
Webcam Server.
Dependencies: uvchost FatFileSystem mbed HTTPServer NetServicesMin
WebcamInput.cpp
00001 #include "WebcamServerConfig.h" 00002 #include "WebcamInput.h" 00003 #include "WebcamHandler.h" 00004 #include "myjpeg.h" 00005 //#define __DEBUG 00006 #include "mydbg.h" 00007 00008 DigitalOut led1(LED1), led2(LED2), led3(LED3), led4(LED4); 00009 00010 00011 WebcamInput::WebcamInput(int cam) 00012 :m_seq(0),m_cam(cam) 00013 { 00014 DBG("%p cam=%d\n", this, cam); 00015 m_t.reset(); 00016 m_t.start(); 00017 m_size = IMAGE_SIZE; 00018 m_image_buf = new uint8_t[m_size]; 00019 DBG_ASSERT(m_image_buf); 00020 } 00021 00022 void WebcamInput::input(uint16_t frame, uint8_t* buf, int len) 00023 { 00024 if (len <= 12) { 00025 return; 00026 } 00027 uint8_t* data = buf+12; 00028 int data_len = len - 12; 00029 00030 switch(m_seq) { 00031 case 0: 00032 if (m_t.read_ms() > INTERVAL_MS) { 00033 m_seq++; 00034 } 00035 break; 00036 case 1: 00037 if (!WebcamHandler::busy()) { 00038 m_seq++; 00039 } 00040 break; 00041 case 2: 00042 if ((buf[1]^m_bfh)&0x01) { // FID change 00043 m_pos = 0; 00044 if (m_cam == 0) { 00045 led3 = 1; 00046 } else { 00047 led4 = 1; 00048 } 00049 m_seq++; 00050 } else { 00051 break; 00052 } 00053 case 3: 00054 for(int i = 0; m_pos < IMAGE_SIZE && i < data_len; i++) { 00055 m_image_buf[m_pos++] = data[i]; 00056 } 00057 if (buf[1]&0x02) { // EOF 00058 #ifdef UVC_INSERT_DHT 00059 myjpeg JPEG(m_image_buf, m_pos, m_size); 00060 JPEG.analytics(); 00061 if (JPEG.DHT_pos == 0) { 00062 m_pos = JPEG.insertDHT(); 00063 } 00064 #endif // UVC_INSERT_DHT 00065 WebcamHandler::setImage(m_image_buf, m_pos, m_cam); 00066 m_t.reset(); 00067 m_seq = 0; 00068 if (m_cam == 0) { 00069 led3 = 0; 00070 } else { 00071 led4 = 0; 00072 } 00073 } 00074 break; 00075 } 00076 m_bfh = buf[1]; 00077 if (m_cam == 0) { 00078 led1 = buf[1]&1; // FID 00079 } else { 00080 led2 = buf[1]&1; // FID 00081 } 00082 //led4 = WebcamHandler::busy(); 00083 }
Generated on Sat Jul 23 2022 11:10:59 by
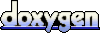