************** 00011 * PROGRAM MODULE 00012 * 00013 * $Id: SharkSslEx.h 3670 2015-03-28 21:25:15Z gianluca $ 00014 * 00015 * COPYRIGHT: Real Time Logic LLC, 2013 00016 * 00017 * This software is copyrighted by and is the sole property of Real 00018 * Time Logic LLC. All rights, title, ownership, or other interests in 00019 * the software remain the property of Real Time Logic LLC. This 00020 * software may only be used in accordance with the terms and 00021 * conditions stipulated in the corresponding license agreement under 00022 * which the software has been supplied. Any unauthorized use, 00023 * duplication, transmission, distribution, or disclosure of this 00024 * software is expressly forbidden. 00025 * 00026 * This Copyright notice may not be removed or modified without prior 00027 * written consent of Real Time Logic LLC. 00028 * 00029 * Real Time Logic LLC. reserves the right to modify this software 00030 * without notice. 00031 * 00032 * http://www.realtimelogic.com 00033 * http://www.sharkssl.com 00034 **************************************************************************** 00035 * 00036 */ 00037 #ifndef _SharkSslEx_h 00038 #define _SharkSslEx_h 00039 00040 #include "SharkSSL.h" 00041 00042 00043 /** Case insensitive string compare. 00044 */ 00045 int sharkStrCaseCmp(const char *a, const char *b, int len); 00046 00047 /** @addtogroup SharkSslInfoAndCodes 00048 @{ 00049 */ 00050 00051 /** #SharkSslCon_trusted return values */ 00052 typedef enum 00053 { 00054 /** Not a secure connection (SSL handshake not completed). 00055 */ 00056 SharkSslConTrust_NotSSL=10, 00057 00058 /** The SSL certificate is not trusted and the subject's common 00059 name does not matches the host name of the URL. 00060 */ 00061 SharkSslConTrust_None, 00062 00063 /** Domain mismatch: The SSL certificate is trusted but the 00064 subject's common name does not matches the host name of the URL. 00065 */ 00066 SharkSslConTrust_Cert, 00067 00068 /** The subject's common name matches the host name of the URL, but 00069 the certificate is not trusted. This is typical for expired 00070 certificates. 00071 */ 00072 SharkSslConTrust_Cn, 00073 00074 /** The peer's SSL certificate is trusted and the 00075 subject's common name matches the host name of the URL. 00076 */ 00077 SharkSslConTrust_CertCn 00078 } SharkSslConTrust; 00079 00080 /** @} */ /* end group SharkSslInfoAndCodes */ 00081 00082 /** @addtogroup SharkSslApi 00083 @{ 00084 */ 00085 00086 /** Returns the peer's "trust" status and certificate. 00087 00088 \param o the SharkSslCon object 00089 00090 \param name is the domain name (common name) 00091 00092 \param cPtr is an optional pointer that will be set to the 00093 connections's SharkSslCertInfo object, if provided. 00094 00095 \returns SharkSslConTrust 00096 00097 \sa SharkSslConTrust and SharkSslCon_trustedCA 00098 */ 00099 SHARKSSL_API SharkSslConTrust SharkSslCon_trusted( 00100 SharkSslCon* o, const char* name, SharkSslCertInfo** cPtr); 00101 00102 /** @} */ /* end group SharkSslApi */ 00103 00104 #endif
Generated on Wed Jul 13 2022 10:54:53 by
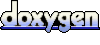