
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
mem.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __MEM_H__ 00010 #define __MEM_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief VM Memory 00016 * 00017 * VM memory header. 00018 */ 00019 00020 00021 /** 00022 * Memory Space enum. 00023 * 00024 * Defines the different addressable areas of the system. 00025 */ 00026 typedef enum PmMemSpace_e 00027 { 00028 MEMSPACE_RAM = 0, 00029 MEMSPACE_PROG, 00030 MEMSPACE_EEPROM, 00031 MEMSPACE_SEEPROM, 00032 MEMSPACE_OTHER0, 00033 MEMSPACE_OTHER1, 00034 MEMSPACE_OTHER2, 00035 MEMSPACE_OTHER3 00036 } PmMemSpace_t, *pPmMemSpace_t; 00037 00038 00039 /** 00040 * Returns the byte at the given address in memspace. 00041 * 00042 * Increments the address (just like getc and read(1)) 00043 * to make image loading work (recursive). 00044 * 00045 * @param memspace memory space/type 00046 * @param paddr ptr to address 00047 * @return byte from memory. 00048 * paddr - points to the next byte 00049 */ 00050 #define mem_getByte(memspace, paddr) plat_memGetByte((memspace), (paddr)) 00051 00052 /** 00053 * Returns the 2-byte word at the given address in memspace. 00054 * 00055 * Word obtained in LITTLE ENDIAN order (per Python convention). 00056 * afterward, addr points one byte past the word. 00057 * 00058 * @param memspace memory space 00059 * @param paddr ptr to address 00060 * @return word from memory. 00061 * addr - points one byte past the word 00062 */ 00063 uint16_t mem_getWord(PmMemSpace_t memspace, uint8_t const **paddr); 00064 00065 /** 00066 * Returns the 4-byte int at the given address in memspace. 00067 * 00068 * Int obtained in LITTLE ENDIAN order (per Python convention). 00069 * afterward, addr points one byte past the int. 00070 * 00071 * @param memspace memory space 00072 * @param paddr ptr to address 00073 * @return int from memory. 00074 * addr - points one byte past the word 00075 */ 00076 uint32_t mem_getInt(PmMemSpace_t memspace, uint8_t const **paddr); 00077 00078 #ifdef HAVE_FLOAT 00079 /** 00080 * Returns the 4-byte float at the given address in memspace. 00081 * 00082 * Float obtained in LITTLE ENDIAN order (per Python convention). 00083 * afterward, addr points one byte past the float. 00084 * 00085 * @param memspace memory space 00086 * @param paddr ptr to address 00087 * @return float from memory. 00088 * addr - points one byte past the word 00089 */ 00090 float mem_getFloat(PmMemSpace_t memspace, uint8_t const **paddr); 00091 #endif /* HAVE_FLOAT */ 00092 00093 /** 00094 * Copies count number of bytes from src in memspace to dest in RAM. 00095 * Leaves dest and src pointing one byte past end of the data. 00096 * 00097 * @param memspace memory space/type of source 00098 * @param pdest ptr to destination address 00099 * @param psrc ptr to source address 00100 * @param count number of bytes to copy 00101 * @return nothing. 00102 * src, dest - point 1 past end of data 00103 * @see sli_memcpy 00104 */ 00105 void mem_copy(PmMemSpace_t memspace, 00106 uint8_t **pdest, uint8_t const **psrc, uint16_t count); 00107 00108 /** 00109 * Returns the number of bytes in the C string pointed to by pstr. 00110 * Does not modify pstr 00111 * 00112 * @param memspace memory space/type of source 00113 * @param pstr ptr to source C string 00114 * @return Number of bytes in the string. 00115 */ 00116 uint16_t mem_getStringLength(PmMemSpace_t memspace, 00117 uint8_t const *const pstr); 00118 00119 /** 00120 * Compares a byte array in RAM to a byte array in the given memory space 00121 * 00122 * @param cname Pointer to byte array in RAM 00123 * @param cnamelen Length of byte array to compare 00124 * @param memspace Memory space of other byte array 00125 * @param paddr Pointer to address of other byte array 00126 * @return PM_RET_OK if all bytes in both arrays match; PM_RET_NO otherwise 00127 */ 00128 PmReturn_t mem_cmpn(uint8_t *cname, uint16_t cnamelen, PmMemSpace_t memspace, 00129 uint8_t const **paddr); 00130 00131 #endif /* __MEM_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
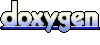