
Test for HCMS2975 Alphanumeric LED display
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
Go to the documentation of this file.
00001 /** 00002 * @file main.cpp 00003 * @brief mbed Avago/HP HCMS2975 LED matrix display Library test. 00004 * @author WH 00005 * @date Copyright (c) 2014 00006 * v01: WH, Initial release 00007 * Info available at http://playground.arduino.cc/Main/LedDisplay and http://www.pjrc.com/teensy/td_libs_LedDisplay.html 00008 */ 00009 #include "mbed.h" 00010 #include "HCMS2975.h" 00011 00012 // mbed Interface Hardware definitions 00013 DigitalOut myled1(LED1); 00014 DigitalOut myled2(LED2); 00015 DigitalOut myled3(LED3); 00016 DigitalOut heartbeatLED(LED4); 00017 00018 // Host PC Communication channels 00019 Serial pc(USBTX, USBRX); 00020 00021 // SPI Communication 00022 SPI spi_led(p5, NC, p7); // MOSI, MISO, SCLK 00023 00024 //Display 00025 //HCMS2975 led(&spi_led, p8, p9, NC, HCMS2975::LED8x1); // SPI bus, CS pin, RS pin, RST pin, LEDType = Ok 00026 //HCMS2975 led(&spi_led, p8, p9, NC, HCMS2975::LED16x1); // SPI bus, CS pin, RS pin, RST pin, LEDType = Ok 00027 //HCMS2975 led(&spi_led, p8, p20, NC, HCMS2975::LED8x1); // SPI bus, CS pin, RS pin, RST pin, LEDType = Ok 00028 //HCMS2975 led(&spi_led, p8, p20, NC, HCMS2975::LED8x2); // SPI bus, CS pin, RS pin, RST pin, LEDType = Ok 00029 //HCMS2975 led(&spi_led, p8, p20, NC, HCMS2975::LED16x1); // SPI bus, CS pin, RS pin, RST pin, LEDType = Ok 00030 HCMS2975 led(&spi_led, p8, p20, NC, HCMS2975::LED16x2); // SPI bus, CS pin, RS pin, RST pin, LEDType = Ok 00031 00032 // Variables for Heartbeat and Status monitoring 00033 Ticker heartbeat; 00034 bool heartbeatflag=false; 00035 00036 // Heartbeat monitor 00037 void pulse() { 00038 heartbeatLED = !heartbeatLED; 00039 } 00040 00041 void heartbeat_start() { 00042 heartbeat.attach(&pulse, 0.5); 00043 heartbeatflag = true; 00044 } 00045 00046 void heartbeat_stop() { 00047 heartbeat.detach(); 00048 heartbeatflag = false; 00049 } 00050 00051 int main() { 00052 int cnt; 00053 00054 pc.printf("Hello mbed from HCMS2975\n\r"); 00055 00056 heartbeat_start(); 00057 00058 led.locate(0, 0); 00059 00060 //led.printf("*=%6d", 123456); 00061 // 12345678 00062 led.printf("Hi mbed "); 00063 wait(2); 00064 00065 led.setUDC(0, (char *) udc_0); 00066 led.setUDC(1, (char *) udc_1); 00067 led.setUDC(2, (char *) udc_2); 00068 led.setUDC(3, (char *) udc_3); 00069 led.setUDC(4, (char *) udc_Bat_Hi); 00070 led.setUDC(5, (char *) udc_Bat_Ha); 00071 led.setUDC(6, (char *) udc_Bat_Lo); 00072 // led.setUDC(7, (char *) udc_smiley); 00073 led.setUDC(7, (char *) udc_AC); 00074 00075 led.putc(0); 00076 led.putc(1); 00077 led.putc(2); 00078 led.putc(3); 00079 led.putc(4); 00080 led.putc(5); 00081 led.putc(6); 00082 led.putc(7); 00083 wait(2); 00084 00085 led.setBrightness(HCMS2975_BRIGHT_3_3); 00086 00087 cnt=0x20; 00088 while(1) { 00089 wait(0.25); 00090 00091 led.putc(cnt); 00092 pc.putc('#'); 00093 cnt++; 00094 if (cnt == 0x80) cnt=0x20; 00095 } 00096 00097 }
Generated on Fri Jul 15 2022 04:32:36 by
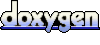