Graphics Drawing Interface EZLCD4 display using serial
Embed:
(wiki syntax)
Show/hide line numbers
gdiezl4.h
00001 /* mbed R/C Servo Library 00002 * Copyright (c) 2007-2010 sford, cstyles 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 // ensure only one copy 00024 #ifndef _GDIEZL4_H 00025 #define _GDIEZL4_H 00026 00027 // include files 00028 #include "mbed.h" 00029 #include "types.h" 00030 #include "MODSERIAL.h" 00031 #include "FPointer.h" 00032 00033 // define the RGB macro 00034 #define RGB( R, G, B ) (((( R >> 3 ) & 0x1F ) << 11 ) | ((( G >> 2 ) & 0x3F ) << 5 ) | (( B >> 3 ) & 0x1F )) 00035 00036 // define the max X/Y in landscaple mode 00037 #define MAX_X_SIZE 319 00038 #define MAX_Y_SIZE 233 00039 00040 // define some standard colcors 00041 #define GDI_CLR_BLK RGB( 0, 0, 0 ) 00042 #define GDI_CLR_WHT RGB( 255, 255, 255 ) 00043 #define GDI_CLR_BLU RGB( 0, 0, 255 ) 00044 #define GDI_CLR_RED RGB( 255, 0, 0 ) 00045 #define GDI_CLR_GRN RGB( 0, 255, 0 ) 00046 00047 // define the no button icon valu 00048 #define GDI_BTN_NOICON 255 00049 00050 // define the button up/down masks 00051 #define GDI_BTN_DNMSK 0x40 00052 #define GDI_BTN_UPMSK 0x80 00053 #define GDI_BTN_VLMSK 0x3F 00054 00055 // define the pong value 00056 #define GDI_PNG_VALUE 0x38 00057 00058 // class definition 00059 class GdiEzL4 00060 { 00061 public: 00062 // construction/destruction 00063 /** Graphics Drawing Interface (EZLCD4 ) construction 00064 * 00065 * @param pinTx = transmit pin 00066 * @param pinRx = receive pin 00067 */ 00068 GdiEzL4( PinName pinTx, PinName pinRx ); 00069 ~GdiEzL4( ); 00070 00071 // attributes 00072 public: 00073 // enumerate the text direction- 00074 enum GDITXTDIR { 00075 GDI_TXT_NORTH = 0, // text direction north 00076 GDI_TXT_EAST, // text direction east 00077 GDI_TXT_SOUTH, // text direction south 00078 GDI_TXT_WEST // text direction west 00079 }; 00080 00081 // enumerate the button state 00082 enum GDIBTNSTATE { 00083 GDI_BTN_UP = 1, // button up 00084 GDI_BTN_DN, // button down 00085 GDI_BTN_DSB, // button disabled 00086 GDI_BTN_NVS // button non-visible 00087 }; 00088 00089 // define the button parameters 00090 struct GDIBTNDEF { 00091 GDIBTNSTATE eInitalState; // initial state 00092 U8 nUpIcon; // up icon index 00093 U8 nDnIcon; // down icon index 00094 U8 nDsIcon; // disabled icon index 00095 U16 wUpLfX; // upper left X 00096 U8 nUpLfY; // upper left Y 00097 U8 nTouchWidth; // touch width 00098 U8 nTouchHeight; // touch height 00099 }; 00100 00101 private: 00102 MODSERIAL m_serDisp; 00103 FPointer m_pvFuncCallBack; 00104 00105 // local functions 00106 void LocalCallback( void ); 00107 00108 // enumerate the LCD commands 00109 enum COMMANDS { 00110 EZ_CLS = 0x21, // 0x21 - clear screen 00111 EZ_LON, // 0x22 - backlight on 00112 EZ_LOF, // 0x23 - backlight off 00113 EZ_PLT = 0x26, // 0x26 - plot a pixel 00114 EZ_PIC = 0x2A, // 0x2A - draw a picture 00115 EZ_FNT, // 0x2B - select the font 00116 EZ_CHR, // 0x2C - draw a character 00117 EZ_STR, // 0x2D - draw a string 00118 EZ_SPS = 0x35, // 0x35 - store position 00119 EZ_RPS, // 0x36 - restore position 00120 EZ_CHB = 0x3C, // 0x3C - draw character on the background 00121 EZ_STB, // 0x3D - draw a string on the background 00122 EZ_VLN = 0x41, // 0x41 - draw a vertical line 00123 EZ_ICF = 0x58, // 0x58 - draw an icon from serial flash 00124 EZ_STY = 0x5F, // 0x5F - set y position 00125 EZ_TXN = 0x60, // 0x60 - set text direction to north 00126 EZ_TXE, // 0x61 - set text direction to east 00127 EZ_TXS, // 0x62 - set text direction to south 00128 EZ_TXW, // 0x63 - set text direction to west 00129 EZ_STX = 0x6E, // 0x6E - set X position 00130 EZ_BKL = 0x80, // 0x80 - brightness 00131 EZ_PNG = 0x83, // 0x83 - ping the unit 00132 EZ_FGC, // 0x84 - set foreground color 00133 EZ_SXY, // 0x85 - set X/y position 00134 EZ_PXY = 0x87, // 0x87 - plot a pixel at x,y 00135 EZ_LIN, // 0x88 - draw a line 00136 EZ_CIR, // 0x89 - draw a circle 00137 EZ_ARC = 0x8F, // 0x8F - draw an arc 00138 EZ_PIE, // 0x90 - draw a pie 00139 EZ_BGC = 0x94, // 0x94 - set background color 00140 EZ_CRF = 0x99, // 0x99 - draw a filled circle 00141 EZ_BMP = 0x9E, // 0x9E - draw a bitmap 00142 EZ_HLN = 0xA0, // 0xA0 - draw a horizontal line 00143 EZ_BOX = 0xA2, // 0xA2 - draw a box 00144 EZ_BXF, // 0xA3 - draw a filled box 00145 EZ_BTD = 0xB0, // 0xB0 - define a touch button 00146 EZ_BTS, // 0xB1 - changes a button state 00147 EZ_BTP, // 0xB2 - sets the button protocol 00148 EZ_BUP, // 0xB3 - all buttons up 00149 EZ_BDL, // 0xB4 - delete all buttons 00150 EZ_BOF = 0xD0, // 0xD0 - buzzer off 00151 EZ_BON, // 0xD1 - buzzer on 00152 EZ_BEE // 0xD2 - beep the buzzer for some time 00153 }; 00154 00155 public: 00156 // implementation 00157 /** backlight ontrol 00158 * 00159 * @param bOffOn = state of the backlight 00160 */ 00161 void BacklightCtl( BOOL bOffOn ); 00162 00163 /** clear the screen 00164 * 00165 * @param wColor = background color 00166 */ 00167 void ClearScreen( U16 wColor ); 00168 00169 /** Draw a rectangle 00170 * 00171 * @param wColor = color of the rectangle 00172 * @param wSx = starting X location ( top left ) 00173 * @param nSy = starting Y location ( top left ) 00174 * @param wWidth = width of the rectangle 00175 * @param nHeight = height of the rectangle 00176 * @param bFill = TRUE if rectangle is filled 00177 */ 00178 void DrawRect( U16 wColor, U16 wSx, U8 nSy, U16 wWidth, U8 nHeight, BOOL fFill ); 00179 00180 /** Draw a line 00181 * 00182 * @param wColor = color of the line 00183 * @param wSx = starting X location ( top left ) 00184 * @param nSy = starting Y location ( top left ) 00185 * @param wEx = ending X location ( bottom right ) 00186 * @param nEy = ending Y location ( bottom right ) 00187 */ 00188 void DrawLine( U16 wColor, U16 wSx, U8 nSy, U16 wEx, U8 nEy ); 00189 00190 /** Draw an Icon 00191 * 00192 * @param wSx = starting X location ( top left ) 00193 * @param nSy = starting Y location ( top left ) 00194 * @param nIcon = icon index 00195 */ 00196 void DrawIcon( U16 wSx, U8 nSy, U8 nIcon ); 00197 00198 /** Draw a character 00199 * 00200 * @param wColor = color of the character 00201 * @param nFont = font index 00202 * @param wSx = starting X location ( top left ) 00203 * @param nSy = starting Y location ( top left ) 00204 * @param bBackground = display as inverted background 00205 * @param eDir = text direction 00206 * @param cChar = character to draw 00207 */ 00208 void DrawChar( U16 wColor, U8 nFont, U16 wSx, U8 nSy, BOOL bBackground, GDITXTDIR eDir, C8 cChar ); 00209 00210 /** draw a string 00211 * 00212 * @param wColor = color of the character 00213 * @param nFont = font index 00214 * @param wSx = starting X location ( top left ) 00215 * @param nSy = starting Y location ( top left ) 00216 * @param bBackground = display as inverted background 00217 * @param eDir = text direction 00218 * @param pszMsg = pointer to the message 00219 */ 00220 void DrawString( U16 wFgClr, U16 wBgClr, U8 nFont, U16 wSx, U8 nSy, BOOL bBackground, GDITXTDIR eDir, PC8 pszMsg ); 00221 00222 /** backlight ontrol 00223 * 00224 * @param nBtnIdx = button index 00225 * @param ptBugDef -> pointer to the button definition structure 00226 */ 00227 void DrawButton( U8 nBtnIdx, GDIBTNDEF* ptButDef ); 00228 00229 /** backlight ontrol 00230 * 00231 * @param bOffOn = state of the backlight 00232 */ 00233 void RemoveAllButtons( void ); 00234 00235 /** backlight ontrol 00236 * 00237 * @param bOffOn = state of the backlight 00238 */ 00239 void PingDisplay( void ); 00240 00241 /** backlight ontrol 00242 * 00243 * @param bOffOn = state of the backlight 00244 */ 00245 void attach( U32 ( *function )( U32 ) = 0) { m_pvFuncCallBack.attach( function ); } 00246 00247 /** backlight ontrol 00248 * 00249 * @param bOffOn = state of the backlight 00250 */ 00251 template<class T> 00252 void attach( T* item, U32 ( T::*method )( U32 )) { m_pvFuncCallBack.attach( item, method ); } 00253 }; 00254 00255 00256 #endif 00257
Generated on Sat Jul 30 2022 03:09:17 by
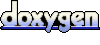