PCA9672 Library (I2C IO Expander Interface)
Embed:
(wiki syntax)
Show/hide line numbers
PCA9672.h
00001 /* mbed PCA9672 I2C I/O Expander Library 00002 * Copyright (c) 2013 viswesr 00003 * 00004 * MIT License 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00007 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00008 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00009 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in all copies or 00013 * substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00016 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00017 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00018 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00020 */ 00021 00022 #ifndef PCA9672_H 00023 #define PCA9672_H 00024 00025 #include "mbed.h" 00026 00027 // PCA9672 IIC slave address 00028 #define PCA9672_ADDR 0x46 00029 00030 00031 //!Library for the PCA9672 I/O expander. 00032 /*! 00033 The PCA9672 is an I2C I/O expander. It has 8 I/O pins. 00034 */ 00035 class PCA9672 00036 { 00037 public: 00038 /*! 00039 Connect PCA9672 to I2C port pins sda and scl. 00040 */ 00041 PCA9672 (PinName sda, PinName scl); 00042 00043 /*! 00044 Set the frequency of the I2C interface. 00045 */ 00046 void frequency (int hz); 00047 00048 /*! 00049 Write the value to the IO Expander (pins XP0-XP7 output) 00050 */ 00051 void write (char value); 00052 00053 /*! 00054 Read the value of the IO Expander (pins XP0-XP7 input) 00055 */ 00056 int read (void); 00057 00058 /*! 00059 Destroys instance. 00060 */ 00061 ~PCA9672 (); 00062 00063 private: 00064 00065 I2C _i2c; 00066 00067 }; 00068 00069 #endif
Generated on Tue Jul 12 2022 18:49:51 by
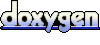