
Fork of my original MQTTGateway
fopen.cpp File Reference
Test cases to POSIX file fopen() interface. More...
Go to the source code of this file.
Functions | |
static int32_t | fsfat_filepath_split (char *filepath, char *parts[], uint32_t num) |
Split a file path into its component parts, setting '/' characters to '\0', and returning pointers to the file path components in the parts array. | |
int32_t | fsfat_filepath_remove_all (char *filepath) |
remove all directories and file in the given filepath | |
static int32_t | fsfat_filepath_make_dirs (char *filepath, bool do_asserts) |
make all directories in the given filepath. | |
static control_t | fsfat_fopen_test_01 (const size_t call_count) |
Basic fopen test which does the following:
| |
control_t | fsfat_fopen_test_02 (const size_t call_count) |
test to fopen() a pre-existing key and try to write it, which should fail as by default pre-existing keys are opened read-only | |
control_t | fsfat_fopen_test_03 (const size_t call_count) |
test to fopen() a pre-existing file and try to write it, which should succeed because the key was opened read-write permissions explicitly | |
control_t | fsfat_fopen_test_04 (const size_t call_count) |
test to call fopen() with a filename string that exceeds the maximum length
| |
control_t | fsfat_fopen_test_05 (const size_t call_count) |
test to call fopen() with filename that in includes illegal characters
| |
control_t | fsfat_fopen_test_06 (const size_t call_count) |
test to call fopen() with filename that in includes illegal characters
| |
control_t | fsfat_fopen_test_07 (const size_t call_count) |
test for errno reporting on a failed fopen()call | |
control_t | fsfat_fopen_test_08 (const size_t call_count) |
test for operation of clearerr() and ferror() | |
control_t | fsfat_fopen_test_09 (const size_t call_count) |
test for operation of ftell() | |
control_t | fsfat_fopen_test_10 (const size_t call_count) |
test for operation of remove() | |
control_t | fsfat_fopen_test_11 (const size_t call_count) |
test for operation of rename() | |
control_t | fsfat_fopen_test_12 (const size_t call_count) |
test for operation of readdir(). | |
control_t | fsfat_fopen_test_13 (const size_t call_count) |
test for operation of mkdir()/remove() | |
control_t | fsfat_fopen_test_14 (const size_t call_count) |
test for operation of stat() | |
control_t | fsfat_fopen_test_15 (const size_t call_count) |
test for operation of SDFileSystem::format() | |
control_t | fsfat_fopen_test_16 (const size_t call_count) |
stress test to write data to fs | |
static control_t | fsfat_fopen_test_dummy () |
fsfat_fopen_test_dummy Dummy test case for testing when platform doesnt have an SDCard installed. |
Detailed Description
Test cases to POSIX file fopen() interface.
Please consult the documentation under the test-case functions for a description of the individual test case.
Definition in file fopen.cpp.
Function Documentation
static int32_t fsfat_filepath_make_dirs | ( | char * | filepath, |
bool | do_asserts | ||
) | [static] |
make all directories in the given filepath.
Do not create the file if present at end of filepath
ARGUMENTS
- Parameters:
-
filepath IN file path containing directories and file do_asserts IN set to true if function should assert on errors
- Returns:
- On success, this returns 0, otherwise < 0 is returned;
int32_t fsfat_filepath_remove_all | ( | char * | filepath ) |
static int32_t fsfat_filepath_split | ( | char * | filepath, |
char * | parts[], | ||
uint32_t | num | ||
) | [static] |
Split a file path into its component parts, setting '/' characters to '\0', and returning pointers to the file path components in the parts array.
For example, if filepath = "/sd/fopentst/hello/world/animal/wobbly/dog/foot/frontlft.txt" then *parts[0] = "sd" *parts[1] = "fopentst" *parts[2] = "hello" *parts[3] = "world" *parts[4] = "animal" *parts[5] = "wobbly" *parts[6] = "dog" *parts[7] = "foot" *parts[8] = "frontlft.txt" parts[9] = NULL
ARGUMENTS
- Parameters:
-
filepath IN file path string to split into component parts. Expected to start with '/' parts IN OUT array to hold pointers to parts num IN number of components available in parts
- Returns:
- On success, this returns the number of components in the filepath Returns number of compoee
static control_t fsfat_fopen_test_01 | ( | const size_t | call_count ) | [static] |
Basic fopen test which does the following:
- creates file and writes some data to the value blob.
