
smart rest test program
Dependencies: C027 MbedSmartRest UbloxUSBModem mbed
main.cpp
00001 #include "mbed.h" 00002 #include "C027.h" 00003 #include "UbloxUSBGSMModem.h" 00004 #include "UbloxUSBCDMAModem.h" 00005 00006 #include "HTTPClient.h" 00007 00008 C027 c027; 00009 00010 void test(void const*) 00011 { 00012 c027.mdmPower(true); 00013 UbloxUSBGSMModem modem; // for LISA-C use the UbloxUSBCDMAModem instead 00014 HTTPClient http; 00015 char str[512]; 00016 00017 int ret = modem.connect("internet"); // eventaully set another apn here 00018 if(ret) 00019 { 00020 printf("Could not connect %d\n", ret); 00021 return; 00022 } 00023 00024 //GET data 00025 printf("Trying to fetch page...\n"); 00026 ret = http.get("http://mbed.org/media/uploads/donatien/hello.txt", str, 128); 00027 if (!ret) { 00028 printf("Page fetched successfully - read %d characters\n", strlen(str)); 00029 printf("Result: %s\n", str); 00030 } else { 00031 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00032 } 00033 00034 //POST data 00035 HTTPMap map; 00036 HTTPText text(str, 512); 00037 map.put("Hello", "World"); 00038 map.put("test", "1234"); 00039 printf("Trying to post data...\n"); 00040 ret = http.post("http://httpbin.org/post", map, &text); 00041 if (!ret) { 00042 printf("Executed POST successfully - read %d characters\n", strlen(str)); 00043 printf("Result: %s\n", str); 00044 } else { 00045 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00046 } 00047 00048 modem.disconnect(); 00049 c027.mdmPower(false); 00050 00051 while(1) { 00052 } 00053 } 00054 00055 00056 int main() 00057 { 00058 Thread testTask(test, NULL, osPriorityNormal, 1024 * 4); 00059 DigitalOut led(LED); // on rev A you should reasign the signal to A0 00060 while(1) { 00061 led=!led; 00062 Thread::wait(1000); 00063 } 00064 00065 return 0; 00066 }
Generated on Thu Aug 4 2022 21:59:56 by
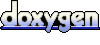