
EtherCAT slave that reads 3 Xsens IMU's connected to a Xbus Master
Dependencies: MODSERIAL mbed KL25Z_ClockControl
Fork of EtherCAT by
esc_hw.cpp
00001 /* 00002 * SOES Simple Open EtherCAT Slave 00003 * 00004 * File : esc_hw.c 00005 * Version : 0.9.2 00006 * Date : 22-02-2010 00007 * Copyright (C) 2007-2010 Arthur Ketels 00008 * 00009 * SOES is free software; you can redistribute it and/or modify it under 00010 * the terms of the GNU General Public License version 2 as published by the Free 00011 * Software Foundation. 00012 * 00013 * SOES is distributed in the hope that it will be useful, but WITHOUT ANY 00014 * WARRANTY; without even the implied warranty of MERCHANTABILITY or 00015 * FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License 00016 * for more details. 00017 * 00018 * As a special exception, if other files instantiate templates or use macros 00019 * or inline functions from this file, or you compile this file and link it 00020 * with other works to produce a work based on this file, this file does not 00021 * by itself cause the resulting work to be covered by the GNU General Public 00022 * License. However the source code for this file must still be made available 00023 * in accordance with section (3) of the GNU General Public License. 00024 * 00025 * This exception does not invalidate any other reasons why a work based on 00026 * this file might be covered by the GNU General Public License. 00027 * 00028 * The EtherCAT Technology, the trade name and logo "EtherCAT" are the intellectual 00029 * property of, and protected by Beckhoff Automation GmbH. 00030 */ 00031 #include "cpuinit.h" 00032 #include "utypes.h" 00033 00034 #define ESC_CMD_READ 0x02 00035 #define ESC_CMD_READWS 0x03 00036 #define ESC_CMD_WRITE 0x04 00037 #define ESC_CMD_NOP 0x00 00038 #define ESC_TERM 0xff 00039 #define ESC_NEXT 0x00 00040 00041 #ifndef WSREAD 00042 00043 /** \fn uint8 ESC_read(uint16 address, void *buf, uint8 len, void *tALevent) 00044 \brief Read a value from the ESC. 00045 \param address ESC address 00046 \param *buf pointer to buffer of uint8s in RAM 00047 \param len length of buffer in RAM 00048 \param *tALevent pointer to Application Layer event 00049 */ 00050 uint8 ESC_read(uint16 address, void *buf, uint8 len, void *tALevent) 00051 { 00052 uint8 pstat; 00053 uint8 count; 00054 uint8 *ptr; 00055 uint16union adr; 00056 //SPI_Cmd(SPI1, ENABLE); //pull nSS low 00057 //GPIO_ResetBits(GPIOA, GPIO_Pin_4); 00058 et1100_ss.write(0); 00059 adr.w = address << 3; 00060 pstat = !et1100_miso.read(); //last cmd result; read MISO pin state and invert 00061 ptr = (uint8_t *)tALevent; 00062 *ptr = et1100_spi.write(adr.b[1]); //write first address byte 00063 ptr++; //increase write pointer 00064 *ptr = et1100_spi.write(adr.b[0] + ESC_CMD_READ); //write second address byte, with read command 00065 count = len; // 00066 ptr = (uint8_t *)buf; //let ptr point to the buffer location 00067 while (count > 1) { //while number of received bytes is smaller than len 00068 *ptr = et1100_spi.write(ESC_NEXT); //write dummy byte to start transaction 00069 count--; //decrease bytecounter 00070 ptr++; //increase buffer pointer 00071 } 00072 *ptr = et1100_spi.write( ESC_TERM); //write last byte 00073 00074 //SPI_Cmd(SPI1, DISABLE); //set SS high 00075 et1100_ss.write(1); 00076 return pstat; //return inverted MISO state from previous transaction 00077 } 00078 #endif 00079 00080 #ifdef WSREAD 00081 // use read with wait state byte, needed if SPI>12Mhz or>16KB addressing 00082 /** void ESC_read(uint16 address, void *buf, uint8 len, void *tALevent) 00083 \brief read function for SPI ESC interface ("PDI") 00084 \param address - address of register in ESC 00085 \param *buf pointer to read buffer in microcontroller 00086 \param len length of memory section to read, from start of 'address' 00087 \param *tAlevent microcontroller status register to write response to 00088 */ 00089 uint8 ESC_read(uint16 address, void *buf, uint8 len, void *tALevent) 00090 { 00091 uint8 pstat; 00092 uint8 count; 00093 uint8 *ptr; 00094 uint16union adr; 00095 //SPI_Cmd(SPI1, ENABLE); //pull nSS low 00096 et1100_ss.write(0); 00097 adr.w = address << 3; 00098 pstat = !et1100_miso.read(); 00099 ptr = tALevent; 00100 *ptr = et1100_spi.write(adr.b[1]); //write first address byte 00101 ptr++; //increase write pointer 00102 *ptr = et1100_spi.write(adr.b[0] + ESC_CMD_READWS); //write second address byte, with readws command 00103 et1100_spi.write( ESC_TERM); //write byte to start transaction //COMMENT: Why not ESC_NEXT? 00104 ptr = buf; //change ptr to point to buffer 00105 count = len; // 00106 while (count > 1) { //while number of received bytes is smaller than len 00107 *(ptr) = et1100_spi.write( ESC_NEXT); //write dummy byte to start transaction 00108 count--; //decrease bytecounter 00109 ptr++; //increase buffer pointer 00110 } 00111 *(ptr) = et1100_spi.write( ESC_TERM); //write last byte 00112 //SPI_Cmd(SPI1, DISABLE); //set SS high 00113 et1100_ss.write(1); 00114 return pstat; //return inverted MISO state from previous transaction 00115 } 00116 #endif 00117 00118 /** void ESC_write(uint16 address, void *buf, uint8 len, void *tALevent) 00119 \brief write function for SPI ESC interface ("PDI") 00120 \param address - address of register in ESC 00121 \param *buf pointer to data buffer in microcontroller, buffer contains 'len' bytes to write to location 'address' 00122 \param len length of memory section to write, from start of 'address' 00123 \param *tAlevent microcontroller status register to write response to 00124 */ 00125 uint8 ESC_write(uint16 address, void *buf, uint8 len, void *tALevent) 00126 { 00127 uint8 pstat; 00128 uint8 count; 00129 uint8 *ptr; 00130 uint16union adr; 00131 adr.w = address << 3; //shift 16-bit address value 3 places, then write to adr (union of uint16 and uint8[2]) 00132 pstat = !3; //last cmd result ///////COMMENT: Shouldn't this be pstat = !(PINB & (1 << i_spimiso));? Otherwise just return 0 00133 //SPI_Cmd(SPI1, ENABLE); //pull nSS low 00134 et1100_ss.write(0); 00135 ptr = (uint8_t *)tALevent; //set pointer to tAlevent 00136 *ptr = et1100_spi.write( adr.b[1]); //write first address byte 00137 ptr++; //increase pointer to second byte of tAlevent 00138 *ptr = et1100_spi.write(adr.b[0] + ESC_CMD_WRITE); //write second address byte with write command 00139 count = len; // 00140 ptr = (uint8_t *)buf; //set pointer to buffer location 00141 while (count > 0) { //while number of received bytes is smaller than len 00142 et1100_spi.write( *(ptr)); //write byte from buffer 00143 ptr++; //increase buffer pointer 00144 count--; //decrease counter 00145 } 00146 //SPI_Cmd(SPI1, DISABLE); //set SS high 00147 et1100_ss.write(1); 00148 return pstat; 00149 } 00150 00151
Generated on Wed Jul 13 2022 17:16:52 by
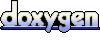