
Adafruit GPS library sample with Xadow GPS and Xadow Oled
Dependencies: MBed_Adafruit-GPS-Library SSD1308_128x64_I2C USBDevice mbed
Fork of AVC_gps by
main.cpp
00001 #include "mbed.h" 00002 #include "pinmap.h" 00003 #include "SSD1308.h" 00004 #include "MBed_Adafruit_GPS.h" 00005 00006 Serial gps(P0_19,P0_18); 00007 00008 #define DEBUG 00009 00010 #ifdef DEBUG 00011 #include "USBSerial.h" // To use USB virtual serial, a driver is needed, check http://mbed.org/handbook/USBSerial 00012 #define LOG(args...) pc.printf(args) 00013 USBSerial pc; 00014 #else 00015 #define LOG(args...) 00016 #endif 00017 00018 #define HARD_SPI 1 00019 #define I2C_FREQ 100000 00020 00021 I2C i2c(I2C_SDA, I2C_SCL); 00022 //Use Xadow OLED for display 00023 SSD1308 oled = SSD1308(i2c, SSD1308_SA0); 00024 00025 //GPS Data 00026 00027 00028 int main() 00029 { 00030 Adafruit_GPS myGPS(&gps); 00031 char c; //when read via Adafruit_GPS::read(), the class returns single character stored here 00032 Timer refresh_Timer; //sets up a timer for use in loop; how often do we print GPS info? 00033 const int refresh_Time = 1000; //refresh time in ms 00034 00035 myGPS.begin(9600); 00036 oled.clearDisplay(); 00037 oled.writeString(0,0,"GPS Test"); 00038 oled.writeString(1,0,"GPS Start"); 00039 00040 wait(1); 00041 00042 refresh_Timer.start(); //starts the clock on the timer 00043 00044 while (true) { 00045 c = myGPS.read(); //queries the GPS 00046 00047 if (c) { 00048 pc.printf("%c", c); //this line will echo the GPS data if not paused 00049 } 00050 00051 //check if we recieved a new message from GPS, if so, attempt to parse it, 00052 if ( myGPS.newNMEAreceived() ) { 00053 if ( !myGPS.parse(myGPS.lastNMEA()) ) { 00054 continue; 00055 } 00056 } 00057 00058 00059 00060 //check if enough time has passed to warrant printing GPS info to screen 00061 //note if refresh_Time is too low or pc.baud is too low, GPS data may be lost during printing 00062 if (refresh_Timer.read_ms() >= refresh_Time) { 00063 refresh_Timer.reset(); 00064 pc.printf("Time: %d:%d:%d.%u\n", myGPS.hour, myGPS.minute, myGPS.seconds, myGPS.milliseconds); 00065 pc.printf("Date: %d/%d/20%d\n", myGPS.day, myGPS.month, myGPS.year); 00066 pc.printf("Fix: %d\n", (int) myGPS.fix); 00067 pc.printf("Quality: %d\n", (int) myGPS.fixquality); 00068 if (myGPS.fix) { 00069 pc.printf("Location: %5.2f%c, %5.2f%c\n", myGPS.latitude, myGPS.lat, myGPS.longitude, myGPS.lon); 00070 pc.printf("Speed: %5.2f knots\n", myGPS.speed); 00071 pc.printf("Angle: %5.2f\n", myGPS.angle); 00072 pc.printf("Altitude: %5.2f\n", myGPS.altitude); 00073 pc.printf("Satellites: %d\n", myGPS.satellites); 00074 } 00075 00076 if (myGPS.fix) { 00077 char oled_str[20]; 00078 oled.writeString(1,0,"GPS Lock "); 00079 sprintf(oled_str,"LAT:%f",myGPS.latitude); 00080 oled.writeString(2,0,oled_str); 00081 sprintf(oled_str,"LON:%f",myGPS.longitude); 00082 oled.writeString(3,0,oled_str); 00083 sprintf(oled_str,"ALT:%f",myGPS.altitude); 00084 oled.writeString(4,0,oled_str); 00085 } else { 00086 oled.writeString(1,0,"GPS Lost "); 00087 } 00088 } 00089 } 00090 }
Generated on Mon Jul 18 2022 09:09:06 by
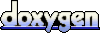