Seeedstudio Arch Examples : Grove Serial LCD - Display temperature using Grove-Temperature
Dependencies: Grove_Serial_LCD mbed
Fork of Arch_GroveSerialLCD_Ex1 by
main.cpp
00001 #include "mbed.h" 00002 #include "SerialLCD.h" 00003 00004 SerialLCD lcd(P1_13, P1_14); /* Grove Serial LCD is connected to UART Tx and Rx pins*/ 00005 00006 AnalogIn thermistor(P0_11); /* Thermistor output connected to P0_11 */ 00007 00008 int main() 00009 { 00010 char strBuffer[16]; 00011 unsigned int a, beta = 3975; 00012 float temperature, resistance; 00013 00014 lcd.begin(); /* initialize Serial LCD communication. */ 00015 00016 while(1) { 00017 a = thermistor.read_u16(); /* Read analog value */ 00018 00019 /* Calculate the resistance of the thermistor from analog votage read. */ 00020 resistance= (float) 10000.0 * ((65536.0 / a) - 1.0); 00021 00022 /* Convert the resistance to temperature using Steinhart's Hart equation */ 00023 temperature=(1/((log(resistance/5000.0)/beta) + (1.0/298.15)))-273.15; 00024 00025 sprintf(strBuffer, "Tmp %4.2f deg C", temperature); /* prepare a string buffer to print number */ 00026 lcd.setCursor(0, 0); /* set cursor at 0th column and 0st row */ 00027 lcd.print(strBuffer); /* print the string buffer */ 00028 00029 wait(0.5); 00030 } 00031 }
Generated on Tue Jul 12 2022 17:49:53 by
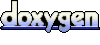