
Fork of my original MQTTGateway
Timer Private Functions
[SPIRIT_Timer]
Functions | |
void | SpiritTimerLdcrMode (SpiritFunctionalState xNewState) |
Enables or Disables the LDCR mode. | |
void | SpiritTimerLdcrAutoReload (SpiritFunctionalState xNewState) |
Enables or Disables the LDCR timer reloading with the value stored in the LDCR_RELOAD registers. | |
SpiritFunctionalState | SpiritTimerLdcrGetAutoReload (void) |
Returns the LDCR timer reload bit. | |
void | SpiritTimerSetRxTimeout (uint8_t cCounter, uint8_t cPrescaler) |
Sets the RX timeout timer initialization registers with the values of COUNTER and PRESCALER according to the formula: Trx=PRESCALER*COUNTER*Tck. | |
void | SpiritTimerSetRxTimeoutMs (float fDesiredMsec) |
Sets the RX timeout timer counter and prescaler from the desired value in ms. | |
void | SpiritTimerSetRxTimeoutCounter (uint8_t cCounter) |
Sets the RX timeout timer counter. | |
void | SpiritTimerSetRxTimeoutPrescaler (uint8_t cPrescaler) |
Sets the RX timeout timer prescaler. | |
void | SpiritTimerGetRxTimeout (float *pfTimeoutMsec, uint8_t *pcCounter, uint8_t *pcPrescaler) |
Returns the RX timeout timer. | |
void | SpiritTimerSetWakeUpTimer (uint8_t cCounter, uint8_t cPrescaler) |
Sets the LDCR wake up timer initialization registers with the values of COUNTER and PRESCALER according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us. | |
void | SpiritTimerSetWakeUpTimerMs (float fDesiredMsec) |
Sets the LDCR wake up timer counter and prescaler from the desired value in ms, according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us. | |
void | SpiritTimerSetWakeUpTimerCounter (uint8_t cCounter) |
Sets the LDCR wake up timer counter. | |
void | SpiritTimerSetWakeUpTimerPrescaler (uint8_t cPrescaler) |
Sets the LDCR wake up timer prescaler. | |
void | SpiritTimerGetWakeUpTimer (float *pfWakeUpMsec, uint8_t *pcCounter, uint8_t *pcPrescaler) |
Returns the LDCR wake up timer, according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us. | |
void | SpiritTimerSetWakeUpTimerReload (uint8_t cCounter, uint8_t cPrescaler) |
Sets the LDCR wake up timer reloading registers with the values of COUNTER and PRESCALER according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us. | |
void | SpiritTimerSetWakeUpTimerReloadMs (float fDesiredMsec) |
Sets the LDCR wake up reload timer counter and prescaler from the desired value in ms, according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us. | |
void | SpiritTimerSetWakeUpTimerReloadCounter (uint8_t cCounter) |
Sets the LDCR wake up timer reload counter. | |
void | SpiritTimerSetWakeUpTimerReloadPrescaler (uint8_t cPrescaler) |
Sets the LDCR wake up timer reload prescaler. | |
void | SpiritTimerGetWakeUpTimerReload (float *pfWakeUpReloadMsec, uint8_t *pcCounter, uint8_t *pcPrescaler) |
Returns the LDCR wake up reload timer, according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us. | |
uint16_t | SpiritTimerGetRcoFrequency (void) |
Computes and returns the RCO frequency. | |
void | SpiritTimerComputeWakeUpValues (float fDesiredMsec, uint8_t *pcCounter, uint8_t *pcPrescaler) |
Computes the values of the wakeup timer counter and prescaler from the user time expressed in millisecond. | |
void | SpiritTimerComputeRxTimeoutValues (float fDesiredMsec, uint8_t *pcCounter, uint8_t *pcPrescaler) |
Computes the values of the rx_timeout timer counter and prescaler from the user time expressed in millisecond. | |
void | SpiritTimerSetRxTimeoutStopCondition (RxTimeoutStopCondition xStopCondition) |
Sets the RX timeout stop conditions. | |
void | SpiritTimerReloadStrobe (void) |
Sends the LDC_RELOAD command to SPIRIT. |
Function Documentation
void SpiritTimerComputeRxTimeoutValues | ( | float | fDesiredMsec, |
uint8_t * | pcCounter, | ||
uint8_t * | pcPrescaler | ||
) |
Computes the values of the rx_timeout timer counter and prescaler from the user time expressed in millisecond.
