Api wrapper to communicate with EVRYTHNG's Engine.
Dependencies: EthernetInterface mbed-rtos
Dependents: EvrythngApiExample
JsonParser.h
00001 /* 00002 * (c) Copyright 2012 EVRYTHNG Ltd London / Zurich 00003 * www.evrythng.com 00004 * 00005 * --- DISCLAIMER --- 00006 * 00007 * EVRYTHNG provides this source code "as is" and without warranty of any kind, 00008 * and hereby disclaims all express or implied warranties, including without 00009 * limitation warranties of merchantability, fitness for a particular purpose, 00010 * performance, accuracy, reliability, and non-infringement. 00011 * 00012 * Author: Michel Yerly 00013 * 00014 */ 00015 #ifndef JSONPARSER_H 00016 #define JSONPARSER_H 00017 00018 #include <vector> 00019 #include <map> 00020 #include <string> 00021 00022 /* 00023 * Possible JSON value types 00024 */ 00025 enum ValueType { 00026 00027 VT_DOUBLE, // Number 00028 VT_CHAR_PTR, // String 00029 VT_VEC_PTR, // Array 00030 VT_MAP_PTR, // Object 00031 VT_CST_NULL, // Null 00032 VT_CST_TRUE, // True 00033 VT_CST_FALSE // False 00034 }; 00035 00036 /* 00037 * Class to hold a JSON value. A JSON value may be composed of other JSON 00038 * values. 00039 */ 00040 class JsonValue 00041 { 00042 public: 00043 00044 /* 00045 * Creates a new string JSON value. The string is copied into the object. 00046 * WARNING: a new object is created. The caller is responsible of deleting 00047 * it. 00048 * buffer: the string to read from. 00049 * len: the number of characters to read. 00050 */ 00051 static JsonValue* createString(const char* buffer, int len); 00052 00053 /* Creates a new number JSON value. 00054 * WARNING: a new object is created. The caller is responsible of deleting 00055 * it. 00056 */ 00057 static JsonValue* createDouble(double d); 00058 00059 /* Creates a new object JSON value. 00060 * WARNING: a new object is created. The caller is responsible of deleting 00061 * it. 00062 */ 00063 static JsonValue* createMap(); 00064 00065 /* Creates a new array JSON value. 00066 * WARNING: a new object is created. The caller is responsible of deleting 00067 * it. 00068 */ 00069 static JsonValue* createVector(); 00070 00071 /* Creates a new true or false JSON value, depending on b. 00072 * WARNING: a new object is created. The caller is responsible of deleting 00073 * it. 00074 */ 00075 static JsonValue* createBoolean(bool b); 00076 00077 /* Creates a new null JSON value. 00078 * WARNING: a new object is created. The caller is responsible of deleting 00079 * it. 00080 */ 00081 static JsonValue* createNull(); 00082 00083 /* 00084 * Destructor. 00085 * The destructor also deletes nested JsonValues if any. 00086 */ 00087 virtual ~JsonValue(); 00088 00089 /* 00090 * Gets a JSON value given a path. 00091 * If the path is NULL or an empty string the method returns this. 00092 * If there is an error, the method returns NULL. 00093 * The path is composed of strings (object keys) and numbers (array 00094 * indices) separated by '/'. 00095 * 00096 * Example: 00097 * JSON: {"xy":34,"ab":[{"r":true},{"s":false}]} 00098 * Path: ab/1/s 00099 * Returns: a JsonValue representing false. 00100 */ 00101 JsonValue* get(const char* path); 00102 00103 /* 00104 * Gets a pointer to a double given a path (see get()). 00105 * If there is an error, the method returns NULL. 00106 */ 00107 const double* getDouble(const char* path); 00108 00109 /* 00110 * Gets a pointer to a string given a path (see get()). 00111 * If there is an error, the method returns NULL. 00112 */ 00113 const char* getString(const char* path); 00114 00115 /* 00116 * Gets a pointer to an array given a path (see get()). 00117 * If there is an error, the method returns NULL. 00118 */ 00119 const std::vector<JsonValue*>* getVector(const char* path); 00120 00121 /* 00122 * Gets a pointer to a map given a path (see get()). 00123 * If there is an error, the method returns NULL. 00124 */ 00125 const std::map<std::string,JsonValue*>* getMap(const char* path); 00126 00127 /* 00128 * Gets a pointer to a boolean given a path (see get()). 00129 * If there is an error, the method returns NULL. 00130 */ 00131 const bool* getBoolean(const char* path); 00132 00133 /* 00134 * Determines if the value at the path (see get()) is null. 00135 * Return true if and only if the value is explicitely null. 00136 */ 00137 bool isNull(const char* path); 00138 00139 /* 00140 * Debug function. 00141 */ 00142 void print(); 00143 00144 friend class JsonParser; 00145 00146 private: 00147 00148 /* 00149 * Constructor that creates a string JSON value. The string is copied into 00150 * the object. 00151 * buffer: the string to read from. 00152 * len: the number of characters to read. 00153 */ 00154 JsonValue(const char* buffer, int len); 00155 00156 /* 00157 * Constructor that creates a number JSON value. 00158 */ 00159 JsonValue(double d); 00160 00161 /* 00162 * Constructor that creates either a True or a False JSON value. 00163 */ 00164 JsonValue(bool b); 00165 00166 /* 00167 * Constructor that creates a JSON value without specifying the type. 00168 */ 00169 JsonValue(); 00170 00171 ValueType type; 00172 union { 00173 double d; 00174 char* s; 00175 std::vector<JsonValue*>* vec; 00176 std::map<std::string,JsonValue*>* map; 00177 } value; 00178 00179 }; 00180 00181 enum TokenType { 00182 TOKEN_EOS, 00183 TOKEN_NUMBER, 00184 TOKEN_STRING, 00185 TOKEN_DELIMITER, 00186 TOKEN_TRUE, 00187 TOKEN_FALSE, 00188 TOKEN_NULL 00189 }; 00190 00191 /* 00192 * Class to parse a JSON string. 00193 * 00194 * NOTE: The current implementation does only support ASCII encoding. 00195 */ 00196 class JsonParser 00197 { 00198 public: 00199 00200 /* 00201 * Constructor. 00202 */ 00203 JsonParser(); 00204 00205 /* 00206 * Destructor. 00207 * The destructor also deletes the parsed document (the one you get with 00208 * getDocument(). 00209 */ 00210 virtual ~JsonParser(); 00211 00212 /* 00213 * Parses the json string. 00214 * json: String that contains the json to parse. 00215 * Returns 0 on success, or -1 on error. 00216 * 00217 * NOTE: This method deletes the previously parsed document. 00218 */ 00219 int parse(const char* json); 00220 00221 /* 00222 * Returns the parsed document, or NULL if the json is not parsed. 00223 */ 00224 JsonValue* getDocument(); 00225 00226 private: 00227 const char* json; 00228 int json_len; 00229 TokenType ct; 00230 int ctPos; 00231 int ctLen; 00232 double ctNumberVal; 00233 JsonValue* pDocument; 00234 00235 int goToNextToken(); 00236 00237 int parseObject(JsonValue** object); 00238 int parseValue(JsonValue** value); 00239 int parseArray(JsonValue** array); 00240 }; 00241 00242 #endif
Generated on Tue Jul 12 2022 16:31:38 by
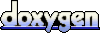