Modified to run on Renesas GR Peach
Fork of mbed-rpc by
Embed:
(wiki syntax)
Show/hide line numbers
Arguments.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef ARGUMENTS_H 00017 #define ARGUMENTS_H 00018 00019 #include "platform.h" 00020 00021 namespace mbed { 00022 00023 #define RPC_MAX_STRING 128 00024 #define RPC_MAX_ARGS 16 00025 00026 class Arguments { 00027 public: 00028 Arguments(const char* rqs); 00029 00030 template<typename Arg> 00031 Arg getArg(void); 00032 00033 char *obj_name; 00034 char *method_name; 00035 00036 int argc; 00037 char* argv[RPC_MAX_ARGS]; 00038 00039 private: 00040 // This copy can be removed if we can assume the request string is 00041 // persistent and writable for the duration of the call 00042 char request[RPC_MAX_STRING]; 00043 int index; 00044 char* search_arg(char **arg, char *p, char next_sep); 00045 }; 00046 00047 class Reply { 00048 public: 00049 Reply(char* r); 00050 00051 template<typename Data> 00052 void putData(Data d); 00053 00054 private: 00055 void separator(void); 00056 bool first; 00057 char* reply; 00058 }; 00059 00060 00061 } // Namespace mbed 00062 00063 #endif
Generated on Sat Jul 16 2022 20:13:06 by
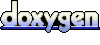