- closes the newly created file.
- opens the file (r-only)
- reads the file data and checks its the same as the previously created data.
- closes the opened file
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
control_t fsfat_fopen_test_02 | ( | const size_t | call_count ) |
test to fopen() a pre-existing key and try to write it, which should fail as by default pre-existing keys are opened read-only
Basic open test which does the following:
- creates file with default rw perms and writes some data to the value blob.
- closes the newly created file.
- opens the file with the default permissions (read-only)
- tries to write the file data which should fail because file was not opened with write flag set.
- closes the opened key
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
control_t fsfat_fopen_test_03 | ( | const size_t | call_count ) |
test to fopen() a pre-existing file and try to write it, which should succeed because the key was opened read-write permissions explicitly
Basic open test which does the following:
- creates file with default rw perms and writes some data to the value blob.
- closes the newly created file.
- opens the file with the rw permissions (non default)
- tries to write the file data which should succeeds because file was opened with write flag set.
- closes the opened key
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
control_t fsfat_fopen_test_04 | ( | const size_t | call_count ) |
test to call fopen() with a filename string that exceeds the maximum length
- chanFS supports the exFAT format which should support 255 char filenames
- check that filenames of this length can be created
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
control_t fsfat_fopen_test_05 | ( | const size_t | call_count ) |
test to call fopen() with filename that in includes illegal characters
- the character(s) can be at the beginning of the filename
- the character(s) can be at the end of the filename
- the character(s) can be somewhere within the filename string
- a max-length string of random characters (legal and illegal)
- a max-length string of random illegal characters only
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
control_t fsfat_fopen_test_06 | ( | const size_t | call_count ) |
control_t fsfat_fopen_test_07 | ( | const size_t | call_count ) |
test for errno reporting on a failed fopen()call
This test does the following:
- tries to open a file that does not exist for reading, and checks that a NULL pointer is returned.
- checks that errno is not 0 as there is an error.
- checks that ferror() returns 1 indicating an error exists.
Note: see NOTE_1 below.
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
control_t fsfat_fopen_test_08 | ( | const size_t | call_count ) |
test for operation of clearerr() and ferror()
The test does the following:
- opens and then closes a file, but keeps a copy of the FILE pointer fp.
- set errno to 0.
- write to the close file with fwrite(fp) which should return 0 (no writes) and set the errno.
- check the error condition is set with ferror().
- clear the error with clearerr().
- check the error condition is reset with ferror().
NOTE_1: GCC/ARMCC support for setting errno
- Documentation (e.g. fwrite() man page) does not explicity say fwrite() sets errno (e.g. for an fwrite() on a read-only file).
- GCC libc fwrite() appears to set errno as expected.
- ARMCC & IAR libc fwrite() appears not to set errno.
The following ARMCC documents are silent on whether fwrite() sets errno:
- "ARM C and C++ Libraries and Floating-Point Support".
- "RL-ARM User Guide fwrite() section".
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
control_t fsfat_fopen_test_09 | ( | const size_t | call_count ) |
control_t fsfat_fopen_test_10 | ( | const size_t | call_count ) |
test for operation of remove()
Performs the following tests: 1. test remove() on a file that exists. This should succeed. 2. test remove() on a dir that exists. This should succeed. 3. test remove() on a file that doesnt exist. This should fail. check errno set. 4. test remove() on a dir that doesnt exist. This should fail. check errno set.
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
control_t fsfat_fopen_test_11 | ( | const size_t | call_count ) |
test for operation of rename()
This test does the following: 1) test rename() on a file that exists to a new filename within the same directory. 2) test rename() on a file that exists to a new filename within a different directory.
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
control_t fsfat_fopen_test_12 | ( | const size_t | call_count ) |
control_t fsfat_fopen_test_13 | ( | const size_t | call_count ) |
test for operation of mkdir()/remove()
This test checks that:
- The mkdir() function successfully creates a directory that is not already present.
- The mkdir() function returns EEXIST when trying to create a directory thats already present.
- The remove() function successfully removes a directory that is present.
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
control_t fsfat_fopen_test_14 | ( | const size_t | call_count ) |
control_t fsfat_fopen_test_15 | ( | const size_t | call_count ) |
control_t fsfat_fopen_test_16 | ( | const size_t | call_count ) |
Generated on Tue Jul 12 2022 18:06:47 by
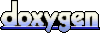