
Vincent Nys
Fork of PGO6_VoteController_template by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #define APP_VERSION 0.6f 00002 #define MQTT_VERSION 3 00003 #define BROKER_NAME "broker.hivemq.com" 00004 #define BROKER_PORT 1883 00005 00006 #include "debounce_button.h" 00007 #include "EthernetInterface.h" 00008 #include "MQTTNetwork.h" 00009 #include "MQTTmbed.h" 00010 #include "MQTTClient.h" 00011 00012 char* topic = "dansvloerVincent/votes"; 00013 volatile bool button1_pressed = false; // Used in the main loop 00014 volatile bool button1_enabled = true; // Used for debouncing 00015 volatile int multiclick_state = 0; // Counts how many clicks occured in the time slot, used in main loop 00016 volatile bool button1_busy = false; // Informs the mainloop that the user is clicking the button 00017 00018 /** 00019 TODO 00020 ---- 00021 - Check if the button has been pressed. If so, print the amount of clicks to a serial terminal. 00022 - Make an MQTT-service which: 00023 - starts up a network using EthernetInterface. Make sure the development board requests its address via DHCP. 00024 - makes a client and connects it to the broker using a client ID and credentials (username & password). 00025 - sends messages at the same topic as the smartphone app from PGO 2. Feel free to choose which Quality of Service 00026 you are going to use. Make a separate function which handles the sending procedure. Therefore, this function 00027 can be called each time we want to send a certain message. 00028 - When the button is pressed once, we send an upvote. When pressed twice, a downvote is sent. By pressing 4 times, 00029 the program disconnects from the broker and terminates. 00030 00031 Extra 00032 ----- 00033 - Subscribe to the topic on which the song data is published. Display this received message on the serial terminal. 00034 - Test this controller in the complete system of PGO 2. Use these controllers instead of the smartphones. 00035 00036 Tips & tricks 00037 ------------- 00038 - To generate an interrupt on the press of a button, use: 00039 InterruptIn button(USER_BUTTON); 00040 ... 00041 button.fall(callback(someFunction)); 00042 - Before implementing MQTT, test the multiclick feature first. 00043 - Have a look at the MQTT-library for Mbed and the HelloMQTT-example. 00044 - To have a uniform message sending procedure, use the following function usage: 00045 sendMessage(&client, topic, buf, qos, retained, duplicate) 00046 */ 00047 00048 int main(int argc, char* argv[]) 00049 { 00050 printf("Vote controller booting...\n"); 00051 int rc; 00052 00053 InterruptIn button(USER_BUTTON); 00054 button.fall(&button1_onpressed_cb); 00055 00056 EthernetInterface eth; 00057 eth.connect(); 00058 const char *ip = eth.get_ip_address(); 00059 printf("IP address is: %s\n", ip ? ip : "No IP"); 00060 00061 NetworkInterface *net = ð 00062 MQTTNetwork mqtt( net ); 00063 00064 MQTT::Client<MQTTNetwork, Countdown> client( mqtt ); 00065 00066 char *host = "143.129.39.151";//"broker.mqttdashboard.com"; 00067 int port = 1883; 00068 rc = mqtt.connect(host, port); 00069 if (rc != 0) 00070 printf("rc from TCP connect is %d\n", rc); 00071 00072 00073 00074 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00075 data.MQTTVersion = MQTT_VERSION; 00076 data.clientID.cstring = "VincentController"; 00077 data.username.cstring = "smartcity"; 00078 data.password.cstring = "smartcity"; 00079 if ((rc = client.connect(data)) != 0) 00080 printf("rc from MQTT connect is %d\n", rc); 00081 00082 MQTT::Message message; 00083 message.qos = MQTT::QOS1; 00084 printf("Start clicking\n"); 00085 char buf[100]; 00086 00087 while( multiclick_state < 4 ) 00088 { 00089 // Keep running 00090 00091 00092 if(!button1_busy && multiclick_state > 0) 00093 { 00094 //printf("%d\n", multiclick_state); 00095 if(multiclick_state == 1) 00096 sprintf(buf, "like\n"); 00097 else 00098 //buf = "dislike\n"; 00099 sprintf(buf, "dislike\n"); 00100 multiclick_state = 0; 00101 00102 printf("%s\n", buf); 00103 message.payload = (void *)buf; 00104 message.payloadlen = strlen(buf) + 1; 00105 client.publish(topic, message); 00106 } 00107 00108 00109 //printf("%d\n", multiclick_state); 00110 } 00111 00112 00113 00114 if ((rc = client.disconnect()) != 0) 00115 printf("rc from disconnect was %d\n", rc); 00116 mqtt.disconnect(); 00117 00118 printf("Terminate...\n"); 00119 00120 return 0; 00121 }
Generated on Mon Aug 15 2022 01:45:55 by
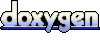