PCA9672 Library (I2C IO Expander Interface)
Embed:
(wiki syntax)
Show/hide line numbers
PCA9672.cpp
00001 /* mbed PCA9672 I2C I/O Expander Library 00002 * Copyright (c) 2013 viswesr 00003 * 00004 * MIT License 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00007 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00008 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00009 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in all copies or 00013 * substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00016 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00017 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00018 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00020 */ 00021 00022 #include "PCA9672.h" 00023 00024 PCA9672::PCA9672 (PinName sda, PinName scl) : _i2c(sda, scl) 00025 { 00026 // Software Reset 00027 _i2c.start(); 00028 while(_i2c.write(0x00) !=1); 00029 while(_i2c.write(0x06) !=1); 00030 _i2c.stop(); 00031 00032 /* Software reset is not required. But, gives an 00033 indication if the selected I2C bus frequency works */ 00034 } 00035 00036 void PCA9672::frequency (int hz) 00037 { 00038 _i2c.frequency(hz); 00039 } 00040 00041 void PCA9672::write (char value) 00042 { 00043 _i2c.write(PCA9672_ADDR, &value, 1); 00044 } 00045 00046 int PCA9672::read (void) 00047 { 00048 _i2c.read(PCA9672_ADDR | 0x01); 00049 } 00050 00051 PCA9672::~PCA9672 () 00052 { 00053 00054 }
Generated on Tue Jul 12 2022 18:49:51 by
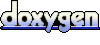