
EtherCAT slave that reads 3 Xsens IMU's connected to a Xbus Master
Dependencies: MODSERIAL mbed KL25Z_ClockControl
Fork of EtherCAT by
esc.h
00001 /* 00002 * SOES Simple Open EtherCAT Slave 00003 * 00004 * File : esc.h 00005 * Version : 1.0.0 00006 * Date : 11-07-2010 00007 * Copyright (C) 2007-2010 Arthur Ketels 00008 * 00009 * SOES is free software; you can redistribute it and/or modify it under 00010 * the terms of the GNU General Public License version 2 as published by the Free 00011 * Software Foundation. 00012 * 00013 * SOES is distributed in the hope that it will be useful, but WITHOUT ANY 00014 * WARRANTY; without even the implied warranty of MERCHANTABILITY or 00015 * FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License 00016 * for more details. 00017 * 00018 * As a special exception, if other files instantiate templates or use macros 00019 * or inline functions from this file, or you compile this file and link it 00020 * with other works to produce a work based on this file, this file does not 00021 * by itself cause the resulting work to be covered by the GNU General Public 00022 * License. However the source code for this file must still be made available 00023 * in accordance with section (3) of the GNU General Public License. 00024 * 00025 * This exception does not invalidate any other reasons why a work based on 00026 * this file might be covered by the GNU General Public License. 00027 * 00028 * The EtherCAT Technology, the trade name and logo "EtherCAT" are the intellectual 00029 * property of, and protected by Beckhoff Automation GmbH. 00030 */ 00031 #ifndef __esc__ 00032 #define __esc__ 00033 00034 // storage modifier for data stored in flashmemory 00035 #define FLASHSTORE const 00036 //#define FLASHSTORE 00037 #define PACKED __attribute__((__packed__)) 00038 00039 #define ESCREG_ADDRESS 0x0010 00040 #define ESCREG_DLSTATUS 0x0110 00041 #define ESCREG_ALCONTROL 0x0120 00042 #define ESCREG_ALSTATUS 0x0130 00043 #define ESCREG_ALERROR 0x0134 00044 #define ESCREG_ALEVENT_SM_MASK 0x0310 00045 #define ESCREG_ALEVENT_SMCHANGE 0x0010 00046 #define ESCREG_ALEVENT_CONTROL 0x0001 00047 #define ESCREG_ALEVENT_SM2 0x0400 00048 #define ESCREG_WDSTATUS 0x0440 00049 #define ESCREG_SM0 0x0800 00050 #define ESCREG_SM0STATUS ESCREG_SM0 + 5 00051 #define ESCREG_SM0PDI ESCREG_SM0 + 7 00052 #define ESCREG_SM1 ESCREG_SM0 + 0x08 00053 #define ESCREG_SM2 ESCREG_SM0 + 0x10 00054 #define ESCREG_SM3 ESCREG_SM0 + 0x18 00055 #define ESCREG_LOCALTIME 0x0910 00056 #define ESCREG_SMENABLE_BIT 0x01 00057 00058 #define ESCinit 0x01 00059 #define ESCpreop 0x02 00060 #define ESCsafeop 0x04 00061 #define ESCop 0x08 00062 #define ESCerror 0x10 00063 00064 #define INIT_TO_INIT 0x11 00065 #define INIT_TO_PREOP 0x21 00066 #define INIT_TO_BOOT 0x31 00067 #define INIT_TO_SAFEOP 0x41 00068 #define INIT_TO_OP 0x81 00069 #define PREOP_TO_INIT 0x12 00070 #define PREOP_TO_PREOP 0x22 00071 #define PREOP_TO_BOOT 0x32 00072 #define PREOP_TO_SAFEOP 0x42 00073 #define PREOP_TO_OP 0x82 00074 #define SAFEOP_TO_INIT 0x14 00075 #define SAFEOP_TO_PREOP 0x24 00076 #define SAFEOP_TO_BOOT 0x34 00077 #define