Shutter release remote for D3200 camera. Should work with other DSLR cameras that support ML-L3 IR remote.
Fork of HelloWorld by
main.cpp
00001 /* IR remote for D3200 DSLR camera. Should work with other DSLR cameras that support ML-L3 remote. Implemented with mbed 00002 based on a nice documentation of ML-L3 protocol by Michele Bighignoli (http://www.bigmike.it/ircontrol/) */ 00003 00004 #include "mbed.h" 00005 00006 /* P0_9 is connected to an IR LED and P1_14 to a button in Seeedstudio Arch. 00007 Change these pins as per your platform. */ 00008 #define LED_PIN P0_9 00009 #define BUTTON_PIN P1_14 00010 00011 PwmOut IRled(LED_PIN); /* Connect a suitable current limiting resistor. 00012 For longer range, use a transistor for driving IR LED */ 00013 00014 DigitalIn button(BUTTON_PIN); /* Push button to enable shutter release */ 00015 00016 void shutterrelease(); 00017 00018 int main() 00019 { 00020 button.mode(PullDown); 00021 IRled.period_us(26); // set PWM period for Carrier Frequency of 38.4 KHz 00022 IRled.write(0); 00023 00024 while(1) { 00025 if(button) { 00026 shutterrelease(); 00027 } 00028 } 00029 00030 } 00031 00032 void shutterrelease() 00033 { 00034 /* Shutter release command sequence */ 00035 IRled.write(0.5); 00036 wait_us(2000); 00037 IRled.write(0); 00038 wait_us(27830); 00039 IRled.write(0.5); 00040 wait_us(400); 00041 IRled.write(0); 00042 wait_us(1580); 00043 IRled.write(0.5); 00044 wait_us(400); 00045 IRled.write(0); 00046 wait_us(3580); 00047 IRled.write(0.5); 00048 wait_us(400); 00049 IRled.write(0); 00050 wait_us(63200); // Wait for 63.2ms and repeat the above sequence. 00051 IRled.write(0.5); 00052 wait_us(2000); 00053 IRled.write(0); 00054 wait_us(27830); 00055 IRled.write(0.5); 00056 wait_us(400); 00057 IRled.write(0); 00058 wait_us(1580); 00059 IRled.write(0.5); 00060 wait_us(400); 00061 IRled.write(0); 00062 wait_us(3580); 00063 IRled.write(0.5); 00064 wait_us(400); 00065 IRled.write(0); 00066 }
Generated on Wed Jul 13 2022 02:47:23 by
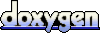