
Vincent Nys
Fork of PGO6_VoteController_template by
Embed:
(wiki syntax)
Show/hide line numbers
debounce_button.cpp
00001 #include "debounce_button.h" 00002 00003 DigitalOut led1(LED1); 00004 volatile int click_counter = 0; 00005 Timeout multiclick; 00006 Timeout debounce; 00007 00008 /** 00009 Some tips and tricks: 00010 - To use the built-in LED: 00011 DigitalOut led1(LED1); 00012 ... 00013 led1 = 1; 00014 - To delay the call of a function: 00015 Timeout someTimeout; 00016 ... 00017 someTimeout.attach(callback(&someFunction), 0.5) with 0.5 as 500 milliseconds 00018 - The variables that are used in interrupt callbacks have to be volatile, 00019 because these variables can change at any time. Therefore, the compiler is not 00020 going to make optimisations. 00021 */ 00022 00023 /** 00024 TODO 00025 ---- 00026 This function: 00027 - stores the amount of clicks in a variable which is read by the main loop. 00028 - resets the click counter which is used inside this file. 00029 - lowers a flag which tells the main loop that the user stopped pressing the button 00030 such that it can proceed its program. 00031 - turns the built-in LED off. Therefore, the user gets informed that the program stopped counting the clicks. 00032 */ 00033 void button1_multiclick_reset_cb(void) 00034 { 00035 button1_busy = false; 00036 multiclick_state = click_counter; 00037 click_counter = 0; 00038 led1 = 0; 00039 } 00040 00041 /** 00042 TODO 00043 ---- 00044 This function enables the button again, such that unwanted clicks of the bouncing button get ignored. 00045 */ 00046 void button1_enabled_cb(void) 00047 { 00048 button1_enabled = true; 00049 } 00050 00051 /** 00052 TODO 00053 ---- 00054 This function: 00055 - turns the built-in LED on, so the user gets informed that the program has started with counting clicks 00056 - disables the button such that the debouncer is active 00057 - enables the button again after a certain amount of time 00058 (use interrupts with "button1_enabled_cb()" as callback. 00059 - counts the amount of clicks within a period of 1 second 00060 - informs the main loop that the button has been pressed 00061 - informs the main loop that the user is clicking the button. 00062 Therefore, this main loop cannot continue its procedure until the clicks within 1 second have been counted. 00063 */ 00064 void button1_onpressed_cb(void) 00065 { 00066 if(!button1_busy) 00067 { 00068 multiclick.attach( &button1_multiclick_reset_cb, 1 ); 00069 } 00070 button1_busy = true; 00071 led1 = 1; 00072 if( button1_enabled ) 00073 { 00074 button1_enabled = false; 00075 click_counter++; 00076 debounce.attach( &button1_enabled_cb, 0.08 ); // 80ms 00077 } 00078 }
Generated on Mon Aug 15 2022 01:45:55 by
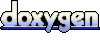