This is the final version of Mini Gateway for Automation and Security desgined for Renesas GR Peach Design Contest
Dependencies: GR-PEACH_video GraphicsFramework HTTPServer R_BSP mbed-rpc mbed-rtos Socket lwip-eth lwip-sys lwip FATFileSystem
Fork of mbed-os-example-mbed5-blinky by
FunctionPointer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_FUNCTIONPOINTER_H 00017 #define MBED_FUNCTIONPOINTER_H 00018 00019 #include "platform/Callback.h" 00020 #include "platform/toolchain.h" 00021 #include <string.h> 00022 #include <stdint.h> 00023 00024 namespace mbed { 00025 /** \addtogroup platform */ 00026 /** @{*/ 00027 00028 00029 // Declarations for backwards compatibility 00030 // To be foward compatible, code should adopt the Callback class 00031 template <typename R, typename A1> 00032 class FunctionPointerArg1 : public Callback<R(A1)> { 00033 public: 00034 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00035 "FunctionPointerArg1<R, A> has been replaced by Callback<R(A)>") 00036 FunctionPointerArg1(R (*function)(A1) = 0) 00037 : Callback<R(A1)>(function) {} 00038 00039 template<typename T> 00040 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00041 "FunctionPointerArg1<R, A> has been replaced by Callback<R(A)>") 00042 FunctionPointerArg1(T *object, R (T::*member)(A1)) 00043 : Callback<R(A1)>(object, member) {} 00044 00045 R (*get_function())(A1) { 00046 return *reinterpret_cast<R (**)(A1)>(this); 00047 } 00048 00049 R call(A1 a1) const { 00050 if (!Callback<R(A1)>::operator bool()) { 00051 return (R)0; 00052 } 00053 00054 return Callback<R(A1)>::call(a1); 00055 } 00056 00057 R operator()(A1 a1) const { 00058 return Callback<R(A1)>::call(a1); 00059 } 00060 }; 00061 00062 template <typename R> 00063 class FunctionPointerArg1<R, void> : public Callback<R()> { 00064 public: 00065 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00066 "FunctionPointer has been replaced by Callback<void()>") 00067 FunctionPointerArg1(R (*function)() = 0) 00068 : Callback<R()>(function) {} 00069 00070 template<typename T> 00071 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00072 "FunctionPointer has been replaced by Callback<void()>") 00073 FunctionPointerArg1(T *object, R (T::*member)()) 00074 : Callback<R()>(object, member) {} 00075 00076 R (*get_function())() { 00077 return *reinterpret_cast<R (**)()>(this); 00078 } 00079 00080 R call() const { 00081 if (!Callback<R()>::operator bool()) { 00082 return (R)0; 00083 } 00084 00085 return Callback<R()>::call(); 00086 } 00087 00088 R operator()() const { 00089 return Callback<R()>::call(); 00090 } 00091 }; 00092 00093 typedef FunctionPointerArg1<void, void> FunctionPointer; 00094 00095 00096 } // namespace mbed 00097 00098 #endif 00099 00100 /** @}*/
Generated on Tue Jul 12 2022 15:10:51 by
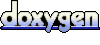