
working code of cdms with i2c
Fork of rtos_basic by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "master.h" 00004 00005 //#include "tmtc.h" 00006 //#include "science.h" 00007 //#include "hk.h" 00008 00009 #define intmax 11999 00010 00011 Thread* t_tmtc; 00012 Thread* t_science_store_sd; 00013 Thread* t_hk_store_sd; 00014 00015 void t_tmtc_main(void const *args); 00016 void t_science_main(void const *args); 00017 void t_hk_main(void const *args); 00018 void scheduler(void const * args); 00019 00020 Timer timer_main; 00021 int schedcount = 1; 00022 Mutex critical; 00023 00024 int main() { 00025 Thread thread_tmtc(t_tmtc_main, NULL, osPriorityIdle); 00026 t_tmtc = &thread_tmtc; 00027 Thread thread_science(t_science_main, NULL, osPriorityLow); 00028 t_science_store_sd = &thread_science; 00029 Thread thread_hk(t_hk_main, NULL, osPriorityBelowNormal); 00030 t_hk_store_sd = &thread_hk; 00031 00032 RtosTimer schedule(scheduler,osTimerPeriodic); 00033 timer_main.start(); 00034 schedule.start(5000); 00035 Thread::wait(osWaitForever); 00036 } 00037 00038 void scheduler(void const * args) 00039 { 00040 if(schedcount == intmax+1) //the value is reset at this value so as to ensure smooth flow, 65532 and 0 are divisible by 3 and 2. 00041 { 00042 schedcount =0; 00043 } 00044 00045 if(schedcount%4==0) 00046 { 00047 printf("\nHK signal at %f\n",timer_main.read()); 00048 t_hk_store_sd->signal_set(0x01); 00049 } 00050 00051 if(schedcount%3==0) 00052 { 00053 printf("\nScience signal at %f\n",timer_main.read()); 00054 t_science_store_sd->signal_set(0x01); 00055 } 00056 00057 if(schedcount==5) 00058 { 00059 printf("\nTMTC signalled"); 00060 t_tmtc->signal_set(0x1); 00061 } 00062 00063 schedcount++; 00064 } 00065 00066 void t_science_main(void const *args) { 00067 while (true) { 00068 Thread::signal_wait(0x1); 00069 critical.lock(); 00070 printf("science data aquisition started\n"); 00071 wait(3); 00072 printf("science data acquisition ended\n"); 00073 t_hk_store_sd->signal_set(0x10); 00074 critical.unlock(); 00075 wait(3); 00076 printf("Science data written to SD card\n"); 00077 } 00078 } 00079 00080 void t_hk_main(void const *args) { 00081 while (true) { 00082 Thread::signal_wait(0x1); 00083 critical.lock(); 00084 printf("HK acquisition started\n"); 00085 FUNC_I2C_MASTER_MAIN('1',0x20,24 ); 00086 wait(2); 00087 printf("HK acquisition ended\n"); 00088 critical.unlock(); 00089 wait(2); 00090 printf("HK data written to SD card"); 00091 } 00092 } 00093 00094 void t_tmtc_main(void const *args) { 00095 Thread::signal_wait(0x1); 00096 critical.lock(); 00097 printf("tmtc started\n"); 00098 wait(5); 00099 printf("tmtc ended\n"); 00100 critical.unlock(); 00101 wait(5); 00102 printf("tmtc executed\n"); 00103 }
Generated on Sun Jul 24 2022 21:13:36 by
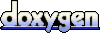