7 Segment LED Displaydriver, I2C interface, SAA1064
Embed:
(wiki syntax)
Show/hide line numbers
SAA1064.h
00001 /* SAA1064 - I2C LED Driver used in multiplex mode (4x 7 Segments and Decimal Point) 00002 * Copyright (c) 2013 Wim Huiskamp 00003 * 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 * 00006 * version 0.2 Initial Release 00007 */ 00008 #ifndef _SAA1064_H 00009 #define _SAA1064_H 00010 00011 /** Driver for SAA1064 I2C 4-Digit 7-Segment LED Driver 00012 * 00013 * @code 00014 * #include "mbed.h" 00015 * #include "SAA1064.h" 00016 * 00017 * // I2C Communication 00018 * I2C i2c_lcd(p28,p27); // SDA, SCL for LPC1768 00019 * //I2C i2c_lcd(P0_10,P0_11); // SDA, SCL for LPC812 00020 * 00021 * SAA1064 LED(&i2c_lcd); // I2C bus, Default SAA1064 Slaveaddress 00022 * 00023 * int main() { 00024 * uint8_t count = 0; 00025 * 00026 * // Display 0, 1, 2, 3 00027 * LED.write(SAA1064_SEGM[0], SAA1064_SEGM[1], SAA1064_SEGM[2], SAA1064_SEGM[3]); 00028 * wait(1); 00029 * 00030 * while(1) { 00031 * wait(0.3); 00032 * count++; 00033 * 00034 * LED.writeInt(-150 + count, 3, false); // Display value, dont suppress leading zero's 00035 * } 00036 * 00037 * } 00038 * @endcode 00039 */ 00040 00041 00042 //Address Defines for SAA1064 00043 #define SAA1064_SA0 0x70 00044 #define SAA1064_SA1 0x72 00045 #define SAA1064_SA2 0x74 00046 #define SAA1064_SA3 0x76 00047 00048 //Register Defines for SAA1064 00049 #define SAA1064_CTRL 0x00 00050 #define SAA1064_DIG1 0x01 00051 #define SAA1064_DIG2 0x02 00052 #define SAA1064_DIG3 0x03 00053 #define SAA1064_DIG4 0x04 00054 00055 //Control Register Defines for SAA1064 00056 //Static display (2 digits) or Multiplexed (4 digits) 00057 #define SAA1064_MPX 0x01 00058 //Digits 1 and 2 On 00059 #define SAA1064_B0 0x02 00060 //Digits 3 and 4 On 00061 #define SAA1064_B1 0x04 00062 //Intensity of display 00063 #define SAA1064_INT0 0x00 00064 #define SAA1064_INT1 0x10 00065 #define SAA1064_INT2 0x20 00066 #define SAA1064_INT3 0x30 00067 #define SAA1064_INT4 0x40 00068 #define SAA1064_INT5 0x50 00069 #define SAA1064_INT6 0x60 00070 #define SAA1064_INT7 0x70 00071 00072 //Default Mode: Multiplex On, All Digits On 00073 #define SAA1064_CTRL_DEF (SAA1064_MPX | SAA1064_B0 | SAA1064_B1) 00074 00075 00076 //Pin Defines for SAA1064 00077 #define D_L0 0x01 00078 #define D_L1 0x02 00079 #define D_L2 0x04 00080 #define D_L3 0x08 00081 #define D_L4 0x10 00082 #define D_L5 0x20 00083 #define D_L6 0x40 00084 #define D_L7 0x80 00085 00086 //Defines for Segments 00087 const uint8_t SAA1064_SEGM[] = {0x3F, //0 00088 0x06, //1 00089 0x5B, //2 00090 0x4F, //3 00091 0x66, //4 00092 0x6D, //5 00093 0x7D, //6 00094 0x07, //7 00095 0x7F, //8 00096 0x6F, //9 00097 0x77, //A 00098 0x7C, //B 00099 0x39, //C 00100 0x5E, //D 00101 0x79, //E 00102 0x71}; //F 00103 00104 #define SAA1064_DP 0x80 //Decimal Point 00105 #define SAA1064_MINUS 0x40 //Minus Sign 00106 #define SAA1064_BLNK 0x00 //Blank Digit 00107 #define SAA1064_ALL 0xFF //All Segments On 00108 00109 00110 00111 00112 /** Create an SAA1064 object connected to the specified I2C bus and deviceAddress 00113 * 00114 */ 00115 class SAA1064 { 00116 public: 00117 /** Create a SAA1064 LED displaydriver object using a specified I2C bus and slaveaddress 00118 * 00119 * @param I2C &i2c the I2C port to connect to 00120 * @param char deviceAddress the address of the SAA1064 00121 */ 00122 SAA1064(I2C *i2c, uint8_t deviceAddress = SAA1064_SA0); 00123 00124 /** Set segment brightness 00125 * 00126 * @param intensity intensity value, valid Range between 0-7, 0 = 0 mA/segment, 1 = 3 mA/segment etc 00127 */ 00128 void setIntensity(uint8_t intensity); 00129 00130 00131 /** Write digits 00132 * 00133 * @param digit1 LED segment pattern for digit1 (MSB) 00134 * @param digit2 LED segment pattern for digit2 00135 * @param digit3 LED segment pattern for digit3 00136 * @param digit4 LED segment pattern for digit4 (LSB) 00137 */ 00138 void write(uint8_t digit1, uint8_t digit2, uint8_t digit3, uint8_t digit4); 00139 00140 /** Write Integer 00141 * 00142 * @param value integer value to display, valid range -999...9999 00143 * @param dp_digit digit where decimal point is set, valid range 1..4 (no DP shown for dp_digit = 0) 00144 * @param leading suppress leading zero (false=show leading zero, true=suppress leading zero) 00145 */ 00146 void writeInt(int value, uint8_t dp_digit=0, bool leading=true); 00147 00148 00149 /** snake: show a short animation 00150 * 00151 * @param count number of times animation is repeated, valid range 0..15 00152 * 00153 */ 00154 void snake(uint8_t count); 00155 00156 /** splash: show a short animation 00157 * 00158 * @param count number of times animation is repeated, valid range 0..15 00159 * 00160 */ 00161 void splash (uint8_t count); 00162 00163 protected: 00164 I2C *_i2c; //I2C bus reference 00165 uint8_t _slaveAddress; //I2C Slave address of device 00166 00167 /** Initialise LED driver 00168 * 00169 */ 00170 void _init(); 00171 }; 00172 00173 #endif
Generated on Tue Jul 12 2022 21:12:25 by
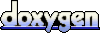