
USBHID test program
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "USBHID.h" 00003 00004 /* 00005 * HidTest.c 00006 * 00007 * Program to test the UsbHid with HidTest.java 00008 * Java files in USBHID bindings page 00009 * 00010 * This test program is allways sending a non blocking message and expecting to read a non blocking message 00011 * Messages are sent with the last byte cycling through '0'-'9' and LED4 toggles with each send 00012 * When a messsage is received: 00013 * LED2 toggles 00014 * Received message is sent, blocking. 00015 * A message is read blocking 00016 * The recived message is now the new non blocking message to be sent 00017 * 00018 * victorix 04.02.2013 00019 */ 00020 uint16_t vendor_id=0x1798; 00021 uint16_t product_id=0x2013; 00022 uint16_t product_release=0x0001; 00023 bool connect=true; 00024 #define HID_LEN 32 00025 00026 USBHID hid(HID_LEN, HID_LEN, vendor_id, product_id, product_release, connect); 00027 HID_REPORT hidOut; 00028 HID_REPORT hidIn; 00029 00030 DigitalOut l2(LED2); 00031 DigitalOut l4(LED4); 00032 00033 int main() { 00034 00035 char s[HID_LEN]="Have a nice day :)"; 00036 memcpy(hidOut.data,s,HID_LEN); 00037 hidOut.length=HID_LEN; 00038 00039 hidIn.length=HID_LEN; 00040 00041 while(1) { 00042 l4=!l4; //toggle Led4 00043 hid.sendNB(&hidOut); //send non blocking 00044 //last byte used as a simple frame counter 00045 if(hidOut.data[HID_LEN-1]>='0' && hidOut.data[HID_LEN-1]<'9') 00046 hidOut.data[HID_LEN-1]++; 00047 else hidOut.data[HID_LEN-1]='0'; 00048 wait(1); //wait 1s 00049 if(hid.readNB(&hidIn)){ //read non blocking 00050 l2=!l2; //toggle LED2 00051 memcpy(hidOut.data, hidIn.data, hidIn.length); //copy input to output 00052 hid.send(&hidOut); //send blocking 00053 wait(2); //wait 2s 00054 if(hid.read(&hidIn)){ //read blocking 00055 memcpy(hidOut.data, hidIn.data, hidIn.length); //copy input to output 00056 } 00057 } 00058 } 00059 }
Generated on Fri Jul 15 2022 01:00:56 by
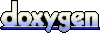