W5200(WIZ820io) network interface
Embed:
(wiki syntax)
Show/hide line numbers
DHCPClient.h
00001 // DHCPClient.h 2012/4/13 00002 #ifndef DHCPCLIENT_H 00003 #define DHCPCLIENT_H 00004 #include "UDPSocket.h" 00005 00006 00007 #define DHCP_OFFSET_OP 0 00008 #define DHCP_OFFSET_XID 4 00009 #define DHCP_OFFSET_YIADDR 16 00010 #define DHCP_OFFSET_CHADDR 28 00011 #define DHCP_OFFSET_MAGIC_COOKIE 236 00012 #define DHCP_OFFSET_OPTIONS 240 00013 #define DHCP_MAX_PACKET_SIZE 600 00014 00015 class DHCPClient { 00016 int discover(uint8_t buf[], int size); 00017 int request(uint8_t buf[], int size); 00018 int offer_ack(uint8_t buf[], int size); 00019 bool verify(uint8_t buf[], int len); 00020 public: 00021 void callback(UDPSocketEvent e); 00022 int setup(int timeout_ms = 15000); 00023 DHCPClient(); 00024 uint8_t chaddr[6]; // MAC 00025 uint8_t yiaddr[4]; // IP 00026 uint8_t dnsaddr[4]; // DNS 00027 uint8_t gateway[4]; 00028 uint8_t netmask[4]; 00029 private: 00030 UDPSocket* m_udp; 00031 uint8_t xid[4]; 00032 bool exit_flag; 00033 }; 00034 #endif //DHCPCLIENT_H
Generated on Tue Jul 12 2022 19:58:52 by
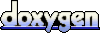