Updated
Fork of BLE_API by
Embed:
(wiki syntax)
Show/hide line numbers
GattClient.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GATT_CLIENT_H__ 00018 #define __GATT_CLIENT_H__ 00019 00020 #include "Gap.h" 00021 #include "GattAttribute.h" 00022 #include "ServiceDiscovery.h" 00023 00024 #include "GattCallbackParamTypes.h" 00025 00026 #include "CallChainOfFunctionPointersWithContext.h" 00027 00028 class GattClient { 00029 public: 00030 typedef FunctionPointerWithContext<const GattReadCallbackParams*> ReadCallback_t; 00031 typedef CallChainOfFunctionPointersWithContext<const GattReadCallbackParams*> ReadCallbackChain_t; 00032 00033 enum WriteOp_t { 00034 GATT_OP_WRITE_REQ = 0x01, /**< Write request. */ 00035 GATT_OP_WRITE_CMD = 0x02, /**< Write command. */ 00036 }; 00037 00038 typedef FunctionPointerWithContext<const GattWriteCallbackParams*> WriteCallback_t; 00039 typedef CallChainOfFunctionPointersWithContext<const GattWriteCallbackParams*> WriteCallbackChain_t; 00040 00041 typedef FunctionPointerWithContext<const GattHVXCallbackParams*> HVXCallback_t; 00042 typedef CallChainOfFunctionPointersWithContext<const GattHVXCallbackParams*> HVXCallbackChain_t; 00043 00044 /* 00045 * The following functions are meant to be overridden in the platform-specific sub-class. 00046 */ 00047 public: 00048 /** 00049 * Launch service discovery. Once launched, application callbacks will be 00050 * invoked for matching services or characteristics. isServiceDiscoveryActive() 00051 * can be used to determine status, and a termination callback (if one was set up) 00052 * will be invoked at the end. Service discovery can be terminated prematurely, 00053 * if needed, using terminateServiceDiscovery(). 00054 * 00055 * @param connectionHandle 00056 * Handle for the connection with the peer. 00057 * @param sc 00058 * This is the application callback for a matching service. Taken as 00059 * NULL by default. Note: service discovery may still be active 00060 * when this callback is issued; calling asynchronous BLE-stack 00061 * APIs from within this application callback might cause the 00062 * stack to abort service discovery. If this becomes an issue, it 00063 * may be better to make a local copy of the discoveredService and 00064 * wait for service discovery to terminate before operating on the 00065 * service. 00066 * @param cc 00067 * This is the application callback for a matching characteristic. 00068 * Taken as NULL by default. Note: service discovery may still be 00069 * active when this callback is issued; calling asynchronous 00070 * BLE-stack APIs from within this application callback might cause 00071 * the stack to abort service discovery. If this becomes an issue, 00072 * it may be better to make a local copy of the discoveredCharacteristic 00073 * and wait for service discovery to terminate before operating on the 00074 * characteristic. 00075 * @param matchingServiceUUID 00076 * UUID-based filter for specifying a service in which the application is 00077 * interested. By default it is set as the wildcard UUID_UNKNOWN, 00078 * in which case it matches all services. If characteristic-UUID 00079 * filter (below) is set to the wildcard value, then a service 00080 * callback will be invoked for the matching service (or for every 00081 * service if the service filter is a wildcard). 00082 * @param matchingCharacteristicUUIDIn 00083 * UUID-based filter for specifying characteristic in which the application 00084 * is interested. By default it is set as the wildcard UUID_UKNOWN 00085 * to match against any characteristic. If both service-UUID 00086 * filter and characteristic-UUID filter are used with non-wildcard 00087 * values, then only a single characteristic callback is 00088 * invoked for the matching characteristic. 00089 * 00090 * @note Using wildcard values for both service-UUID and characteristic- 00091 * UUID will result in complete service discovery: callbacks being 00092 * called for every service and characteristic. 00093 * 00094 * @note Providing NULL for the characteristic callback will result in 00095 * characteristic discovery being skipped for each matching 00096 * service. This allows for an inexpensive method to discover only 00097 * services. 00098 * 00099 * @return 00100 * BLE_ERROR_NONE if service discovery is launched successfully; else an appropriate error. 00101 */ 00102 virtual ble_error_t launchServiceDiscovery(Gap::Handle_t connectionHandle, 00103 ServiceDiscovery::ServiceCallback_t sc = NULL, 00104 ServiceDiscovery::CharacteristicCallback_t cc = NULL, 00105 const UUID &matchingServiceUUID = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN), 00106 const UUID &matchingCharacteristicUUIDIn = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN)) { 00107 /* Avoid compiler warnings about unused variables. */ 00108 (void)connectionHandle; 00109 (void)sc; 00110 (void)cc; 00111 (void)matchingServiceUUID; 00112 (void)matchingCharacteristicUUIDIn; 00113 00114 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00115 } 00116 00117 /** 00118 * Launch service discovery for services. Once launched, service discovery will remain 00119 * active with service-callbacks being issued back into the application for matching 00120 * services. isServiceDiscoveryActive() can be used to 00121 * determine status, and a termination callback (if set up) will be invoked 00122 * at the end. Service discovery can be terminated prematurely, if needed, 00123 * using terminateServiceDiscovery(). 