
USB HID Device that emulates a Gamecontroller
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "USBMouse.h" 00003 #include "USBJoystick.h" 00004 00005 //USBMouse mouse; 00006 USBJoystick joystick; 00007 00008 // Variables for Heartbeat and Status monitoring 00009 DigitalOut myled1(LED1); 00010 DigitalOut myled2(LED2); 00011 DigitalOut myled3(LED3); 00012 DigitalOut heartbeatLED(LED4); 00013 00014 Ticker heartbeat; 00015 Serial pc(USBTX, USBRX); // tx, rx 00016 00017 // Heartbeat monitor 00018 void pulse() { 00019 heartbeatLED = !heartbeatLED; 00020 } 00021 00022 void heartbeat_start() { 00023 heartbeat.attach(&pulse, 0.5); 00024 } 00025 00026 void heartbeat_stop() { 00027 heartbeat.detach(); 00028 } 00029 00030 00031 int main() { 00032 int16_t i = 0; 00033 int16_t throttle = 0; 00034 int16_t rudder = 0; 00035 int16_t x = 0; 00036 int16_t y = 0; 00037 int32_t radius = 120; 00038 int32_t angle = 0; 00039 int8_t button = 0; 00040 int8_t hat = 0; 00041 00042 pc.printf("Hello World!\n\r"); 00043 00044 heartbeat_start(); 00045 00046 while (1) { 00047 // Basic Joystick 00048 throttle = (i >> 8) & 0xFF; // value -127 .. 128 00049 rudder = (i >> 8) & 0xFF; // value -127 .. 128 00050 button = (i >> 8) & 0x0F; // value 0 .. 15, one bit per button 00051 // hat = (i >> 8) & 0x03; // value 0, 1, 2, 3 or 4 for neutral 00052 hat = (i >> 8) & 0x07; // value 0..7 or 8 for neutral 00053 i++; 00054 00055 x = cos((double)angle*3.14/180.0)*radius; // value -127 .. 128 00056 y = sin((double)angle*3.14/180.0)*radius; // value -127 .. 128 00057 angle += 3; 00058 00059 joystick.update(throttle, rudder, x, y, button, hat); 00060 00061 wait(0.001); 00062 } 00063 00064 pc.printf("Bye World!\n\r"); 00065 }
Generated on Thu Jul 14 2022 05:16:23 by
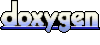