
I plan on using my mbed to capture some raw acceleration data from a rocket that I plan to launch soon. What I have build is a little program that records data from an accelerometer. When you flip the switch, the program automatically starts recording all x,y, and z values to a cvs file which can later be opened in excel to produce a graph. I have also added a mobile lcd screen to output the results.
main.cpp
00001 #include "mbed.h" 00002 #include "MobileLCD.h" 00003 00004 AnalogIn x1(p18); 00005 AnalogIn y1(p19); 00006 AnalogIn z1(p20); 00007 DigitalOut led(LED1); 00008 00009 DigitalIn start(p21); 00010 Serial pc(USBTX, USBRX); // tx, rx 00011 LocalFileSystem local("local"); // Create the local filesystem under the name "local" 00012 00013 MobileLCD lcd(p5, p6, p7, p8, p9); 00014 00015 int count=0; 00016 00017 int main() { 00018 lcd.background(0x0000FF); 00019 lcd.cls(); 00020 00021 00022 lcd.printf("Waiting for start..."); 00023 for (int i=0; i<130; i++) { 00024 lcd.pixel(i, 80 + sin((float)i / 5.0)*10, 0x000000); 00025 } 00026 while (1) { 00027 00028 pc.printf("%d",start.read()); 00029 if (start) { 00030 remove("/local/out.csv"); 00031 FILE *fp = fopen("/local/out.csv", "w"); // Open "out.txt" on the local file system for writing 00032 lcd.background(0x0000FF); 00033 lcd.cls(); 00034 while (start) { 00035 00036 pc.printf("XValue %.4f , YValue %.4f , ZValue %.4f\n",x1.read(),y1.read(),z1.read()); 00037 fprintf(fp," %f , %f , %f\n",x1.read(),y1.read(),z1.read()); 00038 00039 lcd.locate(0,3); 00040 lcd.printf("X:"); 00041 lcd.locate(0,6); 00042 lcd.printf("Y:"); 00043 lcd.locate(0,8); 00044 lcd.printf("Z:"); 00045 lcd.pixel(count++, -10 +x1.read()*100, 0x000000); 00046 lcd.pixel(count, 10+x1.read()*100, 0x000000); 00047 lcd.pixel(count,30+x1.read()*100, 0x000000); 00048 if (count == 120 ) { 00049 count = 0; 00050 lcd.cls(); 00051 } 00052 00053 } 00054 fclose(fp); 00055 } 00056 00057 00058 00059 00060 } 00061 }
Generated on Thu Jul 28 2022 05:07:29 by
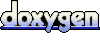