00124 * 00125 * @param connectionHandle 00126 * Handle for the connection with the peer. 00127 * @param sc 00128 * This is the application callback for a matching service. Note: service discovery may still be active 00129 * when this callback is issued; calling asynchronous BLE-stack 00130 * APIs from within this application callback might cause the 00131 * stack to abort service discovery. If this becomes an issue, it 00132 * may be better to make a local copy of the discoveredService and 00133 * wait for service discovery to terminate before operating on the 00134 * service. 00135 * @param matchingServiceUUID 00136 * UUID-based filter for specifying a service in which the application is 00137 * interested. By default it is set as the wildcard UUID_UNKNOWN, 00138 * in which case it matches all services. 00139 * 00140 * @return 00141 * BLE_ERROR_NONE if service discovery is launched successfully; else an appropriate error. 00142 */ 00143 virtual ble_error_t discoverServices(Gap::Handle_t connectionHandle, 00144 ServiceDiscovery::ServiceCallback_t callback, 00145 const UUID &matchingServiceUUID = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN)) { 00146 return launchServiceDiscovery(connectionHandle, callback, NULL, matchingServiceUUID); /* We take advantage of the property 00147 * that providing NULL for the characteristic callback will result in 00148 * characteristic discovery being skipped for each matching 00149 * service. This allows for an inexpensive method to discover only 00150 * services. Porters are free to override this. */ 00151 } 00152 00153 /** 00154 * Launch service discovery for services. Once launched, service discovery will remain 00155 * active with service-callbacks being issued back into the application for matching 00156 * services. isServiceDiscoveryActive() can be used to 00157 * determine status, and a termination callback (if set up) will be invoked 00158 * at the end. Service discovery can be terminated prematurely, if needed, 00159 * using terminateServiceDiscovery(). 00160 * 00161 * @param connectionHandle 00162 * Handle for the connection with the peer. 00163 * @param sc 00164 * This is the application callback for a matching service. Note: service discovery may still be active 00165 * when this callback is issued; calling asynchronous BLE-stack 00166 * APIs from within this application callback might cause the 00167 * stack to abort service discovery. If this becomes an issue, it 00168 * may be better to make a local copy of the discoveredService and 00169 * wait for service discovery to terminate before operating on the 00170 * service. 00171 * @param startHandle, endHandle 00172 * Handle range within which to limit the search. 00173 * 00174 * @return 00175 * BLE_ERROR_NONE if service discovery is launched successfully; else an appropriate error. 00176 */ 00177 virtual ble_error_t discoverServices(Gap::Handle_t connectionHandle, 00178 ServiceDiscovery::ServiceCallback_t callback, 00179 GattAttribute::Handle_t startHandle, 00180 GattAttribute::Handle_t endHandle) { 00181 /* Avoid compiler warnings about unused variables. */ 00182 (void)connectionHandle; 00183 (void)callback; 00184 (void)startHandle; 00185 (void)endHandle; 00186 00187 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00188 } 00189 00190 /** 00191 * Is service-discovery currently active? 00192 */ 00193 virtual bool isServiceDiscoveryActive(void) const { 00194 return false; /* Requesting action from porters: override this API if this capability is supported. */ 00195 } 00196 00197 /** 00198 * Terminate an ongoing service discovery. This should result in an 00199 * invocation of TerminationCallback if service-discovery is active. 00200 */ 00201 virtual void terminateServiceDiscovery(void) { 00202 /* Requesting action from porters: override this API if this capability is supported. */ 00203 } 00204 00205 /* Initiate a GATT Client read procedure by attribute-handle. */ 00206 virtual ble_error_t read(Gap::Handle_t connHandle, GattAttribute::Handle_t attributeHandle, uint16_t offset) const { 00207 /* Avoid compiler warnings about unused variables. */ 00208 (void)connHandle; 00209 (void)attributeHandle; 00210 (void)offset; 00211 00212 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00213 } 00214 00215 /** 00216 * Initiate a GATT Client write procedure. 