SAFEOP_TO_SAFEOP 0x44 00078 #define SAFEOP_TO_OP 0x84 00079 #define OP_TO_INIT 0x18 00080 #define OP_TO_PREOP 0x28 00081 #define OP_TO_BOOT 0x38 00082 #define OP_TO_SAFEOP 0x48 00083 #define OP_TO_OP 0x88 00084 00085 #define ALERR_NONE 0x0000 00086 #define ALERR_INVALIDSTATECHANGE 0x0011 00087 #define ALERR_UNKNOWNSTATE 0x0012 00088 #define ALERR_BOOTNOTSUPPORTED 0x0013 00089 #define ALERR_INVALIDMBXCONFIG 0x0016 00090 #define ALERR_INVALIDSMCONFIG 0x0017 00091 #define ALERR_WATCHDOG 0x0019 00092 #define ALERR_INVALIDOUTPUTSM 0x001D 00093 #define ALERR_INVALIDINPUTSM 0x001E 00094 00095 #define MBXERR_SYNTAX 0x0001 00096 #define MBXERR_UNSUPPORTEDPROTOCOL 0x0002 00097 #define MBXERR_INVALIDCHANNEL 0x0003 00098 #define MBXERR_SERVICENOTSUPPORTED 0x0004 00099 #define MBXERR_INVALIDHEADER 0x0005 00100 #define MBXERR_SIZETOOSHORT 0x0006 00101 #define MBXERR_NOMOREMEMORY 0x0007 00102 #define MBXERR_INVALIDSIZE 0x0008 00103 00104 #define ABORT_NOTOGGLE 0x05030000 00105 #define ABORT_UNKNOWN 0x05040001 00106 #define ABORT_UNSUPPORTED 0x06010000 00107 #define ABORT_WRITEONLY 0x06010001 00108 #define ABORT_READONLY 0x06010002 00109 #define ABORT_NOOBJECT 0x06020000 00110 #define ABORT_TYPEMISMATCH 0x06070010 00111 #define ABORT_NOSUBINDEX 0x06090011 00112 #define ABORT_GENERALERROR 0x08000000 00113 #define ABORT_NOTINTHISSTATE 0x08000022 00114 00115 #define MBXstate_idle 0x00 00116 #define MBXstate_inclaim 0x01 00117 #define MBXstate_outclaim 0x02 00118 #define MBXstate_outreq 0x03 00119 #define MBXstate_outpost 0x04 00120 #define MBXstate_backup 0x05 00121 #define MBXstate_again 0x06 00122 00123 #define COE_DEFAULTLENGTH 0x0a 00124 #define COE_HEADERSIZE 0x0a 00125 #define COE_SEGMENTHEADERSIZE 0x03 00126 #define COE_SDOREQUEST 0x02 00127 #define COE_SDORESPONSE 0x03 00128 #define COE_SDOINFORMATION 0x08 00129 #define COE_COMMAND_SDOABORT 0x80 00130 #define COE_COMMAND_UPLOADREQUEST 0x40 00131 #define COE_COMMAND_UPLOADRESPONSE 0x40 00132 #define COE_COMMAND_UPLOADSEGMENT 0x00 00133 #define COE_COMMAND_UPLOADSEGREQ 0x60 00134 #define COE_COMMAND_DOWNLOADRESPONSE 0x60 00135 #define COE_COMMAND_LASTSEGMENTBIT 0x01 00136 #define COE_SIZE_INDICATOR 0x01 00137 #define COE_EXPEDITED_INDICATOR 0x02 00138 #define COE_COMPLETEACCESS 0x10 00139 #define COE_TOGGLEBIT 0x10 00140 #define COE_INFOERROR 0x07 00141 #define COE_GETODLISTRESPONSE 0x02 00142 #define COE_GETODRESPONSE 0x04 00143 #define COE_ENTRYDESCRIPTIONRESPONSE 0x06 00144 #define COE_VALUEINFO_ACCESS 0x01 00145 #define COE_VALUEINFO_OBJECT 0x02 00146 #define COE_VALUEINFO_MAPPABLE 0x04 00147 #define COE_VALUEINFO_TYPE 0x08 00148 #define COE_VALUEINFO_DEFAULT 0x10 00149 #define COE_VALUEINFO_MINIMUM 0x20 00150 #define COE_VALUEINFO_MAXIMUM 0x40 00151 00152 ////////////////////////////////////////////////////////// 00153 #define MBXSIZE 0x80 00154 #define MBXBUFFERS 3 00155 00156 #define MBX0_sma 0x1000 00157 #define MBX0_sml MBXSIZE 00158 #define MBX0_sme MBX0_sma+MBX0_sml-1 00159 #define MBX0_smc 0x26 00160 #define MBX1_sma 0x1080 00161 #define MBX1_sml MBXSIZE 00162 #define MBX1_sme MBX1_sma+MBX1_sml-1 00163 #define MBX1_smc 0x22 00164 00165 #define SM2_sma 0x1100 00166 #define SM2_smc 0x24 00167 #define SM2_act 0x01 00168 #define SM3_sma 0x1180 00169 #define SM3_smc 0x20 00170 #define SM3_act 0x01 00171 ///////////////////////////////////////////////////////////// 00172 00173 #define MBXHSIZE sizeof(_MBXh) 00174 #define MBXDSIZE MBXSIZE-MBXHSIZE 00175 00176 #define MBXERR 0x00 00177 #define MBXAOE 0x01 00178 #define MBXEOE 0x02 00179 #define MBXCOE 0x03 00180 #define MBXFOE 0x04 00181 #define MBXODL 0x10 00182 #define MBXOD 0x20 00183 #define MBXED 0x30 00184 #define MBXSEU 0x40 00185 #define MBXSED 0x50 00186 00187 #define SMRESULT_ERRSM0 0x01 00188 #define SMRESULT_ERRSM1 0x02 00189 #define SMRESULT_ERRSM2 0x04 00190 #define SMRESULT_ERRSM3 0x08 00191 00192 // Attention! this struct is always little-endian 00193 typedef struct PACKED 00194 { 00195 uint16 PSA; 00196 uint16 Length; 00197 00198 #if defined(EC_LITTLE_ENDIAN) 00199 uint8 Mode:2; 00200 uint8 Direction:2; 00201 uint8 IntECAT:1; 00202 uint8 IntPDI:1; 00203 uint8 WTE:1; 00204 uint8 R1:1; 00205 00206 uint8 IntW:1; 00207 uint8 IntR:1; 00208 uint8 R2:1; 00209 uint8 MBXstat:1; 00210 uint8 BUFstat:2; 00211 uint8 R3:2; 00212 00213 uint8 ECsm:1; 00214 uint8 ECrep:1; 00215 uint8 ECr4:4; 00216 uint8 EClatchEC:1; 00217 uint8 EClatchPDI:1; 00218 00219 uint8 PDIsm:1; 00220 uint8 PDIrep:1; 00221 uint8 PDIr5:6; 00222 #endif 00223 00224 #if defined(EC_BIG_ENDIAN) 00225 uint8 R1:1; 00226 uint8 WTE:1; 00227 uint8 IntPDI:1; 00228 uint8 IntECAT:1; 00229 uint8 Direction:2; 00230 uint8 Mode:2; 00231 00232 uint8 R3:2; 00233 uint8 BUFstat:2; 00234 uint8 MBXstat:1; 00235 uint8 R2:1; 00236 uint8 IntR:1; 00237 uint8 IntW:1; 00238 00239 uint8 EClatchPDI:1; 00240 uint8 EClatchEC:1; 00241 uint8 ECr4:4; 00242 uint8 ECrep:1; 00243 uint8 ECsm:1; 00244 00245 uint8 PDIr5:6; 00246 uint8 PDIrep:1; 00247 uint8 PDIsm:1; 00248 #endif 00249 } _ESCsm; 00250 00251 // Attention! this struct is always little-endian 00252 typedef struct PACKED 00253 { 00254 uint16 PSA; 00255 uint16 Length; 00256 uint8 Command; 00257 uint8 Status; 00258 uint8 ActESC; 00259 uint8 ActPDI; 00260 } _ESCsm2; 00261 00262 typedef FLASHSTORE struct PACKED 00263 { 00264 uint16 PSA; 00265 uint16 Length; 00266 uint8 Command; 00267 } _ESCsmCompact; 00268 00269 typedef struct 00270 { 00271 uint16 ALevent; 00272 uint16 ALstatus; 00273 uint16 ALcontrol; 00274 uint16 ALerror; 00275 uint16 DLstatus; 00276 uint16 address; 00277 uint8 mbxcnt; 00278 uint8 mbxincnt; 00279 uint8 mbxoutpost; 00280 uint8 mbxbackup; 00281 uint8 xoe; 00282 uint8 txcue; 00283 uint8 mbxfree; 00284 uint8 segmented; 00285 void *data; 00286 uint16 entries; 00287 uint16 frags; 00288 uint16 fragsleft; 00289 00290 #if defined(EC_LITTLE_ENDIAN) 00291 uint8 r1:1; 00292 uint8 toggle:1; 00293 uint8 r2:6; 00294 #endif 00295 00296 #if defined(EC_BIG_ENDIAN) 00297 uint8 r2:6; 00298 uint8 toggle:1; 00299 uint8 r1:1; 00300 #endif 00301 00302 uint8 SMtestresult; 00303 int16 temp; 00304 uint16 wdcnt; 00305 uint32 PrevTime; 00306 uint32 Time; 00307 _ESCsm SM[4]; 00308 } _ESCvar; 00309 00310 typedef struct PACKED 00311 { 00312 uint16 length; 00313 uint16 address; 00314 00315 #if