The prescaler and the counter values are computed maintaining the prescaler value as small as possible in order to obtain the best resolution, and in the meantime minimizing the error.
- Parameters:
-
fDesiredMsec desired rx_timeout in millisecs. This parameter must be a float. Since the counter and prescaler are 8 bit registers the maximum reachable value is maxTime = fTclk x 255 x 255. pcCounter pointer to the variable in which the value for the rx_timeout counter has to be stored. This parameter must be a uint8_t*. pcPrescaler pointer to the variable in which the value for the rx_timeout prescaler has to be stored. This parameter must be an uint8_t*.
- Return values:
-
None
Definition at line 596 of file SPIRIT_Timer.c.
void SpiritTimerComputeWakeUpValues | ( | float | fDesiredMsec, |
uint8_t * | pcCounter, | ||
uint8_t * | pcPrescaler | ||
) |
Computes the values of the wakeup timer counter and prescaler from the user time expressed in millisecond.
The prescaler and the counter values are computed maintaining the prescaler value as small as possible in order to obtain the best resolution, and in the meantime minimizing the error.
- Parameters:
-
fDesiredMsec desired wakeup timeout in millisecs. This parameter must be a float. Since the counter and prescaler are 8 bit registers the maximum reachable value is maxTime = fTclk x 256 x 256. pcCounter pointer to the variable in which the value for the wakeup timer counter has to be stored. This parameter must be a uint8_t*. pcPrescaler pointer to the variable in which the value for the wakeup timer prescaler has to be stored. This parameter must be an uint8_t*.
- Return values:
-
None
Definition at line 539 of file SPIRIT_Timer.c.
uint16_t SpiritTimerGetRcoFrequency | ( | void | ) |
Computes and returns the RCO frequency.
This frequency depends on the xtal frequency and the XTAL bit in register 0x01.
- Return values:
-
RCO frequency in Hz as an uint16_t.
Definition at line 500 of file SPIRIT_Timer.c.
void SpiritTimerGetRxTimeout | ( | float * | pfTimeoutMsec, |
uint8_t * | pcCounter, | ||
uint8_t * | pcPrescaler | ||
) |
Returns the RX timeout timer.
- Parameters:
-
pfTimeoutMsec pointer to the variable in which the timeout expressed in milliseconds has to be stored. If the returned value is 0, it means that the RX_Timeout is infinite. This parameter must be a float*. pcCounter pointer to the variable in which the timer counter has to be stored. This parameter must be an uint8_t*. pcPrescaler pointer to the variable in which the timer prescaler has to be stored. This parameter must be an uint8_t*.
- Return values:
-
None.
Definition at line 268 of file SPIRIT_Timer.c.
void SpiritTimerGetWakeUpTimer | ( | float * | pfWakeUpMsec, |
uint8_t * | pcCounter, | ||
uint8_t * | pcPrescaler | ||
) |
Returns the LDCR wake up timer, according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us.
- Parameters:
-
pfWakeUpMsec pointer to the variable in which the wake-up time expressed in milliseconds has to be stored. This parameter must be a float*. pcCounter pointer to the variable in which the timer counter has to be stored. This parameter must be an uint8_t*. pcPrescaler pointer to the variable in which the timer prescaler has to be stored. This parameter must be an uint8_t*.
- Return values:
-
None.
Definition at line 374 of file SPIRIT_Timer.c.
void SpiritTimerGetWakeUpTimerReload | ( | float * | pfWakeUpReloadMsec, |
uint8_t * | pcCounter, | ||
uint8_t * | pcPrescaler | ||
) |
Returns the LDCR wake up reload timer, according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us.