00217 * 00218 * @param[in] cmd 00219 * Command can be either a write-request (which generates a 00220 * matching response from the peripheral), or a write-command 00221 * (which doesn't require the connected peer to respond). 00222 * @param[in] connHandle 00223 * Connection handle. 00224 * @param[in] attributeHandle 00225 * Handle for the target attribtue on the remote GATT server. 00226 * @param[in] length 00227 * Length of the new value. 00228 * @param[in] value 00229 * New value being written. 00230 */ 00231 virtual ble_error_t write(GattClient::WriteOp_t cmd, 00232 Gap::Handle_t connHandle, 00233 GattAttribute::Handle_t attributeHandle, 00234 size_t length, 00235 const uint8_t *value) const { 00236 /* Avoid compiler warnings about unused variables. */ 00237 (void)cmd; 00238 (void)connHandle; 00239 (void)attributeHandle; 00240 (void)length; 00241 (void)value; 00242 00243 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porters: override this API if this capability is supported. */ 00244 } 00245 00246 /* Event callback handlers. */ 00247 public: 00248 /** 00249 * Set up a callback for read response events. 00250 * It is possible to remove registered callbacks using 00251 * onDataRead().detach(callbackToRemove) 00252 */ 00253 void onDataRead(ReadCallback_t callback) { 00254 onDataReadCallbackChain.add(callback); 00255 } 00256 00257 /** 00258 * @brief provide access to the callchain of read callbacks 00259 * It is possible to register callbacks using onDataRead().add(callback); 00260 * It is possible to unregister callbacks using onDataRead().detach(callback) 00261 * @return The read callbacks chain 00262 */ 00263 ReadCallbackChain_t& onDataRead() { 00264 return onDataReadCallbackChain; 00265 } 00266 00267 /** 00268 * Set up a callback for write response events. 00269 * It is possible to remove registered callbacks using 00270 * onDataWritten().detach(callbackToRemove). 00271 * @Note: Write commands (issued using writeWoResponse) don't generate a response. 00272 */ 00273 void onDataWritten(WriteCallback_t callback) { 00274 onDataWriteCallbackChain.add(callback); 00275 } 00276 00277 /** 00278 * @brief provide access to the callchain of data written callbacks 00279 * It is possible to register callbacks using onDataWritten().add(callback); 00280 * It is possible to unregister callbacks using onDataWritten().detach(callback) 00281 * @return The data written callbacks chain 00282 */ 00283 WriteCallbackChain_t& onDataWritten() { 00284 return onDataWriteCallbackChain; 00285 } 00286 00287 /** 00288 * Set up a callback for write response events. 00289 * @Note: Write commands (issued using writeWoResponse) don't generate a response. 00290 * 00291 * @note: This API is now *deprecated* and will be dropped in the future. 00292 * Please use onDataWritten() instead. 00293 */ 00294 void onDataWrite(WriteCallback_t callback) { 00295 onDataWritten(callback); 00296 } 00297 00298 /** 00299 * Set up a callback for when serviceDiscovery terminates. 00300 */ 00301 virtual void onServiceDiscoveryTermination(ServiceDiscovery::TerminationCallback_t callback) { 00302 (void)callback; /* Avoid compiler warnings about ununsed variables. */ 00303 00304 /* Requesting action from porters: override this API if this capability is supported. */ 00305 } 00306 00307 /** 00308 * Set up a callback for when the GATT client receives an update event 00309 * corresponding to a change in the value of a characteristic on the remote 00310 * GATT server. 00311 * It is possible to remove registered callbacks using onHVX().detach(callbackToRemove). 00312 */ 00313 void onHVX(HVXCallback_t callback) { 00314 onHVXCallbackChain.add(callback); 00315 } 00316 00317 00318 /** 00319 * @brief provide access to the callchain of HVX callbacks 00320 * It is possible to register callbacks using onHVX().add(callback); 00321 * It is possible to unregister callbacks using onHVX().detach(callback) 00322 * @return The HVX callbacks chain 00323 */ 00324 HVXCallbackChain_t& onHVX() { 00325 return onHVXCallbackChain; 00326 } 00327 00328 protected: 00329 GattClient() { 00330 /* Empty */ 00331 } 00332 00333 /* Entry points for the underlying stack to report events back to the user. */ 00334 public: 00335 void processReadResponse(const GattReadCallbackParams *params) { 00336 onDataReadCallbackChain(params); 00337 } 00338 00339 void processWriteResponse(const GattWriteCallbackParams *params) { 00340 onDataWriteCallbackChain(params); 00341 } 00342 00343 void processHVXEvent(const GattHVXCallbackParams *params) { 00344 if (onHVXCallbackChain) { 00345 onHVXCallbackChain(params); 00346 } 00347 } 00348 00349 protected: 00350 ReadCallbackChain_t onDataReadCallbackChain; 00351 WriteCallbackChain_t onDataWriteCallbackChain; 00352 HVXCallbackChain_t onHVXCallbackChain; 00353 00354 private: 00355 /* Disallow copy and assignment. */ 00356 GattClient(const GattClient &); 00357 GattClient& operator=(const GattClient &); 00358 }; 00359 00360 #endif // ifndef __GATT_CLIENT_H__
Generated on Sun Jul 17 2022 08:42:21 by
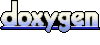