
USB composite device example program, drag-and-drop flash writer.
Dependencies: SWD USBDevice mbed BaseDAP
USB_CDC.cpp
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "stdint.h" 00020 #include "USB_CDC.h" 00021 #define MY_DEBUG 00022 #include "mydebug.h" 00023 00024 #define CDC_SET_LINE_CODING 0x20 00025 #define CDC_GET_LINE_CODING 0x21 00026 #define CDC_SET_CONTROL_LINE_STATE 0x22 00027 #define CDC_SEND_BREAK 0x23 00028 00029 #define MAX_CDC_REPORT_SIZE MAX_PACKET_SIZE_EPBULK 00030 00031 USB_CDC::USB_CDC(USBDevice* device) : _device(device), _rx_buf(128) 00032 { 00033 terminal_connected = false; 00034 //USBDevice::connect(); 00035 } 00036 00037 void USB_CDC::putc(int c) 00038 { 00039 if (terminal_connected) { 00040 uint8_t buf[1]; 00041 buf[0] = c; 00042 _device->write(CDC_EPBULK_IN, buf, sizeof(buf), MAX_CDC_REPORT_SIZE); 00043 } 00044 } 00045 00046 int USB_CDC::getc() 00047 { 00048 uint8_t c = 0; 00049 while (_rx_buf.isEmpty()); 00050 _rx_buf.dequeue(&c); 00051 return c; 00052 } 00053 00054 int USB_CDC::readable() 00055 { 00056 return _rx_buf.available() > 0 ? 1 : 0; 00057 } 00058 00059 int USB_CDC::writeable() 00060 { 00061 return 1; 00062 } 00063 00064 void USB_CDC::baud_callback(int baudrate) 00065 { 00066 DBG("baudrate=%d", baudrate); 00067 } 00068 00069 void USB_CDC::send_break_callback(uint16_t duration) 00070 { 00071 DBG("duration=%04x", duration); 00072 } 00073 00074 void USB_CDC::control_line_callback(int rts, int dtr) 00075 { 00076 DBG("rts=%d, dtr=%d", rts, dtr); 00077 } 00078 00079 bool USB_CDC::send(uint8_t * buffer, uint32_t size) { 00080 return _device->write(CDC_EPBULK_IN, buffer, size, MAX_CDC_REPORT_SIZE); 00081 } 00082 00083 bool USB_CDC::readEP(uint8_t * buffer, uint32_t * size) { 00084 if (!_device->readEP(CDC_EPBULK_OUT, buffer, size, MAX_CDC_REPORT_SIZE)) 00085 return false; 00086 if (!_device->readStart(CDC_EPBULK_OUT, MAX_CDC_REPORT_SIZE)) 00087 return false; 00088 return true; 00089 } 00090 00091 bool USB_CDC::readEP_NB(uint8_t * buffer, uint32_t * size) { 00092 if (!_device->readEP_NB(CDC_EPBULK_OUT, buffer, size, MAX_CDC_REPORT_SIZE)) 00093 return false; 00094 if (!_device->readStart(CDC_EPBULK_OUT, MAX_CDC_REPORT_SIZE)) 00095 return false; 00096 return true; 00097 } 00098 00099 bool USB_CDC::Request_callback(CONTROL_TRANSFER* transfer) 00100 { 00101 static uint8_t cdc_line_coding[7]= {0x80, 0x25, 0x00, 0x00, 0x00, 0x00, 0x08}; 00102 00103 if (transfer->setup.bmRequestType.Type == CLASS_TYPE) { 00104 switch (transfer->setup.bRequest) { 00105 case CDC_SET_LINE_CODING: // 0x20 00106 transfer->remaining = 7; 00107 transfer->notify = true; 00108 terminal_connected = true; 00109 return true; 00110 00111 case CDC_GET_LINE_CODING: // x021 00112 transfer->remaining = 7; 00113 transfer->ptr = cdc_line_coding; 00114 transfer->direction = DEVICE_TO_HOST; 00115 return true; 00116 00117 case CDC_SET_CONTROL_LINE_STATE: // 0x22 00118 control_line_callback((transfer->setup.wValue>>1) & 1, (transfer->setup.wValue) & 1); 00119 terminal_connected = false; 00120 return true; 00121 00122 case CDC_SEND_BREAK: // 0x23 00123 send_break_callback(transfer->setup.wValue); 00124 return true; 00125 } 00126 } 00127 return false; 00128 } 00129 00130 static uint32_t LD32(uint8_t* buf) 00131 { 00132 return buf[0]|buf[1]<<8|buf[2]<<16|buf[3]<<24; 00133 } 00134 00135 bool USB_CDC::RequestCompleted_callback(CONTROL_TRANSFER* transfer, uint8_t* buf, int length) 00136 { 00137 int baudrate; 00138 if (transfer->setup.bmRequestType.Type == CLASS_TYPE) { 00139 switch (transfer->setup.bRequest) { 00140 case CDC_SET_LINE_CODING: // 0x20 00141 baudrate = LD32(buf); 00142 baud_callback(baudrate); 00143 return true; 00144 } 00145 } 00146 DBG_HEX((uint8_t*)transfer, sizeof(CONTROL_TRANSFER)); 00147 return false; 00148 } 00149 00150 bool USB_CDC::EPBULK_OUT_callback() // virtual COM to target 00151 { 00152 uint8_t buf[MAX_CDC_REPORT_SIZE]; 00153 uint32_t size = 0; 00154 //we read the packet received and put it on the circular buffer 00155 _device->readEP(CDC_EPBULK_OUT, buf, &size, MAX_CDC_REPORT_SIZE); 00156 for(int i = 0; i < size; i++) { 00157 _rx_buf.queue(buf[i]); 00158 } 00159 00160 // We reactivate the endpoint to receive next characters 00161 _device->readStart(CDC_EPBULK_OUT, MAX_PACKET_SIZE_EPBULK); 00162 return true; 00163 }
Generated on Wed Jul 13 2022 08:08:25 by
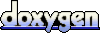