A simple serial shell running on its own thread (stack is statically allocated).
Dependents: FRDM_K64F_IOT lpc1768_blinky
Shell.h
00001 #ifndef _SERIAL_SHELL_H_ 00002 #define _SERIAL_SHELL_H_ 00003 00004 #include "mbed.h" 00005 #include "rtos.h" 00006 00007 #include <string> 00008 #include <map> 00009 00010 #define SHELL_MAX_LINE_LENGTH 64 00011 #define SHELL_MAX_ARGUMENTS 4 00012 00013 typedef void (*shellcmd_t) (Stream *, int , char **); 00014 00015 class Shell { 00016 public: 00017 Shell(Stream * channel); 00018 virtual ~Shell() {} 00019 00020 void addCommand(std::string name, shellcmd_t func); 00021 void start(osPriority priority = osPriorityNormal, 00022 int stackSize = 1024, 00023 unsigned char *stack_pointer=NULL); 00024 00025 private: 00026 static void threadHelper(const void * arg); 00027 00028 void shellMain(); 00029 void shellUsage(const char *p); 00030 bool shellGetLine(char *line, unsigned size); 00031 void listCommands(); 00032 bool cmdExec(char * name, int argc, char *argv[]); 00033 00034 Stream * _chp; 00035 Thread * _thread; 00036 00037 // UART/Serial buffers for 00038 // parsing command line 00039 char line[SHELL_MAX_LINE_LENGTH]; 00040 char *args[SHELL_MAX_ARGUMENTS + 1]; 00041 00042 // commands 00043 std::map<std::string, shellcmd_t> _commands; 00044 }; 00045 00046 #endif
Generated on Tue Jul 12 2022 19:14:34 by
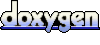