
Program that uses the EOF sequence of Vizualeyez motion tracker to trigger an output.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut gpo(PTD7); 00004 DigitalOut led(LED_RED); 00005 00006 InterruptIn trig(PTD1); 00007 Timer trigtimer; 00008 Timeout output_timeout; 00009 Timeout input_timeout; 00010 00011 00012 typedef enum {TRIG_DETECT_IDLE, TRIG_LOW, TRIG_FIRST_HIGH} trig_enum_t; 00013 typedef enum {TRIG_EVENT_RISING, TRIG_EVENT_FALLING, TRIG_EVENT_TIMEOUT} trig_event_t; 00014 00015 trig_enum_t trigger = TRIG_DETECT_IDLE; 00016 00017 //Function declarations 00018 void input_timeout_handler(void); 00019 void get_new_state(trig_enum_t * trig, trig_event_t event); 00020 void set_output(void); 00021 void trig_rise_event(void); 00022 void trig_fall_event(void); 00023 00024 00025 00026 void input_timeout_handler(void) 00027 { 00028 get_new_state(&trigger, TRIG_EVENT_TIMEOUT); 00029 } 00030 00031 void get_new_state(trig_enum_t * trig, trig_event_t event) 00032 { 00033 const int jitter = 8; 00034 switch(*trig) 00035 { 00036 case TRIG_DETECT_IDLE: 00037 { 00038 if(event == TRIG_EVENT_FALLING) 00039 { 00040 *trig = TRIG_LOW; 00041 trigtimer.reset(); 00042 } 00043 else // should not happen 00044 *trig = TRIG_DETECT_IDLE; 00045 break; 00046 } 00047 case TRIG_LOW: 00048 { 00049 if(event == TRIG_EVENT_RISING) 00050 { 00051 if(trigtimer.read_us() > (30 - jitter) && trigtimer.read_us() < (30+jitter) ) //if first low period detected 00052 { 00053 *trig = TRIG_FIRST_HIGH; 00054 input_timeout.attach_us(input_timeout_handler, 60); 00055 } 00056 else //pulse too short or too long 00057 *trig = TRIG_DETECT_IDLE; 00058 } 00059 00060 else // should not happen 00061 *trig = TRIG_DETECT_IDLE; 00062 break; 00063 } 00064 case TRIG_FIRST_HIGH: 00065 { 00066 led=!led; 00067 if(event == TRIG_EVENT_TIMEOUT) 00068 { 00069 gpo.write(0); 00070 output_timeout.attach_us(set_output, 1000); 00071 *trig = TRIG_DETECT_IDLE; 00072 } 00073 00074 else // should not happen, maybe falling edge due to TOP684 00075 { 00076 *trig = TRIG_DETECT_IDLE; 00077 input_timeout.detach(); 00078 } 00079 break; 00080 } 00081 default: 00082 break; 00083 } 00084 00085 } 00086 00087 00088 void set_output(void) 00089 { 00090 gpo.write(1); 00091 } 00092 00093 00094 void trig_rise_event(void) 00095 { 00096 get_new_state(&trigger, TRIG_EVENT_RISING); 00097 } 00098 00099 00100 void trig_fall_event(void) 00101 { 00102 get_new_state(&trigger, TRIG_EVENT_FALLING); 00103 00104 } 00105 00106 int main() 00107 { 00108 trigtimer.start(); 00109 trig.rise(trig_rise_event); 00110 trig.fall(trig_fall_event); 00111 while (true) { 00112 //gpo = !gpo; // toggle pin 00113 //led = !led; // toggle led 00114 wait(0.2f); 00115 } 00116 }
Generated on Thu Jul 28 2022 09:09:44 by
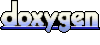