- Parameters:
-
pfWakeUpReloadMsec pointer to the variable in which the wake-up reload time expressed in milliseconds has to be stored. This parameter must be a float*. pcCounter pointer to the variable in which the timer counter has to be stored. This parameter must be an uint8_t*. pcPrescaler pointer to the variable in which the timer prescaler has to be stored. This parameter must be an uint8_t*.
- Return values:
-
None.
Definition at line 477 of file SPIRIT_Timer.c.
void SpiritTimerLdcrAutoReload | ( | SpiritFunctionalState | xNewState ) |
Enables or Disables the LDCR timer reloading with the value stored in the LDCR_RELOAD registers.
- Parameters:
-
xNewState new state for LDCR reloading. This parameter can be: S_ENABLE or S_DISABLE.
- Return values:
-
None.
Definition at line 150 of file SPIRIT_Timer.c.
SpiritFunctionalState SpiritTimerLdcrGetAutoReload | ( | void | ) |
Returns the LDCR timer reload bit.
- Parameters:
-
None.
- Return values:
-
SpiritFunctionalState,: value of the reload bit.
Definition at line 178 of file SPIRIT_Timer.c.
void SpiritTimerLdcrMode | ( | SpiritFunctionalState | xNewState ) |
Enables or Disables the LDCR mode.
- Parameters:
-
xNewState new state for LDCR mode. This parameter can be: S_ENABLE or S_DISABLE.
- Return values:
-
None.
Definition at line 121 of file SPIRIT_Timer.c.
void SpiritTimerReloadStrobe | ( | void | ) |
Sends the LDC_RELOAD command to SPIRIT.
Reload the LDC timer with the value stored in the LDC_PRESCALER / COUNTER registers.
- Parameters:
-
None.
- Return values:
-
None
Definition at line 676 of file SPIRIT_Timer.c.
void SpiritTimerSetRxTimeout | ( | uint8_t | cCounter, |
uint8_t | cPrescaler | ||
) |
Sets the RX timeout timer initialization registers with the values of COUNTER and PRESCALER according to the formula: Trx=PRESCALER*COUNTER*Tck.
Remember that it is possible to have infinite RX_Timeout writing 0 in the RX_Timeout_Counter and/or RX_Timeout_Prescaler registers.
- Parameters:
-
cCounter value for the timer counter. This parameter must be an uint8_t. cPrescaler value for the timer prescaler. This parameter must be an uint8_t.
- Return values:
-
None.
Definition at line 198 of file SPIRIT_Timer.c.
void SpiritTimerSetRxTimeoutCounter | ( | uint8_t | cCounter ) |
Sets the RX timeout timer counter.
If it is equal to 0 the timeout is infinite.
- Parameters:
-
cCounter value for the timer counter. This parameter must be an uint8_t.
- Return values:
-
None.
Definition at line 235 of file SPIRIT_Timer.c.
void SpiritTimerSetRxTimeoutMs | ( | float | fDesiredMsec ) |
Sets the RX timeout timer counter and prescaler from the desired value in ms.
it is possible to fix the RX_Timeout to a minimum value of 50.417us to a maximum value of about 3.28 s.
- Parameters:
-
fDesiredMsec desired timer value. This parameter must be a float.
- Return values:
-
None
Definition at line 216 of file SPIRIT_Timer.c.
void SpiritTimerSetRxTimeoutPrescaler | ( | uint8_t | cPrescaler ) |
Sets the RX timeout timer prescaler.
If it is equal to 0 the timeout is infinite.
- Parameters:
-
cPrescaler value for the timer prescaler. This parameter must be an uint8_t.
- Return values:
-
None
Definition at line 249 of file SPIRIT_Timer.c.
void SpiritTimerSetRxTimeoutStopCondition | ( | RxTimeoutStopCondition | xStopCondition ) |
Sets the RX timeout stop conditions.
- Parameters:
-
xStopCondition new stop condition. This parameter can be any value of RxTimeoutStopCondition.
- Return values:
-
None
Definition at line 650 of file SPIRIT_Timer.c.
void SpiritTimerSetWakeUpTimer | ( | uint8_t | cCounter, |
uint8_t | cPrescaler | ||
) |
Sets the LDCR wake up timer initialization registers with the values of COUNTER and PRESCALER according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us.
