
Test software for PCF8591 and SAA1064
Dependencies: PCF8591 SAA1064 mbed
main.cpp
00001 #include "mbed.h" 00002 #include "SAA1064.h" 00003 #include "PCF8591.h" 00004 00005 #define lpc812 1 00006 #define lpc1768 0 00007 00008 //DigitalOut myled1(LED1); 00009 DigitalOut heartbeatLED(LED1); 00010 00011 //Pin Defines for I2C Bus 00012 00013 #if (lpc1768) 00014 // SDA, SCL for LPC1768 00015 #define D_SDA p28 00016 #define D_SCL p27 00017 #endif 00018 00019 #if (lpc812) 00020 // SDA, SCL for LPC812 00021 #define D_SDA P0_10 00022 #define D_SCL P0_11 00023 #endif 00024 00025 //I2C Bus 00026 I2C i2c_bus(D_SDA, D_SCL); 00027 00028 00029 //SAA1064 LED(&i2c_bus, SAA1064_SA0); 00030 SAA1064 LED(&i2c_bus); 00031 00032 //PCF8591 adc_dac(&i2c_bus, PCF8591_SA0); 00033 PCF8591 adc_dac(&i2c_bus); 00034 00035 PCF8591_AnalogOut anaOut(&i2c_bus); 00036 00037 PCF8591_AnalogIn anaIn(&i2c_bus, PCF8591_ADC1); 00038 00039 00040 //Host PC Baudrate (Virtual Com Port on USB) 00041 #define D_BAUDRATE 9600 00042 //#define D_BAUDRATE 57600 00043 00044 // Host PC Communication channels 00045 #if (lpc1768) 00046 Serial pc(USBTX, USBRX); // tx, rx for mbed LPC800 MAX 00047 #endif 00048 00049 #if (lpc812) 00050 Serial pc(P0_4, P0_0); // tx, rx for LPC812 LPCXpresso 00051 #endif 00052 00053 // Variables for Heartbeat and Status monitoring 00054 Ticker heartbeat; 00055 bool heartbeatflag=false; 00056 00057 // Cycle Timer 00058 //const int maxcount = 1000; 00059 00060 // Local functions 00061 void clear_screen() { 00062 //ANSI Terminal Commands 00063 // pc.printf("\x1B[2J"); 00064 // pc.printf("\x1B[H"); 00065 } 00066 00067 00068 void init_interfaces() { 00069 // Init Host PC communication, default is 9600 00070 //pc.baud(D_BAUDRATE); 00071 00072 // Init I/F hardware 00073 i2c_bus.frequency(100000); 00074 00075 // Init LEDs off 00076 heartbeatLED = 1; 00077 } 00078 00079 // Heartbeat monitor 00080 void pulse() { 00081 heartbeatLED = !heartbeatLED; 00082 } 00083 00084 void heartbeat_start() { 00085 heartbeat.attach(&pulse, 0.5); 00086 } 00087 00088 void heartbeat_stop() { 00089 heartbeat.detach(); 00090 } 00091 00092 00093 int main() { 00094 uint8_t slave_address = 0x40; //PCF8574 00095 char buf[128]; 00096 uint8_t count = 0; 00097 uint8_t analog; 00098 00099 init_interfaces(); 00100 00101 heartbeat_start(); 00102 00103 clear_screen(); 00104 00105 pc.printf("\r\nHello World from LPC812\r\n"); 00106 00107 LED.write(SAA1064_SEGM[0], SAA1064_SEGM[1], SAA1064_SEGM[2], SAA1064_SEGM[3]); 00108 // LED.write(SAA1064_SEGM[4], SAA1064_SEGM[5], SAA1064_SEGM[6], SAA1064_SEGM[7]); 00109 // LED.write(SAA1064_SEGM[8], SAA1064_SEGM[9], SAA1064_SEGM[0x0A], SAA1064_SEGM[0x0B]); 00110 // LED.write(SAA1064_SEGM[0x0C], SAA1064_SEGM[0x0D], SAA1064_SEGM[0x0E], SAA1064_SEGM[0x0F]); 00111 00112 wait(1.0); 00113 00114 LED.snake(1); 00115 wait(1.0); 00116 00117 LED.snake(4); 00118 wait(1.0); 00119 00120 LED.splash(6); 00121 wait(1.0); 00122 00123 00124 //LPC812 I2C BLOCKWRITE BUG: ADC results are unstable, DAC values leaking through... 00125 //The Heartbeat Interrupt also seems to make the problem worse 00126 00127 // Test for PCF8591 00128 while(1) { 00129 float ana; 00130 //pc.putc('*'); 00131 00132 wait_ms(20); 00133 00134 // analog = adc_dac.read(PCF8591_ADC0); // read A/D value for Channel 0 (LDR) 00135 // analog = adc_dac.read(PCF8591_ADC1); // read A/D value for Channel 1 (potmeter) 00136 // pc.printf("%d ", analog); 00137 00138 ana=anaIn; 00139 pc.printf("%2.2f ", ana); 00140 00141 // pc.printf("%2.2f ", anaIn); 00142 00143 anaOut = ana; 00144 } 00145 00146 00147 // Test for SAA1064 and PCF8591 00148 while(0) { 00149 00150 //pc.printf("*"); 00151 pc.putc('*'); 00152 wait_ms(200); 00153 00154 buf[0] = count; 00155 i2c_bus.write(slave_address, buf, 1); 00156 count++; 00157 00158 // LED.writeInt(-150 + count, 3, false); 00159 LED.writeInt(-150 + count, 3); //suppress leading zero 00160 00161 adc_dac.write(count); // write D/A value 00162 00163 // analog = adc_dac.read(PCF8591_ADC0); // read A/D value for Channel 0 (LDR) 00164 analog = adc_dac.read(PCF8591_ADC1); // read A/D value for Channel 1 (potmeter) 00165 pc.printf("%d ", analog); 00166 00167 } 00168 00169 // pc.printf("Bye World!\n\r"); 00170 }
Generated on Thu Jul 14 2022 01:51:08 by
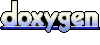