This package includes the SharkSSL lite library and header files.
Dependents: WebSocket-Client-Example SharkMQ-LED-Demo
DoubleList.h
00001 /* 00002 * ____ _________ __ _ 00003 * / __ \___ ____ _/ /_ __(_)___ ___ ___ / / ____ ____ _(_)____ 00004 * / /_/ / _ \/ __ `/ / / / / / __ `__ \/ _ \/ / / __ \/ __ `/ / ___/ 00005 * / _, _/ __/ /_/ / / / / / / / / / / / __/ /___/ /_/ / /_/ / / /__ 00006 * /_/ |_|\___/\__,_/_/ /_/ /_/_/ /_/ /_/\___/_____/\____/\__, /_/\___/ 00007 * /____/ 00008 * 00009 * Barracuda Embedded Web-Server 00010 * 00011 **************************************************************************** 00012 * HEADER 00013 * 00014 * $Id: DoubleList.h 2881 2013-04-22 22:34:04Z wini $ 00015 * 00016 * COPYRIGHT: Real Time Logic, 2004 - 2012 00017 * 00018 * This software is copyrighted by and is the sole property of Real 00019 * Time Logic LLC. All rights, title, ownership, or other interests in 00020 * the software remain the property of Real Time Logic LLC. This 00021 * software may only be used in accordance with the terms and 00022 * conditions stipulated in the corresponding license agreement under 00023 * which the software has been supplied. Any unauthorized use, 00024 * duplication, transmission, distribution, or disclosure of this 00025 * software is expressly forbidden. 00026 * 00027 * This Copyright notice may not be removed or modified without prior 00028 * written consent of Real Time Logic LLC. 00029 * 00030 * Real Time Logic LLC. reserves the right to modify this software 00031 * without notice. 00032 * 00033 * http://www.realtimelogic.com 00034 **************************************************************************** 00035 * 00036 * DiskIo implements the abstract class IoIntf. See the reference 00037 * manual for more information on the IoIntf (IO interface) 00038 * requirements. 00039 * 00040 * This is a generic header file for all file systems and 00041 * platforms. See the sub-directories for platform specific 00042 * implementations. 00043 */ 00044 00045 #ifndef _DoubleList_h 00046 #define _DoubleList_h 00047 00048 #include <TargConfig.h> 00049 00050 struct DoubleList; 00051 00052 typedef struct DoubleLink 00053 { 00054 #ifdef __cplusplus 00055 void *operator new(size_t s) { return ::baMalloc(s); } 00056 void operator delete(void* d) { if(d) ::baFree(d); } 00057 void *operator new(size_t, void *place) { return place; } 00058 void operator delete(void*, void *) { } 00059 DoubleLink(); 00060 ~DoubleLink(); 00061 void insertAfter(DoubleLink* newLink); 00062 void insertBefore(DoubleLink* newLink); 00063 void unlink(); 00064 bool isLinked(); 00065 DoubleLink* getNext(); 00066 #endif 00067 struct DoubleLink* next; 00068 struct DoubleLink* prev; 00069 } DoubleLink; 00070 00071 00072 00073 typedef struct DoubleList 00074 { 00075 #ifdef __cplusplus 00076 DoubleList(); 00077 void insertFirst(DoubleLink* newLink); 00078 void insertLast(DoubleLink* newLink); 00079 bool isLast(DoubleLink* n); 00080 DoubleLink* firstNode(); 00081 DoubleLink* lastNode(); 00082 bool isEmpty(); 00083 DoubleLink* removeFirst(); 00084 bool isInList(DoubleLink* n); 00085 #endif 00086 DoubleLink* next; 00087 DoubleLink* prev; 00088 } DoubleList; 00089 00090 00091 #define DoubleLink_constructor(o) do { \ 00092 ((DoubleLink*)o)->next = 0; \ 00093 ((DoubleLink*)o)->prev = 0; \ 00094 } while(0) 00095 00096 00097 #define DoubleLink_destructor(o) do { \ 00098 if(DoubleLink_isLinked(o)) \ 00099 DoubleLink_unlink(o); \ 00100 } while(0) 00101 00102 #define DoubleLink_insertAfter(o, newLink) do { \ 00103 baAssert(((DoubleLink*)newLink)->prev==0&&((DoubleLink*)newLink)->next==0);\ 00104 ((DoubleLink*)newLink)->next = ((DoubleLink*)o)->next; \ 00105 ((DoubleLink*)newLink)->prev = ((DoubleLink*)o); \ 00106 ((DoubleLink*)o)->next->prev = ((DoubleLink*)newLink); \ 00107 ((DoubleLink*)o)->next = ((DoubleLink*)newLink); \ 00108 } while(0) 00109 00110 00111 #define DoubleLink_insertBefore(o, newLink) do { \ 00112 baAssert(((DoubleLink*)newLink)->prev==0&&((DoubleLink*)newLink)->next==0);\ 00113 ((DoubleLink*)newLink)->prev = ((DoubleLink*)o)->prev; \ 00114 ((DoubleLink*)newLink)->next = ((DoubleLink*)o); \ 00115 ((DoubleLink*)o)->prev->next = ((DoubleLink*) newLink); \ 00116 ((DoubleLink*)o)->prev = ((DoubleLink*) newLink); \ 00117 } while(0) 00118 00119 00120 #define DoubleLink_unlink(o) do { \ 00121 baAssert(((DoubleLink*)o)->prev && ((DoubleLink*)o)->next);\ 00122 ((DoubleLink*) o)->next->prev = ((DoubleLink*)o)->prev; \ 00123 ((DoubleLink*) o)->prev->next = ((DoubleLink*)o)->next; \ 00124 ((DoubleLink*) o)->next = 0; \ 00125 ((DoubleLink*) o)->prev = 0; \ 00126 } while(0) 00127 00128 #ifdef NDEBUG 00129 #define DoubleLink_isLinked(o) \ 00130 (((DoubleLink*)o)->prev ? TRUE : FALSE) 00131 #else 00132 #define DoubleLink_isLinked(o) \ 00133 (((DoubleLink*)o)->prev ? (baAssert(((DoubleLink*)o)->next), TRUE) : FALSE) 00134 #endif 00135 00136 #define DoubleLink_getNext(o) ((DoubleLink*)(o))->next 00137 00138 00139 #define DoubleList_constructor(o) do { \ 00140 (o)->next = (DoubleLink*)o; \ 00141 (o)->prev = (DoubleLink*)o; \ 00142 } while(0) 00143 00144 00145 #define DoubleList_insertFirst(o, newLink) do { \ 00146 baAssert(((DoubleLink*)newLink)->prev==0&&((DoubleLink*)newLink)->next==0);\ 00147 ((DoubleLink*)newLink)->next = (o)->next; \ 00148 ((DoubleLink*)newLink)->prev = (DoubleLink*)o; \ 00149 (o)->next->prev = ((DoubleLink*) newLink); \ 00150 (o)->next = ((DoubleLink*) newLink); \ 00151 } while(0) 00152 00153 00154 #define DoubleList_insertLast(o, newLink) do { \ 00155 baAssert(((DoubleLink*)newLink)->prev==0&&((DoubleLink*)newLink)->next==0);\ 00156 ((DoubleLink*)newLink)->next = (DoubleLink*)o; \ 00157 ((DoubleLink*)newLink)->prev = (o)->prev; \ 00158 (o)->prev->next = ((DoubleLink*)newLink); \ 00159 (o)->prev = ((DoubleLink*)newLink); \ 00160 } while(0) 00161 00162 00163 #define DoubleList_isLast(o, n) (((DoubleLink*)(n))->next == (DoubleLink*)(o)) 00164 #define DoubleList_isEnd(o, n) ((DoubleLink*)(n) == (DoubleLink*)(o)) 00165 00166 #define DoubleList_firstNode(o) \ 00167 ((o)->next != (DoubleLink*)o ? (o)->next : 0) 00168 00169 00170 #define DoubleList_lastNode(o) \ 00171 ((o)->prev != (DoubleLink*)o ? (o)->prev : 0) 00172 00173 00174 00175 #define DoubleList_isEmpty(o) \ 00176 (((DoubleLink*)(o))->next == (DoubleLink*)(o)) 00177 00178 00179 #ifdef __cplusplus 00180 extern "C" { 00181 #endif 00182 00183 BA_API DoubleLink* DoubleList_removeFirst(DoubleList* o); 00184 00185 /* Returns true if the node is in any list. You cannot 00186 * use this function for testing if the node is in a particular list, that 00187 * is your problem. The function performs some additional tests if 00188 * NDEBUG is not defined and asserts that if the node is in a list, it should 00189 * be in this list. 00190 */ 00191 #ifdef NDEBUG 00192 #define DoubleList_isInList(o, node) (((DoubleLink*)node)->prev ? TRUE : FALSE) 00193 #else 00194 #define DoubleList_isInList(o, node) DoubleList_isInListF(o, node, __FILE__, __LINE__) 00195 BA_API BaBool DoubleList_isInListF(DoubleList* o,void* node,const char* file,int line); 00196 #endif 00197 00198 00199 #ifdef __cplusplus 00200 } 00201 inline DoubleLink::DoubleLink() { 00202 DoubleLink_constructor(this); 00203 } 00204 inline DoubleLink::~DoubleLink() { 00205 DoubleLink_destructor(this); 00206 } 00207 inline void DoubleLink::insertAfter(DoubleLink* newLink) { 00208 DoubleLink_insertAfter(this, newLink); 