The minimum vale of the wakeup timeout is 28.818us (PRESCALER and COUNTER equals to 0) and the maximum value is about 1.89 s (PRESCALER anc COUNTER equals to 255).
- Parameters:
-
cCounter value for the timer counter. This parameter must be an uint8_t. cPrescaler value for the timer prescaler. This parameter must be an uint8_t.
- Return values:
-
None.
Definition at line 302 of file SPIRIT_Timer.c.
void SpiritTimerSetWakeUpTimerCounter | ( | uint8_t | cCounter ) |
Sets the LDCR wake up timer counter.
Remember that this value is incresead by one in the Twu calculation. Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us
- Parameters:
-
cCounter value for the timer counter. This parameter must be an uint8_t.
- Return values:
-
None.
Definition at line 341 of file SPIRIT_Timer.c.
void SpiritTimerSetWakeUpTimerMs | ( | float | fDesiredMsec ) |
Sets the LDCR wake up timer counter and prescaler from the desired value in ms, according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us.
The minimum vale of the wakeup timeout is 28.818us (PRESCALER and COUNTER equals to 0) and the maximum value is about 1.89 s (PRESCALER anc COUNTER equals to 255).
- Parameters:
-
fDesiredMsec desired timer value. This parameter must be a float.
- Return values:
-
None.
Definition at line 321 of file SPIRIT_Timer.c.
void SpiritTimerSetWakeUpTimerPrescaler | ( | uint8_t | cPrescaler ) |
Sets the LDCR wake up timer prescaler.
Remember that this value is incresead by one in the Twu calculation. Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us
- Parameters:
-
cPrescaler value for the timer prescaler. This parameter must be an uint8_t.
- Return values:
-
None.
Definition at line 356 of file SPIRIT_Timer.c.
void SpiritTimerSetWakeUpTimerReload | ( | uint8_t | cCounter, |
uint8_t | cPrescaler | ||
) |
Sets the LDCR wake up timer reloading registers with the values of COUNTER and PRESCALER according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us.
The minimum vale of the wakeup timeout is 28.818us (PRESCALER and COUNTER equals to 0) and the maximum value is about 1.89 s (PRESCALER anc COUNTER equals to 255).
- Parameters:
-
cCounter reload value for the timer counter. This parameter must be an uint8_t. cPrescaler reload value for the timer prescaler. This parameter must be an uint8_t.
- Return values:
-
None.
Definition at line 405 of file SPIRIT_Timer.c.
void SpiritTimerSetWakeUpTimerReloadCounter | ( | uint8_t | cCounter ) |
Sets the LDCR wake up timer reload counter.
Remember that this value is incresead by one in the Twu calculation. Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us
- Parameters:
-
cCounter value for the timer counter. This parameter must be an uint8_t.
- Return values:
-
None
Definition at line 444 of file SPIRIT_Timer.c.
void SpiritTimerSetWakeUpTimerReloadMs | ( | float | fDesiredMsec ) |
Sets the LDCR wake up reload timer counter and prescaler from the desired value in ms, according to the formula: Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us.
The minimum vale of the wakeup timeout is 28.818us (PRESCALER and COUNTER equals to 0) and the maximum value is about 1.89 s (PRESCALER anc COUNTER equals to 255).
- Parameters:
-
fDesiredMsec desired timer value. This parameter must be a float.
- Return values:
-
None.
Definition at line 424 of file SPIRIT_Timer.c.
void SpiritTimerSetWakeUpTimerReloadPrescaler | ( | uint8_t | cPrescaler ) |
Sets the LDCR wake up timer reload prescaler.
Remember that this value is incresead by one in the Twu calculation. Twu=(PRESCALER +1)*(COUNTER+1)*Tck, where Tck = 28.818 us
- Parameters:
-
cPrescaler value for the timer prescaler. This parameter must be an uint8_t.
- Return values:
-
None
Definition at line 459 of file SPIRIT_Timer.c.
Generated on Tue Jul 12 2022 18:06:48 by
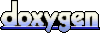