
BLE Observer example for nucleo platform
Dependencies: BLE_API X_NUCLEO_IDB0XA1 mbed
Dependents: BLE_NordicUART_IDB0XA1
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "BLE.h" 00019 00020 BLE ble; 00021 DigitalOut led1(LED1); 00022 00023 void periodicCallback(void) 00024 { 00025 led1 = !led1; /* Do blinky on LED1 while we're waiting for BLE events */ 00026 } 00027 00028 void advertisementCallback(const Gap::AdvertisementCallbackParams_t *params) { 00029 00030 printf("Adv peerAddr: [%02x %02x %02x %02x %02x %02x] rssi %d, ScanResp: %u, AdvType: %u\r\n", 00031 params->peerAddr[5], params->peerAddr[4], params->peerAddr[3], params->peerAddr[2], params->peerAddr[1], params->peerAddr[0], 00032 params->rssi, params->isScanResponse, params->type); 00033 #if DUMP_ADV_DATA 00034 for (unsigned index = 0; index < params->advertisingDataLen; index++) { 00035 printf("%02x ", params->advertisingData[index]); 00036 } 00037 printf("\r\n"); 00038 #endif /* DUMP_ADV_DATA */ 00039 } 00040 00041 int main(void) 00042 { 00043 led1 = 1; 00044 Ticker ticker; 00045 ticker.attach(periodicCallback, 1); 00046 00047 ble.init(); 00048 00049 ble.gap().setScanParams(500 /* scan interval */, 200 /* scan window */); 00050 ble.gap().startScan(advertisementCallback); 00051 00052 while (true) { 00053 ble.waitForEvent(); 00054 } 00055 }
Generated on Fri Jul 15 2022 02:35:06 by
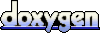