
USB composite device example program, drag-and-drop flash writer.
Dependencies: SWD USBDevice mbed BaseDAP
USB_HID.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #pragma once 00020 00021 /* These headers are included for child class. */ 00022 #include "USBEndpoints.h" 00023 #include "USBDescriptor.h" 00024 #include "USBDevice_Types.h" 00025 00026 #include "USBHID_Types.h" 00027 #include "USBDevice.h" 00028 00029 #define HID_EPINT_IN EP4IN 00030 #define HID_EPINT_OUT EP4OUT 00031 00032 /** USB HID device for CMSIS-DAP 00033 */ 00034 class USB_HID { 00035 public: 00036 00037 /** 00038 * Constructor 00039 * 00040 * @param output_report_length Maximum length of a sent report (up to 64 bytes) (default: 64 bytes) 00041 * @param input_report_length Maximum length of a received report (up to 64 bytes) (default: 64 bytes) 00042 */ 00043 USB_HID(USBDevice* device, uint8_t output_report_length = 64, uint8_t input_report_length = 64); 00044 00045 00046 /** 00047 * Send a Report. warning: blocking 00048 * 00049 * @param report Report which will be sent (a report is defined by all data and the length) 00050 * @returns true if successful 00051 */ 00052 bool send(HID_REPORT *report); 00053 00054 00055 /** 00056 * Send a Report. warning: non blocking 00057 * 00058 * @param report Report which will be sent (a report is defined by all data and the length) 00059 * @returns true if successful 00060 */ 00061 bool sendNB(HID_REPORT *report); 00062 00063 /** 00064 * Read a report: blocking 00065 * 00066 * @param report pointer to the report to fill 00067 * @returns true if successful 00068 */ 00069 bool read(HID_REPORT * report); 00070 00071 /** 00072 * Read a report: non blocking 00073 * 00074 * @param report pointer to the report to fill 00075 * @returns true if successful 00076 */ 00077 bool readNB(HID_REPORT * report); 00078 00079 /* 00080 * Get the length of the report descriptor 00081 * 00082 * @returns the length of the report descriptor 00083 */ 00084 virtual uint16_t reportDescLength(); 00085 00086 bool Request_callback(CONTROL_TRANSFER* transfer, uint8_t* hidDescriptor); 00087 protected: 00088 /* 00089 * Get the Report descriptor 00090 * 00091 * @returns pointer to the report descriptor 00092 */ 00093 virtual uint8_t * reportDesc(); 00094 00095 uint16_t reportLength; 00096 00097 private: 00098 USBDevice* _device; 00099 HID_REPORT outputReport; 00100 uint8_t output_length; 00101 uint8_t input_length; 00102 };
Generated on Wed Jul 13 2022 08:08:25 by
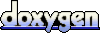