Blynk library for embedded hardware. Works with Arduino, ESP8266, Raspberry Pi, Intel Edison/Galileo, LinkIt ONE, Particle Core/Photon, Energia, ARM mbed, etc. http://www.blynk.cc/
Dependents: Blynk_RBL_BLE_Nano Blynk_MicroBit Blynk_Serial Blynk_RBL_BLE_Nano
BlynkSimpleSerial.h
00001 /** 00002 * @file BlynkSimpleSerial.h 00003 * @author Volodymyr Shymanskyy 00004 * @license This project is released under the MIT License (MIT) 00005 * @copyright Copyright (c) 2015 Volodymyr Shymanskyy 00006 * @date Jan 2015 00007 * @brief 00008 * 00009 */ 00010 00011 #ifndef BlynkSimpleSerial_h 00012 #define BlynkSimpleSerial_h 00013 00014 #ifndef BLYNK_INFO_CONNECTION 00015 #define BLYNK_INFO_CONNECTION "Serial" 00016 #endif 00017 00018 #include <BlynkApiMbed.h > 00019 #include <Blynk/BlynkProtocol.h> 00020 00021 class BlynkTransportSerial 00022 { 00023 public: 00024 BlynkTransportSerial() 00025 : serial(NULL), conn(0) 00026 {} 00027 00028 // IP redirect not available 00029 void begin(char BLYNK_UNUSED *h, uint16_t BLYNK_UNUSED p) {} 00030 00031 void begin(Serial& s) { 00032 serial = &s; 00033 } 00034 00035 bool connect() { 00036 BLYNK_LOG1(BLYNK_F("Connecting...")); 00037 return conn = true; 00038 } 00039 void disconnect() { conn = false; } 00040 00041 size_t read(void* buf, size_t len) { 00042 return fread(buf, 1, len, *serial); 00043 } 00044 size_t write(const void* buf, size_t len) { 00045 return fwrite(buf, 1, len, *serial); 00046 } 00047 00048 bool connected() { return conn; } 00049 int available() { return serial->readable(); } 00050 00051 protected: 00052 Serial* serial; 00053 bool conn; 00054 }; 00055 00056 class BlynkSerial 00057 : public BlynkProtocol<BlynkTransportSerial> 00058 { 00059 typedef BlynkProtocol<BlynkTransportSerial> Base; 00060 public: 00061 BlynkSerial(BlynkTransportSerial& transp) 00062 : Base(transp) 00063 {} 00064 00065 void config(Serial& serial, 00066 const char* auth) 00067 { 00068 Base::begin(auth); 00069 this->conn.begin(serial); 00070 } 00071 00072 void begin(Serial& serial, const char* auth) { 00073 config(serial, auth); 00074 while(this->connect() != true) {} 00075 } 00076 }; 00077 00078 static BlynkTransportSerial _blynkTransport; 00079 BlynkSerial Blynk(_blynkTransport); 00080 00081 #include <BlynkWidgets.h > 00082 00083 #endif
Generated on Tue Jul 12 2022 15:11:12 by
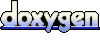