W5200(WIZ820io) network interface
Embed:
(wiki syntax)
Show/hide line numbers
WIZ820ioNetIf.cpp
00001 // WIZ820ioNetIf.cpp 2012/4/17 00002 #include "WIZ820ioNetIf.h" 00003 #include "w5100.h" 00004 00005 extern SPI* pSPI; // w5100.cpp 00006 extern DigitalOut* pCS; // w5100.cpp 00007 00008 DigitalOut* pRESET = NULL; 00009 00010 void hardware_reset() { 00011 if (!pRESET) { 00012 pRESET = new DigitalOut(p15); 00013 } 00014 if (pRESET) { 00015 pRESET->write(1); 00016 pRESET->write(0); 00017 wait_us(2); 00018 pRESET->write(1); 00019 wait_ms(150); 00020 } 00021 } 00022 00023 bool wait_linkup(int timeout = 5000) { 00024 Timer link_t; 00025 link_t.start(); 00026 while(link_t.read_ms() < timeout) { 00027 if (0x20 & W5100.readPHYSTATUS()) { 00028 return true; 00029 } 00030 wait_ms(50); 00031 } 00032 return false; 00033 } 00034 00035 void WIZ820ioNetIf::spi(PinName mosi,PinName miso, PinName sclk) { 00036 pSPI = new SPI(mosi,miso,sclk); 00037 } 00038 void WIZ820ioNetIf::cs(PinName _cs) { 00039 pCS = new DigitalOut(_cs); 00040 } 00041 void WIZ820ioNetIf::reset(PinName _reset) { 00042 pRESET = new DigitalOut(_reset); 00043 } 00044 00045 int WIZ820ioNetIf::setup(int timeout_ms) { 00046 hardware_reset(); 00047 W5100.init(); 00048 wait_linkup(); 00049 return W5200NetIf::setup(timeout_ms); 00050 }
Generated on Tue Jul 12 2022 19:58:52 by
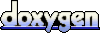