LED Driver, 6 digits @ 8 segm, 8 LEDs, 16 Keys. SPI Interface
Embed:
(wiki syntax)
Show/hide line numbers
Font_7Seg.cpp
00001 /* mbed LED Font Library, for STM STLED316S controller 00002 * Copyright (c) 2016, v01: WH, Initial version 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 #include "Font_7Seg.h" 00023 00024 // ASCII Font definition table for transmission to STLED316S 00025 // 00026 //#define FONT_7S_START 0x20 00027 //#define FONT_7S_END 0x7F 00028 //#define FONT_7S_NR_CHARS (FONT_7_END - FONT_7S_START + 1) 00029 00030 00031 #if (SHOW_ASCII == 1) 00032 //display all ASCII characters 00033 const char FONT_7S[] = { 00034 C7_SPC, //32 0x20, Space 00035 C7_EXC, 00036 C7_QTE, 00037 C7_HSH, 00038 C7_DLR, 00039 C7_PCT, 00040 C7_AMP, 00041 C7_ACC, 00042 C7_LBR, 00043 C7_RBR, 00044 C7_MLT, 00045 C7_PLS, 00046 C7_CMA, 00047 C7_MIN, 00048 C7_DPT, 00049 C7_RS, 00050 C7_0, //48 0x30 00051 C7_1, 00052 C7_2, 00053 C7_3, 00054 C7_4, 00055 C7_5, 00056 C7_6, 00057 C7_7, 00058 C7_8, 00059 C7_9, 00060 C7_COL, //58 0x3A 00061 C7_SCL, 00062 C7_LT, 00063 C7_EQ, 00064 C7_GT, 00065 C7_QM, 00066 C7_AT, //64 0x40 00067 C7_A, //65 0x41, A 00068 C7_B, 00069 C7_C, 00070 C7_D, 00071 C7_E, 00072 C7_F, 00073 C7_G, 00074 C7_H, 00075 C7_I, 00076 C7_J, 00077 C7_K, 00078 C7_L, 00079 C7_M, 00080 C7_N, 00081 C7_O, 00082 C7_P, 00083 C7_Q, 00084 C7_R, 00085 C7_S, 00086 C7_T, 00087 C7_U, 00088 C7_V, 00089 C7_W, 00090 C7_X, 00091 C7_Y, 00092 C7_Z, //90 0x5A, Z 00093 C7_SBL, //91 0x5B 00094 C7_LS, 00095 C7_SBR, 00096 C7_PWR, 00097 C7_UDS, 00098 C7_ACC, 00099 C7_A, //97 0x61, A replacing a 00100 C7_B, 00101 C7_C, 00102 C7_D, 00103 C7_E, 00104 C7_F, 00105 C7_G, 00106 C7_H, 00107 C7_I, 00108 C7_J, 00109 C7_K, 00110 C7_L, 00111 C7_M, 00112 C7_N, 00113 C7_O, 00114 C7_P, 00115 C7_Q, 00116 C7_R, 00117 C7_S, 00118 C7_T, 00119 C7_U, 00120 C7_V, 00121 C7_W, 00122 C7_X, 00123 C7_Y, 00124 C7_Z, // 122 0x7A, Z replacing z 00125 C7_CBL, // 123 0x7B 00126 C7_OR, 00127 C7_CBR, 00128 C7_TLD, 00129 C7_DEL // 127 00130 }; 00131 00132 #else 00133 //display only digits and hex characters 00134 const short FONT_7S[] = { 00135 C7_0, //48 0x30 00136 C7_1, 00137 C7_2, 00138 C7_3, 00139 C7_4, 00140 C7_5, 00141 C7_6, 00142 C7_7, 00143 C7_8, 00144 C7_9, 00145 C7_A, //65 0x41, A 00146 C7_B, 00147 C7_C, 00148 C7_D, 00149 C7_E, 00150 C7_F 00151 };// 127 00152 #endif 00153
Generated on Sat Jul 16 2022 20:44:27 by
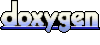