Generic SmartRest library
Dependents: SmartRestUnitTest MbedSmartRest MbedSmartRestStreaming
Aggregator.h
00001 /* 00002 * Aggregator.h 00003 * 00004 * Created on: Nov 1, 2013 00005 * * Authors: Vincent Wochnik <v.wochnik@gmail.com> 00006 * 00007 * Copyright (c) 2013 Cumulocity GmbH 00008 * 00009 * Permission is hereby granted, free of charge, to any person obtaining 00010 * a copy of this software and associated documentation files (the 00011 * "Software"), to deal in the Software without restriction, including 00012 * without limitation the rights to use, copy, modify, merge, publish, 00013 * distribute, sublicense, and/or sell copies of the Software, and to 00014 * permit persons to whom the Software is furnished to do so, subject to 00015 * the following conditions: 00016 * 00017 * The above copyright notice and this permission notice shall be 00018 * included in all copies or substantial portions of the Software. 00019 * 00020 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00021 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00022 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00023 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE 00024 * LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION 00025 * OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION 00026 * WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00027 */ 00028 00029 #ifndef AGGREGATOR_H 00030 #define AGGREGATOR_H 00031 00032 #ifdef HAVE_CONFIG_H 00033 #include <config.h> 00034 #endif 00035 00036 #include <stddef.h> 00037 #include "DataGenerator.h" 00038 00039 #ifndef AGGREGATOR_INITIAL_CAPACITY 00040 #define AGGREGATOR_INITIAL_CAPACITY 50 00041 #endif 00042 #ifndef AGGREGATOR_MEMORY_INCREMENT 00043 #define AGGREGATOR_MEMORY_INCREMENT 25 00044 #endif 00045 //#define AGGREGATOR_FIXED_SIZE 100 00046 00047 /** 00048 * An aggregator of data generators. This class can aggregate instances of 00049 * itself. 00050 * If the aggregator is set to copying all added data generators using 00051 * the copy() method, all objects are being properly deallocated on 00052 * destruction. 00053 */ 00054 class Aggregator : public DataGenerator 00055 { 00056 public: 00057 #ifndef AGGREGATOR_FIXED_SIZE 00058 /** 00059 * Creates a new Aggregator instance. 00060 * @param capacity the initial capacity of the instance 00061 * @param growing specifies the capability of this instance to grow 00062 * @param copy specifies whether all added data generators shall be 00063 * copied using the copy() method 00064 */ 00065 Aggregator(size_t=AGGREGATOR_INITIAL_CAPACITY, bool=true, bool=false); 00066 #else 00067 /** 00068 * Creates a new Aggregator instance. 00069 * @param copy specifies whether all added data generators shall be 00070 * copied using the copy() method 00071 */ 00072 Aggregator(bool=false); 00073 #endif 00074 ~Aggregator(); 00075 00076 /** 00077 * Adds a data generator to the aggregator. 00078 * @param generator the data generator to add 00079 * @return true if added, false otherwise. 00080 */ 00081 bool add(const DataGenerator&); 00082 00083 /** 00084 * Clears the aggregator. The capacity will shrink to it's initial 00085 * size. 00086 */ 00087 void clear(); 00088 00089 /** 00090 * Returns the number of data generators aggregated by this instance. 00091 * @return the number of data generators aggregated 00092 */ 00093 size_t length(); 00094 00095 /** 00096 * Returns whether the aggregator is full. If growing, this will 00097 * always return false. 00098 * @return whether the aggregator is full 00099 */ 00100 bool full(); 00101 00102 /** 00103 * Returns the capacity of the aggregator. This will always return zero 00104 * if the aggregator is growing. 00105 */ 00106 size_t capacity(); 00107 00108 size_t writeTo(AbstractDataSink&) const; 00109 size_t writtenLength() const; 00110 DataGenerator* copy() const; 00111 00112 private: 00113 #ifdef AGGREGATOR_FIXED_SIZE 00114 const DataGenerator *_list[AGGREGATOR_FIXED_SIZE]; 00115 #else 00116 const DataGenerator **_list; 00117 #endif 00118 size_t _length; 00119 bool _alloc; 00120 #ifndef AGGREGATOR_FIXED_SIZE 00121 size_t _capacity, _initial; 00122 bool _growing; 00123 #endif 00124 }; 00125 00126 #endif
Generated on Wed Jul 13 2022 09:53:06 by
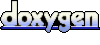