Blynk library for embedded hardware. Works with Arduino, ESP8266, Raspberry Pi, Intel Edison/Galileo, LinkIt ONE, Particle Core/Photon, Energia, ARM mbed, etc. http://www.blynk.cc/
Dependents: Blynk_RBL_BLE_Nano Blynk_MicroBit Blynk_Serial Blynk_RBL_BLE_Nano
BlynkSimpleMicroBit.h
00001 /** 00002 * @file BlynkSimpleRedBearLab_BLE_Nano.h 00003 * @author Volodymyr Shymanskyy 00004 * @license This project is released under the MIT License (MIT) 00005 * @copyright Copyright (c) 2015 Volodymyr Shymanskyy 00006 * @date May 2016 00007 * @brief 00008 * 00009 */ 00010 00011 #ifndef BlynkSimpleRedBearLab_BLE_Nano_h 00012 #define BlynkSimpleRedBearLab_BLE_Nano_h 00013 00014 #ifndef BLYNK_INFO_CONNECTION 00015 #define BLYNK_INFO_CONNECTION "MicroBit" 00016 #endif 00017 00018 #define BLYNK_SEND_ATOMIC 00019 #define BLYNK_SEND_CHUNK 20 00020 #define BLYNK_SEND_THROTTLE 20 00021 00022 #include <BlynkApiMbed.h > 00023 #include <Blynk/BlynkProtocol.h> 00024 #include <utility/BlynkFifo.h> 00025 #include <ble/BLE.h> 00026 00027 /* 00028 * The Nordic UART Service 00029 */ 00030 static const uint8_t uart_base_uuid[] = {0x71, 0x3D, 0, 0, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00031 static const uint8_t uart_base_uuid_rev[] = {0x1E, 0x94, 0x8D, 0xF1, 0x48, 0x31, 0x94, 0xBA, 0x75, 0x4C, 0x3E, 0x50, 0, 0, 0x3D, 0x71}; 00032 static const uint8_t uart_tx_uuid[] = {0x71, 0x3D, 0, 3, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00033 static const uint8_t uart_rx_uuid[] = {0x71, 0x3D, 0, 2, 0x50, 0x3E, 0x4C, 0x75, 0xBA, 0x94, 0x31, 0x48, 0xF1, 0x8D, 0x94, 0x1E}; 00034 00035 #define TXRX_BUF_LEN 20 00036 uint8_t txPayload[TXRX_BUF_LEN] = {0,}; 00037 uint8_t rxPayload[TXRX_BUF_LEN] = {0,}; 00038 00039 GattCharacteristic txCharacteristic (uart_tx_uuid, txPayload, 1, TXRX_BUF_LEN, 00040 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00041 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE); 00042 00043 GattCharacteristic rxCharacteristic (uart_rx_uuid, rxPayload, 1, TXRX_BUF_LEN, 00044 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00045 00046 GattCharacteristic *uartChars[] = {&txCharacteristic, &rxCharacteristic}; 00047 00048 GattService uartService(uart_base_uuid, uartChars, sizeof(uartChars) / sizeof(GattCharacteristic*)); 00049 00050 class BlynkTransportMicroBit 00051 { 00052 public: 00053 BlynkTransportMicroBit() 00054 : mConn (false) 00055 {} 00056 00057 // IP redirect not available 00058 void begin(char* h, uint16_t p) {} 00059 00060 void begin(BLEDevice& bleDevice) { 00061 ble = &bleDevice; 00062 00063 ble->gattServer().addService(uartService); 00064 ble->gattServer().onDataWritten(this, &BlynkTransportMicroBit::writeCallback); 00065 ble->gattServer().onDataSent(this, &BlynkTransportMicroBit::sentCallback); 00066 00067 // Setup advertising 00068 ble->gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS, 00069 uart_base_uuid_rev, sizeof(uart_base_uuid)); 00070 } 00071 00072 bool connect() { 00073 mBuffRX.clear(); 00074 return mConn = true; 00075 } 00076 00077 void disconnect() { 00078 mConn = false; 00079 } 00080 00081 bool connected() { 00082 return mConn; 00083 } 00084 00085 size_t read(void* buf, size_t len) { 00086 millis_time_t start = BlynkMillis(); 00087 while (BlynkMillis() - start < BLYNK_TIMEOUT_MS) { 00088 if (available() < len) { 00089 ble->waitForEvent(); 00090 } else { 00091 break; 00092 } 00093 } 00094 noInterrupts(); 00095 size_t res = mBuffRX.get((uint8_t*)buf, len); 00096 interrupts(); 00097 return res; 00098 } 00099 00100 size_t write(const void* buf, size_t len) { 00101 ble->updateCharacteristicValue(rxCharacteristic.getValueAttribute().getHandle(), (uint8_t*)buf, len); 00102 return len; 00103 } 00104 00105 size_t available() { 00106 noInterrupts(); 00107 size_t rxSize = mBuffRX.size(); 00108 interrupts(); 00109 return rxSize; 00110 } 00111 00112 private: 00113 00114 void writeCallback(const GattWriteCallbackParams *params) 00115 { 00116 noInterrupts(); 00117 //BLYNK_DBG_DUMP(">> ", params->data, params->len); 00118 mBuffRX.put(params->data, params->len); 00119 interrupts(); 00120 } 00121 00122 void sentCallback(unsigned count) 00123 { 00124 //Serial.print("SENT: "); 00125 //Serial.println(count); 00126 } 00127 00128 private: 00129 bool mConn; 00130 BLEDevice* ble; 00131 00132 BlynkFifo<uint8_t, BLYNK_MAX_READBYTES*2> mBuffRX; 00133 }; 00134 00135 class BlynkMicroBit 00136 : public BlynkProtocol<BlynkTransportMicroBit> 00137 { 00138 typedef BlynkProtocol<BlynkTransportMicroBit> Base; 00139 public: 00140 BlynkMicroBit(BlynkTransportMicroBit& transp) 00141 : Base(transp) 00142 {} 00143 00144 void begin(BLEDevice& ble, const char* auth) 00145 { 00146 Base::begin(auth); 00147 state = DISCONNECTED; 00148 conn.begin(ble); 00149 } 00150 }; 00151 00152 static BlynkTransportMicroBit _blynkTransport; 00153 BlynkMicroBit Blynk(_blynkTransport); 00154 00155 #include <BlynkWidgets.h > 00156 00157 #endif
Generated on Tue Jul 12 2022 15:11:12 by
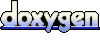