
Sample code that uses EvrythngApi library.
Dependencies: EvrythngApi mbed
eventqueue.h
00001 /* 00002 * (c) Copyright 2012 EVRYTHNG Ltd London / Zurich 00003 * www.evrythng.com 00004 * 00005 * --- DISCLAIMER --- 00006 * 00007 * EVRYTHNG provides this source code "as is" and without warranty of any kind, 00008 * and hereby disclaims all express or implied warranties, including without 00009 * limitation warranties of merchantability, fitness for a particular purpose, 00010 * performance, accuracy, reliability, and non-infringement. 00011 * 00012 * Author: Michel Yerly 00013 * 00014 */ 00015 #include <queue> 00016 #include <stdint.h> 00017 00018 enum EventType { 00019 EVT_SET_PROP, 00020 EVT_GET_PROP 00021 }; 00022 00023 struct Event { 00024 int64_t time; 00025 EventType type; 00026 }; 00027 00028 class EventComparison 00029 { 00030 public: 00031 bool operator() (const Event& lhs, const Event&rhs) const { 00032 return (lhs.time>rhs.time); 00033 } 00034 }; 00035 00036 class EventQueue 00037 { 00038 public: 00039 void put(int64_t time, EventType type); 00040 EventType waitNext(int64_t currentTime); 00041 bool empty(); 00042 int size(); 00043 private: 00044 std::priority_queue<Event, std::vector<Event>, EventComparison> queue; 00045 };
Generated on Fri Jul 15 2022 21:25:04 by
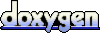