Wrapped version of the ILI9341 graphics library with some MBED tweaks
Dependencies: SDFileSystem SPI_TFT_ILI9341 TFT_fonts
tft.cpp
00001 #include "mbed.h" 00002 #include "tft.h" 00003 00004 #include "Arial12x12.h" 00005 #include "Arial24x23.h" 00006 #include "Arial28x28.h" 00007 #include "font_big.h" 00008 #include "Tahoma22x26.h" 00009 00010 #define PIN_MOSI D11 00011 #define PIN_MISO D12 00012 #define PIN_SCLK D13 00013 #define PIN_DC D4 00014 #define PIN_RST D3 00015 #define PIN_CS D2 00016 00017 #define PIN_SD_MOSI PC_12 00018 #define PIN_SD_SCLK PC_10 00019 #define PIN_SD_MISO PC_11 00020 #define PIN_SD_CS PD_2 00021 00022 extern unsigned char p1[]; // the mbed logo graphic 00023 00024 TFT::TFT() 00025 : SPI_TFT_ILI9341(PIN_MOSI, PIN_MISO, PIN_SCLK, PIN_CS, PIN_RST, PIN_DC,"TFT") 00026 , m_cSD(PIN_SD_MOSI, PIN_SD_MISO, PIN_SD_SCLK, PIN_SD_CS, "sd") 00027 { 00028 init(); 00029 } 00030 00031 void TFT::init() 00032 { 00033 set_orientation(3); 00034 background(Blue); // set background to black 00035 foreground(White); // set chars to white 00036 cls(); // clear the screen 00037 00038 set_font((unsigned char*)Tahoma22x26); 00039 locate(0,0); 00040 } 00041 00042 void TFT::test() 00043 { 00044 //first show the 4 directions 00045 set_orientation(0); 00046 background(Black); 00047 cls(); 00048 00049 set_font((unsigned char*) Arial12x12); 00050 locate(0,0); 00051 printf(" Hello Mbed 0"); 00052 set_orientation(1); 00053 locate(0,0); 00054 printf(" Hello Mbed 1"); 00055 set_orientation(2); 00056 locate(0,0); 00057 printf(" Hello Mbed 2"); 00058 set_orientation(3); 00059 locate(0,0); 00060 printf(" Hello Mbed 3"); 00061 set_orientation(3); 00062 set_font((unsigned char*) Arial24x23); 00063 locate(50,100); 00064 printf("TFT orientation"); 00065 00066 wait(5); // wait two seconds 00067 00068 // draw some graphics 00069 cls(); 00070 set_font((unsigned char*) Arial24x23); 00071 locate(100,100); 00072 printf("Graphic"); 00073 00074 line(0,0,100,0,Green); 00075 line(0,0,0,200,Green); 00076 line(0,0,100,200,Green); 00077 00078 rect(100,50,150,100,Red); 00079 fillrect(180,25,220,70,Blue); 00080 00081 circle(80,150,33,White); 00082 fillcircle(160,190,20,Yellow); 00083 00084 double s; 00085 00086 for (int i=0; i<320; i++) { 00087 s =20 * sin((long double) i / 10 ); 00088 pixel(i,100 + (int)s ,Red); 00089 } 00090 00091 00092 wait(5); // wait two seconds 00093 00094 // bigger text 00095 foreground(White); 00096 background(Blue); 00097 cls(); 00098 set_font((unsigned char*) Arial24x23); 00099 locate(0,0); 00100 printf("Different Fonts :"); 00101 00102 set_font((unsigned char*) Neu42x35); 00103 locate(10,30); 00104 printf("Hello Mbed 1"); 00105 set_font((unsigned char*) Arial24x23); 00106 locate(20,80); 00107 printf("Hello Mbed 2"); 00108 set_font((unsigned char*) Arial12x12); 00109 locate(35,120); 00110 printf("Hello Mbed 3"); 00111 wait(5); 00112 00113 background(Black); 00114 cls(); 00115 locate(10,10); 00116 printf("Graphic from Flash"); 00117 00118 // mbed logo 00119 // defined in graphics.c 00120 //__align(4) 00121 //unsigned char p1[18920] = { 00122 //0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, .... 00123 // 00124 Bitmap(90,90,172,55,p1); 00125 00126 wait(5); 00127 cls(); 00128 00129 // to compare the speed of the internal file system and a SD-card 00130 locate(10,10); 00131 printf("Graphic from internal File System"); 00132 locate(10,20); 00133 printf("open test.bmp"); 00134 int err = BMP_16(1,50,"/local/test.bmp"); 00135 if (err != 1) printf(" - Err: %d",err); 00136 00137 locate(10,110); 00138 printf("Graphic from external SD-card"); 00139 locate(10,120); 00140 BMP_16(1,140,"/sd/test.bmp"); 00141 if (err != 1) printf(" - Err: %d",err); 00142 00143 wait(5); 00144 init(); 00145 }
Generated on Wed Jul 20 2022 00:31:42 by
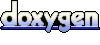