00209 } 00210 inline void DoubleLink::insertBefore(DoubleLink* newLink) { 00211 DoubleLink_insertBefore(this, newLink); 00212 } 00213 inline void DoubleLink::unlink() { 00214 DoubleLink_unlink(this); 00215 } 00216 inline bool DoubleLink::isLinked() { 00217 return DoubleLink_isLinked(this) ? true : false; 00218 } 00219 inline DoubleLink* DoubleLink::getNext() { 00220 return DoubleLink_getNext(this); 00221 } 00222 inline DoubleList::DoubleList() { 00223 DoubleList_constructor(this); 00224 } 00225 inline void DoubleList::insertFirst(DoubleLink* newLink) { 00226 DoubleList_insertFirst(this, newLink); 00227 } 00228 inline void DoubleList::insertLast(DoubleLink* newLink) { 00229 DoubleList_insertLast(this, newLink); 00230 } 00231 inline bool DoubleList::isLast(DoubleLink* n) { 00232 return DoubleList_isLast(this, n) ? true : false; 00233 } 00234 inline DoubleLink* DoubleList::firstNode() { 00235 return DoubleList_firstNode(this); 00236 } 00237 inline DoubleLink* DoubleList::lastNode() { 00238 return DoubleList_lastNode(this); 00239 } 00240 inline bool DoubleList::isEmpty() { 00241 return DoubleList_isEmpty(this) ? true : false; 00242 } 00243 inline DoubleLink* DoubleList::removeFirst() { 00244 return DoubleList_removeFirst(this); 00245 } 00246 inline bool DoubleList::isInList(DoubleLink* n) { 00247 return DoubleList_isInList(this, n) ? true : false; 00248 } 00249 #endif 00250 00251 00252 00253 00254 /*=========================================================================== 00255 * 00256 * Class: DoubleListEnumerator 00257 *--------------------------------------------------------------------------- 00258 * Description: 00259 * Usage: 00260 * DoubleListEnumerator e(list); 00261 * for(DoubleLink* link = e.getElement() ; link ; link = e.nextElement()) 00262 * or 00263 * DoubleListEnumerator e(list); 00264 * DoubleLink* link = e.getElement(); 00265 * while(link) 00266 * { 00267 * if(link bla bla) 00268 * //Deletes current element and returns next element 00269 * link = e.deleteElement(); 00270 * else 00271 * link = e.nextElement(); 00272 * } 00273 */ 00274 typedef struct DoubleListEnumerator 00275 { 00276 #ifdef __cplusplus 00277 DoubleListEnumerator(){} 00278 DoubleListEnumerator(DoubleList* list); 00279 DoubleLink* getElement(); 00280 DoubleLink* nextElement(); 00281 DoubleLink* removeElement(); 00282 private: 00283 #endif 00284 DoubleList* list; 00285 DoubleLink* curElement; 00286 } DoubleListEnumerator; 00287 00288 #define DoubleListEnumerator_constructor(o, listMA) do \ 00289 { \ 00290 (o)->list = listMA; \ 00291 (o)->curElement = DoubleList_firstNode((o)->list);\ 00292 } while(0) 00293 00294 #define DoubleListEnumerator_getElement(o) (o)->curElement 00295 00296 #define DoubleListEnumerator_nextElement(o) \ 00297 ((o)->curElement ? ( \ 00298 (o)->curElement = (o)->curElement->next == (DoubleLink*)(o)->list ? 0 : (o)->curElement->next, \ 00299 (o)->curElement \ 00300 ) : 0) 00301 00302 #ifdef __cplusplus 00303 extern "C" { 00304 #endif 00305 BA_API DoubleLink* DoubleListEnumerator_removeElement(DoubleListEnumerator* o); 00306 #ifdef __cplusplus 00307 } 00308 inline DoubleListEnumerator::DoubleListEnumerator(DoubleList* list) { 00309 DoubleListEnumerator_constructor(this, list); } 00310 inline DoubleLink* 00311 DoubleListEnumerator::removeElement() { 00312 return DoubleListEnumerator_removeElement(this); } 00313 inline DoubleLink* 00314 DoubleListEnumerator::getElement() {return DoubleListEnumerator_getElement(this);} 00315 inline DoubleLink* 00316 DoubleListEnumerator::nextElement() {return DoubleListEnumerator_nextElement(this); } 00317 #endif 00318 00319 00320 #endif
Generated on Wed Jul 13 2022 10:54:53 by
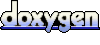