defined(EC_LITTLE_ENDIAN) 00316 uint8 channel:6; 00317 uint8 priority:2; 00318 00319 uint8 mbxtype:4; 00320 uint8 mbxcnt: 4; 00321 #endif 00322 00323 #if defined(EC_BIG_ENDIAN) 00324 uint8 priority:2; 00325 uint8 channel:6; 00326 00327 uint8 mbxcnt: 4; 00328 uint8 mbxtype:4; 00329 #endif 00330 } _MBXh; 00331 00332 typedef struct PACKED 00333 { 00334 _MBXh header; 00335 uint8 b[MBXDSIZE]; 00336 } _MBX; 00337 00338 typedef struct PACKED 00339 { 00340 uint16 numberservice; 00341 } _COEh; 00342 00343 typedef struct PACKED 00344 { 00345 #if defined(EC_LITTLE_ENDIAN) 00346 uint8 opcode:7; 00347 uint8 incomplete:1; 00348 #endif 00349 00350 #if defined(EC_BIG_ENDIAN) 00351 uint8 incomplete:1; 00352 uint8 opcode:7; 00353 #endif 00354 00355 uint8 reserved; 00356 uint16 fragmentsleft; 00357 } _INFOh; 00358 00359 typedef struct PACKED 00360 { 00361 _MBXh mbxheader; 00362 uint16 type; 00363 uint16 detail; 00364 } _MBXerr; 00365 00366 typedef struct PACKED 00367 { 00368 _MBXh mbxheader; 00369 _COEh coeheader; 00370 uint8 command; 00371 uint16 index; 00372 uint8 subindex; 00373 uint32 size; 00374 } _COEsdo; 00375 00376 typedef struct PACKED 00377 { 00378 _MBXh mbxheader; 00379 _COEh coeheader; 00380 _INFOh infoheader; 00381 uint16 index; 00382 uint16 datatype; 00383 uint8 maxsub; 00384 uint8 objectcode; 00385 char name; 00386 } _COEobjdesc; 00387 00388 typedef struct PACKED 00389 { 00390 _MBXh mbxheader; 00391 _COEh coeheader; 00392 _INFOh infoheader; 00393 uint16 index; 00394 uint8 subindex; 00395 uint8 valueinfo; 00396 uint16 datatype; 00397 uint16 bitlength; 00398 uint16 access; 00399 char name; 00400 } _COEentdesc; 00401 00402 // state definition in mailbox 00403 // 0 : idle 00404 // 1 : claimed for inbox 00405 // 2 : claimed for outbox 00406 // 3 : request post outbox 00407 // 4 : outbox posted not send 00408 // 5 : backup outbox 00409 // 6 : mailbox needs to be transmitted again 00410 typedef struct 00411 { 00412 uint8 state; 00413 } _MBXcontrol; 00414 00415 uint16 sizeTXPDO(void); 00416 uint16 sizeRXPDO(void); 00417 uint8 ESC_read(uint16 address,void *buf,uint8 len,void *tALevent); 00418 uint8 ESC_write(uint16 address,void *buf,uint8 len,void *tALevent); 00419 void ESC_ALerror(uint16 errornumber); 00420 void ESC_ALstatus(uint8 status); 00421 void ESC_SMstatus(uint8 n); 00422 uint8 ESC_WDstatus(void); 00423 uint8 ESC_startmbx(uint8 state); 00424 void ESC_stopmbx(void); 00425 uint8 ESC_mbxprocess(void); 00426 void ESC_coeprocess(void); 00427 void ESC_xoeprocess(void); 00428 uint8 ESC_startinput(uint8 state); 00429 void ESC_stopinput(void); 00430 uint8 ESC_startoutput(uint8 state); 00431 void ESC_stopoutput(void); 00432 void ESC_ALevent(void); 00433 void ESC_state(void); 00434 extern void ESC_objecthandler(uint16 index, uint8 subindex); 00435 extern void APP_safeoutput(void); 00436 00437 extern _ESCvar ESCvar; 00438 extern _MBX MBX[MBXBUFFERS]; 00439 extern _MBXcontrol MBXcontrol[MBXBUFFERS]; 00440 extern uint8 MBXrun; 00441 extern uint16 SM2_sml,SM3_sml; 00442 extern uint8 SDO1C12size; 00443 00444 #endif 00445
Generated on Wed Jul 13 2022 17:16:52 by
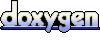