Generic SmartRest library
Dependents: SmartRestUnitTest MbedSmartRest MbedSmartRestStreaming
ComposedRecord.cpp
00001 /* 00002 * ComposedRecord.cpp 00003 * 00004 * Created on: Nov 1, 2013 00005 * * Authors: Vincent Wochnik <v.wochnik@gmail.com> 00006 * 00007 * Copyright (c) 2013 Cumulocity GmbH 00008 * 00009 * Permission is hereby granted, free of charge, to any person obtaining 00010 * a copy of this software and associated documentation files (the 00011 * "Software"), to deal in the Software without restriction, including 00012 * without limitation the rights to use, copy, modify, merge, publish, 00013 * distribute, sublicense, and/or sell copies of the Software, and to 00014 * permit persons to whom the Software is furnished to do so, subject to 00015 * the following conditions: 00016 * 00017 * The above copyright notice and this permission notice shall be 00018 * included in all copies or substantial portions of the Software. 00019 * 00020 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00021 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00022 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00023 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE 00024 * LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION 00025 * OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION 00026 * WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00027 */ 00028 00029 #include "ComposedRecord.h" 00030 #include "NullValue.h" 00031 #include <stdlib.h> 00032 #include <string.h> 00033 00034 ComposedRecord::ComposedRecord(bool copy) 00035 { 00036 _alloc = copy; 00037 #ifndef COMPOSED_FIXED_SIZE 00038 _capacity = COMPOSED_INITIAL_CAPACITY; 00039 _values = (const Value**)malloc(_capacity*sizeof(Value*)); 00040 #endif 00041 _count = 0; 00042 } 00043 00044 ComposedRecord::~ComposedRecord() 00045 { 00046 if (_alloc) { 00047 for (size_t n = 0; n < _count; n++) 00048 delete _values[n]; 00049 } 00050 #ifndef COMPOSED_FIXED_SIZE 00051 free(_values); 00052 #endif 00053 } 00054 00055 ComposedRecord& ComposedRecord::add(const Value& value) 00056 { 00057 #ifndef COMPOSED_FIXED_SIZE 00058 if (_capacity == _count) { 00059 size_t capacity = _capacity + COMPOSED_MEMORY_INCREMENT; 00060 const Value **values = (const Value**)realloc(_values, 00061 capacity*sizeof(Value*)); 00062 if (values == NULL) 00063 return *this; 00064 _values = values; 00065 _capacity = capacity; 00066 } 00067 #else 00068 if (_count == COMPOSED_FIXED_SIZE) 00069 return *this; 00070 #endif 00071 00072 if (_alloc) { 00073 Value *copy = value.copy(); 00074 if (copy == NULL) 00075 return *this; 00076 _values[_count++] = copy; 00077 } else { 00078 _values[_count++] = &value; 00079 } 00080 return *this; 00081 } 00082 00083 void ComposedRecord::clear() 00084 { 00085 _count = 0; 00086 #ifndef COMPOSED_FIXED_SIZE 00087 if (_capacity > COMPOSED_INITIAL_CAPACITY) { 00088 Value** values = (Value**)realloc(_values, 00089 COMPOSED_INITIAL_CAPACITY*sizeof(Value*)); 00090 if (values == NULL) 00091 return; 00092 _capacity = COMPOSED_INITIAL_CAPACITY; 00093 } 00094 #endif 00095 } 00096 00097 size_t ComposedRecord::values() const 00098 { 00099 return _count; 00100 } 00101 00102 const Value& ComposedRecord::value(size_t index) const 00103 { 00104 if (index >= _count) 00105 return aNullValue; 00106 return *_values[index]; 00107 } 00108 00109 DataGenerator* ComposedRecord::copy() const 00110 { 00111 ComposedRecord *copy = new ComposedRecord(true); 00112 for (size_t n = 0; n < _count; n++) 00113 copy->add(*_values[n]); 00114 return copy; 00115 } 00116
Generated on Wed Jul 13 2022 09:53:06 by
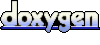