
Sample code that uses EvrythngApi library.
Dependencies: EvrythngApi mbed
main.cpp
00001 /* 00002 * (c) Copyright 2012 EVRYTHNG Ltd London / Zurich 00003 * www.evrythng.com 00004 * 00005 * --- DISCLAIMER --- 00006 * 00007 * EVRYTHNG provides this source code "as is" and without warranty of any kind, 00008 * and hereby disclaims all express or implied warranties, including without 00009 * limitation warranties of merchantability, fitness for a particular purpose, 00010 * performance, accuracy, reliability, and non-infringement. 00011 * 00012 * 00013 * --- READ ME --- 00014 * 00015 * This is a demo application that uses EVRYTHNG's mbed wrapper to read and 00016 * write value to EVRYTHNG's engine. Please refer to the online documentation 00017 * available at http://dev.evrythng.com/mbed. 00018 * 00019 * 00020 * Author: Michel Yerly 00021 * 00022 */ 00023 00024 #include "mbed.h" 00025 #include "EthernetInterface.h" 00026 #include <time.h> 00027 #include "EvrythngApi.h" 00028 #include "util.h" 00029 #include "eventqueue.h" 00030 00031 /* 00032 * Configuration 00033 */ 00034 00035 // Your EVRYTHNG api key. 00036 const char* API_KEY = ""; 00037 00038 // Your thng id, the one that represents your mbed device. 00039 const char* THNG_ID = ""; 00040 00041 // The property you want values to be pushed to. 00042 const char* THNG_PROP_SET = "prop3"; 00043 00044 // The property you want values to be read from. 00045 const char* THNG_PROP_GET = "prop3"; 00046 00047 00048 00049 DigitalOut led1(LED1); 00050 DigitalOut led2(LED2); 00051 DigitalOut led3(LED3); 00052 DigitalOut led4(LED4); 00053 00054 static char displayVal = 0; 00055 00056 /* 00057 * Displays a value between 0 and 15 as binary on the 4 LEDs. 00058 */ 00059 void display(char x) 00060 { 00061 led1 = x & (char)8 ? 1 : 0; 00062 led2 = x & (char)4 ? 1 : 0; 00063 led3 = x & (char)2 ? 1 : 0; 00064 led4 = x & (char)1 ? 1 : 0; 00065 displayVal = x; 00066 } 00067 00068 /* 00069 * Flashes the 4 LEDs, and restores the state of the last display(). 00070 */ 00071 void flash() 00072 { 00073 char s = displayVal; 00074 for (int i = 0; i < 2; ++i) { 00075 display(0x0F); 00076 wait(0.1); 00077 display(0x00); 00078 wait(0.1); 00079 } 00080 display(s); 00081 } 00082 00083 /* 00084 * Function to get the number of milliseconds elapsed since the system startup. 00085 * It is imprecise and if called repetitively and quickly, it may always return 00086 * the same result. It MUST be called at least every 30 minutes. 00087 * NOTE: I'm not proud of it. 00088 */ 00089 int64_t getMillis() 00090 { 00091 static Timer tmr; 00092 static int64_t clk = 0; 00093 00094 tmr.stop(); 00095 clk += tmr.read_ms(); 00096 tmr.reset(); 00097 tmr.start(); 00098 00099 char str[21]; 00100 char* end; 00101 sprinti64(str, clk, &end); 00102 *end = '\0'; 00103 dbg.printf("%s ", str); 00104 00105 return clk; 00106 } 00107 00108 /* 00109 * Entry point. 00110 * 00111 * This demo application uses an event loop to set and get property values 00112 * on EVRYTHNG engine at given intervals. 00113 */ 00114 int main() 00115 { 00116 dbg.printf("Initializing ethernet interface\r\n"); 00117 EthernetInterface eth; 00118 eth.init(); //Use DHCP 00119 eth.connect(); 00120 dbg.printf("IP address: %s\r\n", eth.getIPAddress()); 00121 00122 EvrythngApi api(API_KEY); 00123 00124 // intervals in ms 00125 const int SET_INTERVAL = 5000; 00126 const int GET_INTERVAL = 2000; 00127 00128 int curVal = 0; 00129 display(curVal); 00130 00131 int putVal = 0; 00132 char strPutVal[3]; 00133 int64_t timestamp = 1345736694000LL; // 2012-08-23 10:44:54am EST 00134 00135 EventQueue eventQueue; 00136 eventQueue.put(getMillis()+GET_INTERVAL, EVT_GET_PROP); 00137 eventQueue.put(getMillis()+SET_INTERVAL, EVT_SET_PROP); 00138 00139 while (!eventQueue.empty()) { 00140 EventType event = eventQueue.waitNext(getMillis()); 00141 00142 switch (event) { 00143 00144 case EVT_SET_PROP: 00145 00146 eventQueue.put(SET_INTERVAL + getMillis(), EVT_SET_PROP); 00147 00148 putVal = (putVal + 1) & 0xF; 00149 sprintf(strPutVal, "%d", putVal); 00150 timestamp++; 00151 api.setThngPropertyValue(THNG_ID, THNG_PROP_SET, string(strPutVal), timestamp); 00152 00153 break; 00154 00155 case EVT_GET_PROP: 00156 00157 eventQueue.put(GET_INTERVAL + getMillis(), EVT_GET_PROP); 00158 00159 string v; 00160 int r = api.getThngPropertyValue(THNG_ID, THNG_PROP_GET, v); 00161 if (r == 0) { 00162 flash(); 00163 int newVal = atoi(v.c_str()); 00164 if (newVal != curVal) { 00165 curVal = newVal; 00166 display(curVal); 00167 } 00168 } 00169 break; 00170 } 00171 } 00172 00173 dbg.printf("Disconnecting ethernet interface\r\n"); 00174 eth.disconnect(); 00175 00176 dbg.printf("Done.\r\n"); 00177 while(1) {} 00178 }
Generated on Fri Jul 15 2022 21:25:04 by
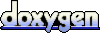