
As of Monday morning, so this is the code we showed at Uncraftivism.
Embed:
(wiki syntax)
Show/hide line numbers
ucam.h
00001 00002 // This is a class for talking to the 4DSystems uCam JPEG camera module 00003 // from an mbed (mbed.org) 00004 00005 // Datasheet: 00006 // http://www.4dsystems.com.au/downloads/micro-CAM/Docs/uCAM-DS-rev2.pdf 00007 00008 00009 // Commands: 00010 #define UCAM_INITIAL 0xAA01 00011 #define UCAM_GET_PICTURE 0xAA04 00012 #define UCAM_SNAPSHOT 0xAA05 00013 #define UCAM_SET_PACKAGE_SIZE 0xAA06 00014 #define UCAM_RESET 0xAA08 00015 #define UCAM_DATA 0xAA0A 00016 #define UCAM_SYNC 0xAA0D 00017 #define UCAM_ACK 0xAA0E 00018 #define UCAM_ACK 0xAA0E 00019 #define UCAM_NAK 0xAA0F 00020 #define UCAM_LIGHT 0xAA13 00021 00022 00023 // Picture types; 00024 #define UCAM_PIC_TYPE_SNAPSHOT 0x01 // gets the pic taken with the SNAPSHOT command 00025 // in which case, the actualy type of the picture is the one specified by the SNAPSHOT 00026 00027 #define UCAM_PIC_TYPE_RAW_PREVIEW 0x02 // gets the current pic, doesn't require the SNAPSHOT command 00028 #define UCAM_PIC_TYPE_JPEG_PREVIEW 0x05 // gets the current pic, doesn't require the SNAPSHOT command 00029 00030 00031 00032 #define UCAM_COLOUR_2_BIT_GREY 0x01 //2-bit Gray Scale (RAW, 2-bit for Y only) 01h 00033 #define UCAM_COLOUR_4_BIT_GREY 0x02 //4-bit Gray Scale (RAW, 4-bit for Y only) 02h 00034 #define UCAM_COLOUR_8_BIT_GREY 0x03 // (RAW, 8-bit for Y only) 00035 #define UCAM_COLOUR_8_BIT_COLOUR 0x04 // (RAW, 332(RGB)) 00036 00037 #define UCAM_COLOUR_12_BIT_COLOR 0x05 // (RAW, 444(RGB)) 05h 00038 #define UCAM_COLOUR_16_BIT_COLOR 0x06 //(RAW, 565(RGB)) 06h 00039 #define UCAM_COLOUR_JPEG 0x07 00040 00041 #define UCAM_COLOUR_NOT_SET 0xFF 00042 00043 00044 // Sizes for raw images 00045 #define UCAM_RAW_SIZE_80x60 0x01 00046 #define UCAM_RAW_SIZE_160x120 0x03 00047 #define UCAM_RAW_SIZE_320x240 0x05 00048 #define UCAM_RAW_SIZE_640x480 0x07 00049 #define UCAM_RAW_SIZE_128x128 0x09 00050 #define UCAM_RAW_SIZE_128x96 0x0B 00051 00052 // Sizes for jpeg images 00053 00054 #define UCAM_JPEG_SIZE_80x64 0x01 00055 #define UCAM_JPEG_SIZE_160x128 0x03 00056 #define UCAM_JPEG_SIZE_320x240 0x05 00057 #define UCAM_JPEG_SIZE_640x480 0x07 00058 00059 // Snapshot types 00060 #define UCAM_SNAPSHOT_JPEG 0x00 00061 #define UCAM_SNAPSHOT_RAW 0x01 00062 00063 #ifndef FILE_WRITE_STRING 00064 #define FILE_WRITE_STRING "w" // value for mbed - will be defined earlier on Win 00065 #define FILE_READ_STRING "r" 00066 #endif 00067 00068 class Frame; 00069 00070 #include "SerialBuffered.h" 00071 00072 class UCam 00073 { 00074 public: 00075 00076 UCam(PinName tx, PinName rx); 00077 00078 void doStartup(); 00079 int doConfig( bool raw, uint8_t colourType, uint8_t imageSize ); 00080 00081 Frame *doGetRawPictureToBuffer( uint8_t picType ); 00082 int doGetJpegPictureToFile( uint8_t picType, char* filename ); 00083 00084 private: 00085 00086 int doConnect(); 00087 int fixConfusion(); 00088 void sendCommand( int command, int p1, int p2, int p3, int p4 ); 00089 int doCommand( int command, int p1, int p2, int p3, int p4 ); 00090 int doReset(); 00091 int doSyncs(); 00092 int doSnapshot( uint8_t snapshotType ); 00093 00094 void sendAck(); 00095 void sendAckForPackage( uint16_t p); 00096 void sendAckForRawData( ) ; 00097 int readAckPatiently( uint16_t command ); 00098 int readAck( uint16_t command ); 00099 int readSync(); 00100 uint32_t readData(); 00101 int readBytes(uint8_t *bytes, int size ); 00102 00103 uint16_t readUInt16(); 00104 int readPackage( FILE *jpgFile, uint16_t targetPackage ); 00105 00106 00107 00108 private: 00109 SerialBuffered camSerial; 00110 //Serial camSerial; 00111 uint8_t lastCommand; 00112 00113 int m_confused; 00114 00115 uint8_t packageBody[512]; 00116 00117 bool m_raw; 00118 uint8_t m_colourType; 00119 uint8_t m_imageSize; 00120 00121 uint8_t m_bitsPerPixel; 00122 uint16_t m_width; 00123 uint16_t m_height; 00124 00125 00126 00127 };
Generated on Wed Jul 27 2022 03:18:56 by
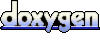